Everything you need to know about PostgreSQL triggers: A Comprehensive Guide
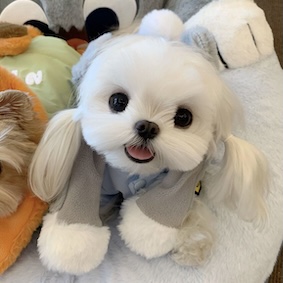
Implementing triggers in PostgreSQL is a powerful method to automate tasks, maintain data integrity, and enhance the functionality of your databases. This article delves into the fundamental concepts of PostgreSQL triggers, their types, how to create them, and best practices for effective usage. We will also explore how to integrate triggers with JSON data, optimizing their performance, and testing/debugging techniques. Additionally, we will highlight the advantages of using Chat2DB, an AI-driven database management tool that simplifies PostgreSQL operations.
Understanding PostgreSQL Triggers
In PostgreSQL, triggers are special procedures that are automatically executed in response to certain events on a particular table or view. They can be defined to run BEFORE or AFTER an event occurs, or even INSTEAD OF certain operations. The primary functions of triggers include enforcing data integrity, logging changes, and automating processes within the database.
Types of PostgreSQL Triggers
Trigger Type | Description |
---|---|
BEFORE Triggers | Executed before the event on a row or a statement, used for validation or modification of data. |
AFTER Triggers | Run after the event has occurred, suitable for logging or executing additional actions. |
INSTEAD OF Triggers | Typically used with views, allowing you to define actions that occur instead of the standard operations. |
Triggers can be defined at the row level or statement level, depending on whether you want to execute the trigger for each affected row or once per statement.
Importance of Triggers
Triggers are essential for maintaining data integrity and automating repetitive tasks in PostgreSQL. For instance, they can automatically log changes to a table, enforce constraints, or even update related tables without requiring additional application logic.
PL/pgSQL for Trigger Functions
The most commonly used language for writing trigger functions in PostgreSQL is PL/pgSQL, which allows for complex logic and control structures. Understanding the syntax and structure of PL/pgSQL is crucial to leveraging the full capabilities of triggers.
Setting Up Your Environment for Trigger Implementation
Before diving into trigger implementation, it is crucial to set up your PostgreSQL environment properly. Here are the necessary steps:
1. Configure PostgreSQL Server
Ensure that your PostgreSQL server is correctly installed and configured. You can use tools like psql or pgAdmin to manage your databases and execute SQL commands.
2. User Permissions
Proper user permissions are essential when creating and managing triggers. Ensure that your user role has the TRIGGER
privilege on the relevant tables.
3. Development Database
Set up a development database to safely test your trigger logic without affecting production data. This allows for experimentation and debugging.
4. Backup Strategy
Implement a robust backup strategy before deploying triggers in a production environment. Triggers can modify data automatically, so it is crucial to have a recovery plan.
5. Using Chat2DB
Consider using Chat2DB (opens in a new tab), a user-friendly platform for managing PostgreSQL databases. Chat2DB offers AI-powered features, such as natural language SQL generation and smart query optimization, making it easier to write SQL scripts and handle complex queries.
Creating Basic Triggers in PostgreSQL
Creating a basic trigger in PostgreSQL involves several steps, including defining a trigger function and associating it with a table. Here’s how to do it:
Step 1: Create a Trigger Function
Below is a simple example of a trigger function that logs changes to a table named employees
.
CREATE OR REPLACE FUNCTION log_employee_changes()
RETURNS TRIGGER AS $$
BEGIN
INSERT INTO employee_log (employee_id, action, change_time)
VALUES (NEW.id, TG_OP, NOW());
RETURN NEW;
END;
$$ LANGUAGE plpgsql;
Step 2: Create the Trigger
Once the trigger function is defined, you can create the trigger itself:
CREATE TRIGGER employee_changes
AFTER INSERT OR UPDATE OR DELETE ON employees
FOR EACH ROW EXECUTE FUNCTION log_employee_changes();
Explanation of the Code
- The
log_employee_changes
function logs the employee ID, the action performed (INSERT, UPDATE, DELETE), and the timestamp of the change. - The
CREATE TRIGGER
statement associates the trigger with theemployees
table and specifies that it should execute the function after any row-level INSERT, UPDATE, or DELETE operation.
Best Practices
- Use descriptive names for your triggers and functions to make their purpose clear.
- Keep trigger functions simple and focused on a single task to enhance maintainability.
- Avoid complex logic within triggers to prevent performance issues.
Advanced Trigger Techniques and Best Practices
As you become more familiar with triggers, you may want to explore advanced techniques. Here are some key considerations:
Conditional Logic
Triggers can include conditional logic to handle various scenarios. For example:
CREATE OR REPLACE FUNCTION check_employee_salary()
RETURNS TRIGGER AS $$
BEGIN
IF NEW.salary < 0 THEN
RAISE EXCEPTION 'Salary cannot be negative';
END IF;
RETURN NEW;
END;
$$ LANGUAGE plpgsql;
Transition Tables
In AFTER triggers, you can access both the old and new states of the data using transition tables. This is useful for auditing changes or enforcing complex business rules.
Recursive Triggers
Be cautious when using recursive triggers, as they can lead to infinite loops. To prevent this, you can use a flag or a counter to limit recursion.
Performance Considerations
Triggers can impact performance, especially in high-traffic databases. Monitor trigger execution time and optimize your SQL queries accordingly. Utilize PostgreSQL's EXPLAIN and ANALYZE tools to identify performance bottlenecks.
Integrating Triggers with JSON Data in PostgreSQL
PostgreSQL's ability to handle JSON data types allows for innovative trigger implementations. You can use triggers to manipulate JSON data stored in tables, enhancing data processing capabilities.
Example Trigger for JSON Data
Consider a scenario where you store user preferences in a JSON column. You can create a trigger to extract specific elements from the JSON array whenever a new record is inserted:
CREATE OR REPLACE FUNCTION process_user_preferences()
RETURNS TRIGGER AS $$
BEGIN
-- Extract the first preference from the JSON array
NEW.first_preference := (SELECT value FROM json_array_elements(NEW.preferences) LIMIT 1);
RETURN NEW;
END;
$$ LANGUAGE plpgsql;
CREATE TRIGGER extract_first_preference
BEFORE INSERT ON user_preferences
FOR EACH ROW EXECUTE FUNCTION process_user_preferences();
Use Cases for JSON-based Triggers
- Dynamic Data Validation: Automatically validate or transform JSON data during insertions.
- Event Logging: Log changes to JSON columns for auditing purposes.
Challenges with JSON Structures
Working with complex JSON structures can present challenges. Ensure that your triggers handle various JSON formats gracefully. Use PostgreSQL's JSON functions and operators to extract and manipulate data effectively.
Testing and Debugging Triggers in PostgreSQL
Testing and debugging triggers is crucial for ensuring their reliability. Here are some strategies:
Writing Test Cases
Develop comprehensive test cases that cover all possible scenarios for your triggers. This includes edge cases and error conditions.
Debugging Techniques
Utilize RAISE
statements within your trigger functions to output debug information. This can help you identify issues during trigger execution.
Monitoring Logs
PostgreSQL logs can provide insights into trigger activity. Monitor these logs to diagnose issues or performance problems.
Validation Checklist
Create a checklist for validating trigger behavior, including:
- Ensuring triggers fire as expected
- Verifying data integrity after trigger execution
- Monitoring performance and resource usage
Optimizing Triggers for Performance
To ensure that your triggers do not negatively affect database performance, consider the following optimization strategies:
Writing Efficient Trigger Functions
Minimize the use of expensive operations within your trigger functions. For example, avoid complex queries or external calls that can slow down execution.
Profiling with EXPLAIN
Use the EXPLAIN command to analyze the performance of SQL statements within your trigger functions. This helps identify slow operations that need optimization.
Regular Monitoring
Implement regular monitoring of your triggers, especially in high-traffic environments. Adjust and tune your triggers as necessary to maintain optimal performance.
Leveraging Chat2DB
With Chat2DB (opens in a new tab), you can analyze and optimize SQL queries more effectively. The AI-driven features of Chat2DB streamline the process of debugging and profiling SQL, making it easier to enhance trigger performance compared to other tools.
FAQ
-
What are triggers in PostgreSQL? Triggers are procedures that automatically execute in response to certain events on a table or view in PostgreSQL.
-
What are the types of triggers available? The main types of triggers are BEFORE, AFTER, and INSTEAD OF, which can be applied at the row level or statement level.
-
How can I optimize trigger performance? You can optimize trigger performance by minimizing complex operations, profiling with EXPLAIN, and regularly monitoring trigger activity.
-
What is the role of PL/pgSQL in triggers? PL/pgSQL is the primary language used for writing trigger functions in PostgreSQL, allowing for complex logic and control structures.
-
How can Chat2DB assist with PostgreSQL triggers? Chat2DB provides AI-powered features that simplify database management, making it easier to write SQL scripts and optimize trigger performance effectively.
By effectively implementing triggers in PostgreSQL, you can automate processes, enforce data integrity, and enhance your database functionality. Utilize tools like Chat2DB (opens in a new tab) to streamline your database management tasks and make the most of your PostgreSQL environment.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!