How to Effectively Implement CREATE SEQUENCE in SQL: A Comprehensive Guide
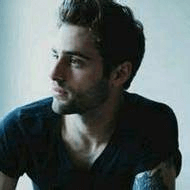
Understanding CREATE SEQUENCE in SQL
The CREATE SEQUENCE
command in SQL is essential for generating unique numeric identifiers within a database. This command allows the creation of a sequence, a database object designed to produce a sequence of numeric values based on specified rules. Utilizing sequences is critical for maintaining data integrity and ensuring that each record can be uniquely identified, especially in large databases where data consistency is vital.
What Is a Sequence?
A sequence is a straightforward database object that generates sequential numbers. Typically, sequences are employed for primary keys in tables, ensuring that each row has a distinct identifier. Various database systems, including PostgreSQL (opens in a new tab), Oracle (opens in a new tab), and SQL Server (opens in a new tab), implement sequences with slight syntax variations, but the fundamental concept remains consistent across platforms.
Importance of Sequences
Using sequences provides numerous advantages over other unique identifier generation methods, such as UUIDs or application-level counters. The key benefits of sequences include:
- Efficiency: Generated at the database level, sequences reduce application logic overhead.
- Consistency: Sequences guarantee uniqueness across transactions, preventing duplication or conflicts.
- Flexibility: Configurable to start at any number, increment by any value, and can even cycle back to the beginning after reaching a maximum value.
These advantages make sequences an invaluable tool for developers working with relational databases.
Setting Up Your Environment for CREATE SEQUENCE
To effectively implement sequences, developers must prepare their SQL environment, ensuring that the right tools and permissions are in place.
Installing and Configuring Chat2DB
One of the best tools for managing and visualizing database objects, including sequences, is Chat2DB (opens in a new tab). Chat2DB is an AI-driven database management tool that simplifies database interactions and enhances productivity through its unique features. Here’s how to get started:
- Download Chat2DB: Visit the Chat2DB website (opens in a new tab) and download the appropriate client for your operating system (Windows, Mac, or Linux).
- Install the Client: Follow installation instructions specific to your OS to get Chat2DB up and running.
- Connect to Your Database: Launch Chat2DB and connect to your desired database by entering the necessary credentials.
Chat2DB offers a user-friendly interface that makes it easy to manage database sequences without extensive SQL knowledge. Its AI features, including natural language processing capabilities, allow you to generate SQL commands simply by describing what you want in plain language.
Permissions and Access Control
Before creating sequences, ensure that your user account has the necessary permissions, typically the CREATE SEQUENCE
privilege in your database. Understanding user roles is crucial to avoid issues during sequence creation.
Choosing the Right Database Management System (DBMS)
Ensure your selected DBMS supports sequences. While most modern relational databases do, syntax may differ. Familiarize yourself with the SQL dialect of your chosen DBMS to ensure smooth sequence implementation.
Best Practices for Development Environment
Establish a development environment that closely mirrors your production setup. This practice prevents configuration drift and ensures that your sequence implementations will function correctly when deployed.
Creating a Basic Sequence
Once your environment is set up, you can start creating sequences. The CREATE SEQUENCE
command has a specific syntax to follow.
Syntax and Parameters
The basic syntax for creating a sequence is as follows:
CREATE SEQUENCE sequence_name
START WITH initial_value
INCREMENT BY increment_value
MINVALUE minimum_value
MAXVALUE maximum_value
CYCLE | NO CYCLE
CACHE cache_size | NO CACHE;
Detailed Example
Let’s walk through an example of creating a simple sequence:
CREATE SEQUENCE order_seq
START WITH 1
INCREMENT BY 1
MINVALUE 1
MAXVALUE 1000
NO CYCLE
CACHE 10;
In this example:
- START WITH 1: The sequence begins at 1.
- INCREMENT BY 1: Each subsequent value will increase by 1.
- MINVALUE 1: The smallest value the sequence can generate is 1.
- MAXVALUE 1000: The largest value the sequence can generate is 1000.
- NO CYCLE: The sequence will not restart after reaching the maximum value.
- CACHE 10: The database will cache 10 sequence values for quick access.
Default Values and Customization
Omitting certain parameters allows the sequence to use default values. For example, if you do not specify MINVALUE
or MAXVALUE
, most databases will set them to their respective defaults. Customizing these parameters is beneficial for specific application needs.
Using CACHE versus NO CACHE
The CACHE
option can significantly improve performance by pre-allocating sequence numbers. However, if the database crashes or a rollback occurs, cached values may be lost, leading to gaps in the sequence. It is essential to weigh performance benefits against the potential for gaps when deciding between CACHE
and NO CACHE
.
Naming Sequences
Choosing descriptive names for sequences is vital for maintainability, as it helps navigate complex database schemas.
Troubleshooting Common Errors
While creating sequences, you might encounter errors such as permission issues or syntax errors. Common troubleshooting steps include:
- Checking user permissions for
CREATE SEQUENCE
. - Verifying the correct database context.
- Ensuring that the sequence name does not conflict with existing objects.
Advanced Sequence Configuration
For more complex applications, you might need to configure sequences with advanced options.
Custom Increment Patterns
You can create sequences with custom increment patterns. For instance, to create a sequence that decrements instead of increments, specify a negative increment:
CREATE SEQUENCE reverse_seq
START WITH 100
INCREMENT BY -1
MINVALUE 1
MAXVALUE 100;
Handling Edge Cases
When a sequence reaches its maximum value, behavior can vary. If you want the sequence to restart from the minimum value after reaching the maximum, use the CYCLE
option:
CREATE SEQUENCE cycling_seq
START WITH 1
INCREMENT BY 1
MINVALUE 1
MAXVALUE 100
CYCLE;
Sequence Ownership and Alteration
After creating a sequence, you may need to alter its properties or transfer ownership. Use the ALTER SEQUENCE
command to modify aspects such as increment value or reset the current value:
ALTER SEQUENCE order_seq
INCREMENT BY 2;
Sequences in Distributed Environments
Managing sequences can become complex in distributed database environments. Strategies to minimize conflicts include:
- Using separate sequences for different database instances.
- Implementing a centralized sequence management service.
Sequences in Replication and Synchronization
Sequences play a role in database replication and synchronization. Ensure that sequence values are consistent across replicated databases to prevent primary key collisions.
Integrating Sequences into SQL Queries
Once sequences are created, they can be integrated into SQL queries to dynamically generate unique values.
Using NEXTVAL and CURRVAL
To retrieve the next value from a sequence, use the NEXTVAL
function:
INSERT INTO orders (order_id, order_date)
VALUES (order_seq.NEXTVAL, CURRENT_DATE);
To retrieve the current value of the sequence for the session, use the CURRVAL
function:
SELECT order_seq.CURRVAL;
Populating Primary Key Columns
Sequences are commonly used to automatically populate primary key columns, ensuring that each new record receives a unique identifier without manual intervention.
Handling Multi-threaded Environments
Using sequences in multi-threaded or concurrent environments can lead to race conditions. Ensure that sequence usage is properly synchronized across threads to mitigate these risks.
Using Sequences with Triggers and Stored Procedures
Sequences can work together with triggers and stored procedures. For example, a trigger can be created to automatically assign a sequence value to a primary key column when a new record is inserted.
Optimizing Queries with Sequences
When queries heavily rely on sequence-generated values, consider optimization strategies, such as indexing columns populated by sequences to enhance query performance.
Maintaining and Managing Sequences
Ongoing sequence maintenance is essential to ensure they meet business requirements.
Regular Sequence Audits
Conduct regular audits of your sequences to ensure alignment with current application needs. This includes checking current values, caching settings, and increment values.
Altering Sequences in Production
Exercise caution when altering sequences in a production environment. Make changes during low-usage times and inform stakeholders of planned adjustments.
Monitoring Sequence Performance
Monitoring sequence performance helps identify bottlenecks. Utilize tools like Chat2DB (opens in a new tab) for effective monitoring of sequence usage and performance metrics.
Handling Sequence Exhaustion
As sequences approach their maximum value, it is critical to have a plan in place for recycling or resetting the sequence. Implement alerts to notify administrators when thresholds are approached.
Backing Up and Restoring Sequences
Include sequences in your database backup strategy. Regularly back up sequences to prevent data loss and ensure quick recovery in case of failure.
Chat2DB Features for Sequence Management
Chat2DB offers several features that facilitate sequence monitoring and reporting, ensuring database administrators can manage sequences effectively and efficiently.
Common Challenges and Solutions with CREATE SEQUENCE
Working with sequences can present various challenges. Here are common issues and their solutions:
Challenge | Solution |
---|---|
Sequences may not be suitable | Consider using UUIDs for globally unique identifiers across databases. |
Sequence gaps | Understand the causes of gaps, such as rollbacks; implement proper transaction management. |
Synchronizing sequences | Maintain consistent sequence values across multiple instances to prevent conflicts. |
Permission-related errors | Review user roles and permissions to ensure necessary privileges are granted. |
Troubleshooting sequence corruption | Check for database-level corruption; validate sequence definition and parameters. |
Conclusion
By leveraging the capabilities of Chat2DB (opens in a new tab), you can simplify the management of sequences and other database objects while benefiting from its AI-driven features. Whether you're generating SQL commands or visualizing database structures, Chat2DB enhances your database management experience, making it a superior choice compared to traditional tools like DBeaver, MySQL Workbench, or DataGrip.
FAQs
1. What is the purpose of CREATE SEQUENCE in SQL?
CREATE SEQUENCE
generates unique numeric identifiers within a database, ensuring data integrity and consistency.
2. Can I use CREATE SEQUENCE in all database systems?
Most modern relational databases support CREATE SEQUENCE
, though syntax may vary. Refer to your specific DBMS documentation for details.
3. What happens if a sequence reaches its maximum value?
If a sequence reaches its maximum value and is configured with NO CYCLE
, it will throw an error. If configured with CYCLE
, it will restart from the minimum value.
4. How can I monitor sequence performance?
Tools like Chat2DB (opens in a new tab) provide monitoring features that allow you to effectively track sequence usage and performance.
5. What should I do if my sequence is not functioning correctly?
If you encounter issues with a sequence, check for permission issues, validate the sequence definition, and consider recreating the sequence if necessary.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!