How to Effectively Implement Constraints in SQL for Data Integrity
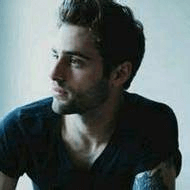
Understanding SQL Constraints for Ensuring Data Integrity
In the realm of database management, constraints in SQL play a pivotal role in maintaining data integrity. Data integrity refers to the accuracy, consistency, and reliability of the data stored in a database. Constraints ensure that the data adheres to certain rules and conditions, preventing invalid entries and maintaining the overall quality of the data. This is crucial for any application that relies on data accuracy, such as finance, healthcare, and e-commerce.
What are SQL Constraints?
SQL constraints are rules applied to table columns to restrict the type of data that can be stored in them. They are essential in enforcing business rules and ensuring that data entered into the database meets specific requirements. The primary types of SQL constraints include:
Constraint Type | Description |
---|---|
Primary Key | Ensures uniqueness for each record in a table. |
Foreign Key | Maintains referential integrity between tables. |
Unique | Guarantees all values in a column are distinct. |
Not Null | Prevents null values from being entered. |
Check | Allows for custom validation rules on data entries. |
Each of these constraints serves a unique purpose. For instance, primary key constraints are critical for uniquely identifying records, while foreign key constraints enforce relationships between tables, ensuring that the data remains connected and reliable.
Importance of SQL Constraints
Implementing constraints is crucial for preventing data anomalies and maintaining the integrity of the database. For example, when a foreign key constraint is applied, it ensures that any entry in the referencing table corresponds to an existing entry in the referenced table. This relationship is vital for maintaining referential integrity.
Constraints also help in enforcing business logic at the database level, which reduces the need for application-level checks and simplifies the overall architecture. This not only saves development time but also enhances performance, as the database engine can optimize operations based on these rules.
Here’s a simple example of creating a table with various constraints:
CREATE TABLE Employees (
EmployeeID INT PRIMARY KEY,
FirstName VARCHAR(50) NOT NULL,
LastName VARCHAR(50) NOT NULL,
Email VARCHAR(100) UNIQUE,
DepartmentID INT,
FOREIGN KEY (DepartmentID) REFERENCES Departments(DepartmentID)
);
In this example, EmployeeID
is a primary key, Email
has a unique constraint, and DepartmentID
is a foreign key referencing another table named Departments
.
Types of SQL Constraints and Their Applications
Understanding the various types of SQL constraints and their applications is essential for effective database design. Here’s a more detailed look at each constraint type:
Primary Key Constraints
A primary key uniquely identifies each record in a table. It cannot contain null values, ensuring that every entry is distinct. This is crucial for tables that require unique identification, such as user accounts or product listings.
CREATE TABLE Products (
ProductID INT PRIMARY KEY,
ProductName VARCHAR(255) NOT NULL,
Price DECIMAL(10, 2) NOT NULL
);
Foreign Key Constraints
Foreign key constraints establish a link between two tables. They ensure that a foreign key value in one table matches a primary key value in another table, maintaining referential integrity.
CREATE TABLE Orders (
OrderID INT PRIMARY KEY,
ProductID INT,
FOREIGN KEY (ProductID) REFERENCES Products(ProductID)
);
Unique Constraints
A unique constraint ensures that all values in a specified column are distinct, preventing duplicate entries. This is particularly useful for columns that must maintain unique identifiers, such as email addresses or usernames.
CREATE TABLE Users (
UserID INT PRIMARY KEY,
Username VARCHAR(50) UNIQUE,
Email VARCHAR(100) UNIQUE
);
Not Null Constraints
The not null constraint prevents null values from being entered into a specified column. This is important for columns that require a value for the application to function correctly, such as usernames or product prices.
CREATE TABLE Inventory (
ItemID INT PRIMARY KEY,
ItemName VARCHAR(255) NOT NULL,
Quantity INT NOT NULL
);
Check Constraints
Check constraints allow for custom validation rules to be applied to data entries. For example, you can ensure that a column value falls within a specific range.
CREATE TABLE Employees (
EmployeeID INT PRIMARY KEY,
Salary DECIMAL(10, 2) CHECK (Salary > 0)
);
Best Practices for Implementing Constraints in SQL
To effectively implement constraints in SQL, consider the following best practices:
Plan Your Database Schema
When designing your database schema, plan your constraints from the outset rather than adding them later. This proactive approach helps in maintaining data integrity from the beginning.
Thoroughly Test Constraints
It is essential to test your constraints thoroughly, including edge cases. This prevents unintentional data errors and ensures that your database functions as expected.
Use Descriptive Constraint Names
Using descriptive and meaningful names for your constraints enhances database readability and maintainability. This practice aids future developers in understanding the purpose of each constraint.
Balance Performance and Data Integrity
While constraints are vital for data integrity, they can sometimes impact performance. Explore strategies such as using indexed constraints to improve query performance.
Regularly Review and Update Constraints
As business requirements evolve, regularly review and update your constraints to ensure ongoing data integrity. This practice is crucial for adapting to changes in business logic or application requirements.
Common Challenges and Solutions in Constraint Implementation
Implementing constraints in SQL can present challenges. Here are some common issues and their solutions:
Handling Complex Business Rules
Complex business rules can complicate constraint implementation. Consider breaking down these rules into simpler constraints or using stored procedures to manage complex logic.
Migrating Legacy Systems
When migrating legacy systems, ensure that proper constraints are defined in the new database. This may involve data cleansing or transformation to fit the new schema.
Performance Concerns
In large databases, constraints can lead to performance issues. Consider optimization techniques such as indexing or partitioning to mitigate these concerns.
Managing Constraint Violations
When constraint violations occur, providing meaningful error messages can help users understand the issue and correct it. This improves user experience and reduces frustration.
Distributed Database Challenges
Maintaining data integrity in distributed SQL databases can be challenging. Implement strategies such as distributed transactions or eventual consistency models to address these challenges.
Utilizing Tools for Constraint Management
Tools like Chat2DB (opens in a new tab) can significantly simplify constraint management and ensure data integrity. Chat2DB is an AI-driven database management tool that enhances database operations through natural language processing and intelligent SQL generation. Its features enable developers and database administrators to manage constraints more effectively, reducing the likelihood of data integrity issues.
Code Example Demonstrating SQL Constraints
Here’s a comprehensive code example that demonstrates various types of constraints in SQL:
CREATE TABLE Customers (
CustomerID INT PRIMARY KEY,
FirstName VARCHAR(50) NOT NULL,
LastName VARCHAR(50) NOT NULL,
Email VARCHAR(100) UNIQUE,
CreatedAt DATETIME DEFAULT CURRENT_TIMESTAMP
);
CREATE TABLE Orders (
OrderID INT PRIMARY KEY,
CustomerID INT,
OrderDate DATETIME DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID)
);
CREATE TABLE Products (
ProductID INT PRIMARY KEY,
ProductName VARCHAR(255) NOT NULL,
Price DECIMAL(10, 2) NOT NULL CHECK (Price > 0)
);
In this example, we have created three tables: Customers
, Orders
, and Products
. Each table implements various constraints to enforce data integrity.
FAQ
1. What are SQL constraints?
SQL constraints are rules applied to table columns to restrict the type of data that can be stored in them, ensuring data integrity.
2. How many types of constraints are there in SQL?
There are several types of constraints in SQL, including primary keys, foreign keys, unique, not null, and check constraints.
3. Why are constraints important in SQL?
Constraints are important because they help maintain data integrity by preventing invalid data entries and ensuring relationships between tables are enforced.
4. How do I implement constraints in SQL?
Constraints can be implemented using SQL commands such as CREATE TABLE
and ALTER TABLE
, where you define the specific rules for each column.
5. How can Chat2DB help with SQL constraint management?
Chat2DB (opens in a new tab) offers AI-driven features that simplify database management, including the ability to easily implement and manage constraints, enhancing overall data integrity. With its intuitive interface, developers can generate SQL queries using natural language, making it easier to design and modify database structures.
By implementing these best practices and leveraging tools like Chat2DB, you can ensure effective management of constraints in SQL, leading to robust data integrity and reliability within your databases. Switch to Chat2DB today for a seamless database management experience that outperforms traditional tools like DBeaver, MySQL Workbench, and DataGrip.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!