How to Efficiently Execute Oracle SQL Insert Statements
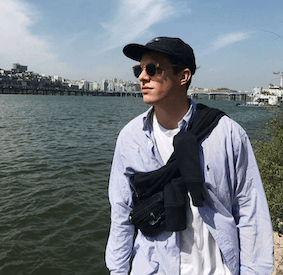
Understanding Oracle SQL Insert Statements
Oracle SQL Insert statements are fundamental commands used to add new records to a table within a database. The Insert statement allows users to specify which table to add data to, as well as which columns the data will populate.
The basic syntax of an Insert statement is as follows:
INSERT INTO table_name (column1, column2, column3, ...)
VALUES (value1, value2, value3, ...);
Types of Insert Statements
There are a couple of distinct types of Insert statements, each serving specific use cases:
- Single-Row Insert: This is the most common type and is used when adding one record at a time. For example:
INSERT INTO employees (first_name, last_name, email)
VALUES ('John', 'Doe', 'john.doe@example.com');
- Multi-Row Insert: This allows the insertion of multiple records in one statement, which can enhance performance. Here’s an example:
INSERT ALL
INTO employees (first_name, last_name, email) VALUES ('Jane', 'Smith', 'jane.smith@example.com')
INTO employees (first_name, last_name, email) VALUES ('Peter', 'Jones', 'peter.jones@example.com')
SELECT * FROM dual;
Importance of Correct Table and Column Names
Specifying the correct table and column names is crucial. If you reference a table or column that does not exist, Oracle will return an error. Furthermore, if you insert data into the wrong column, it may lead to data integrity issues.
Data Types and Constraints
When inserting data, it is essential to consider data types and constraints. Each column in a table has a defined data type, and the data being inserted must match this type. Additionally, constraints like NOT NULL and UNIQUE must be respected to maintain data integrity.
Using Subqueries in Insert Statements
Subqueries can be employed in Insert statements to derive data from other tables. This technique is useful for inserting data that is calculated from existing records. For instance:
INSERT INTO employees (first_name, last_name, email)
SELECT first_name, last_name, email FROM temp_employees WHERE status = 'active';
The RETURNING Clause
The RETURNING clause can be used to retrieve values after an insertion. This is particularly useful when inserting records with auto-generated keys. For example:
INSERT INTO employees (first_name, last_name, email)
VALUES ('Alice', 'Johnson', 'alice.johnson@example.com')
RETURNING employee_id INTO :new_id;
Performance Implications
Using Insert statements incorrectly can have performance implications. Avoid inserting large volumes of data in one go unless necessary, as it can lead to locks and slow performance. Always evaluate and test your Insert statements to ensure optimal performance.
Optimizing Performance for Oracle SQL Insert Statements
To enhance the performance of Oracle SQL Insert statements, consider the following strategies:
Role of Indexes
Indexes improve data retrieval speed but can slow down Insert operations. When inserting data, Oracle must update the index, which can lead to overhead. Therefore, it may be beneficial to drop indexes before large Insert operations and recreate them afterward.
Batching Insert Operations
Batching multiple Insert operations reduces overhead and improves execution time. Instead of executing a separate Insert statement for each record, combine them into a single statement, as shown previously in the multi-row insert example.
Direct-Path Insert
Oracle’s Direct-Path Insert allows for high-performance data loading. This method bypasses certain checks, enabling faster loading of data. Use it when inserting large datasets:
INSERT /*+ APPEND */ INTO employees SELECT * FROM temp_employees;
Reducing Logging
Using the NOLOGGING option can improve Insert performance by reducing the amount of information written to the redo log. This is particularly useful for bulk operations:
ALTER TABLE employees NOLOGGING;
Mitigating Network Latency
Network latency can significantly affect performance, especially in distributed databases. Minimize this impact by grouping data transfers and reducing the number of round trips to the database.
Using Bind Variables
Bind variables enhance Insert efficiency and reduce the risk of SQL injection attacks. By using bind variables, you can prepare a statement once and execute it multiple times with different values:
INSERT INTO employees (first_name, last_name, email)
VALUES (:first_name, :last_name, :email);
Table Partitioning
Table partitioning can optimize Insert operations for large datasets. By dividing a table into smaller, more manageable pieces, Insert operations can be more efficient.
Best Practices for Writing Oracle SQL Insert Statements
Following best practices ensures efficient and error-free Oracle SQL Insert statements:
Use Explicit Column Lists
Always specify the columns in your Insert statement. This practice avoids errors and improves code readability:
INSERT INTO employees (first_name, last_name, email)
VALUES ('Michael', 'Brown', 'michael.brown@example.com');
Use Constants or Variables for Repetitive Values
Using constants or variables for repetitive Insert values maintains consistency and improves code maintainability.
Implement Transactions
Using transactions ensures data integrity and allows for rollback capabilities in case of errors. Always group related Insert statements within a transaction:
BEGIN
INSERT INTO employees (first_name, last_name, email) VALUES ('Lucy', 'Green', 'lucy.green@example.com');
INSERT INTO employees (first_name, last_name, email) VALUES ('Mark', 'White', 'mark.white@example.com');
COMMIT;
EXCEPTION
WHEN OTHERS THEN
ROLLBACK;
END;
Test in Development Environment
Always test your Insert statements in a development environment before deploying them to production. This practice helps catch errors early.
Exception Handling
Incorporate exception handling to manage errors during Insert operations. This ensures that your application can gracefully handle issues without crashing.
Monitor Insert Operations
Regularly monitor and analyze Insert operations to identify performance bottlenecks. This enables you to make necessary adjustments before performance issues become critical.
Respect Constraints and Triggers
Understand and respect database constraints and triggers during Insert operations. This prevents data integrity issues and ensures that your data remains consistent.
Leveraging Chat2DB for Streamlined Oracle SQL Insert Operations
Chat2DB is a powerful tool that enhances and simplifies Oracle SQL Insert operations. Its user-friendly interface assists developers in constructing Insert statements efficiently.
Managing Database Connections
Chat2DB effectively manages database connections and sessions, streamlining Insert operations. This feature minimizes the overhead associated with establishing connections each time an Insert operation is performed.
Syntax Highlighting and Error Checking
With syntax highlighting and error checking capabilities, Chat2DB helps reduce coding mistakes. This ensures that your Insert statements are correctly formatted and ready for execution.
Query Optimization Features
Chat2DB's query optimization features help identify and resolve performance issues in Insert statements. This capability is essential for maintaining optimal database performance.
Integration with Version Control Systems
Chat2DB integrates seamlessly with version control systems, aiding in tracking changes to Insert statement scripts. This functionality is crucial for collaborative development environments.
Automating Repetitive Insert Tasks
Chat2DB can automate repetitive Insert tasks, saving time and reducing errors. Automating these tasks enhances productivity and allows developers to focus on more complex operations.
Resources and Tutorials
Chat2DB provides a wealth of resources and tutorials to help users master Oracle SQL Insert operations. These materials are invaluable for both novice and experienced developers looking to improve their skills.
Advanced Techniques for Oracle SQL Insert Statements
To further enhance the functionality and efficiency of Oracle SQL Insert statements, consider these advanced techniques:
Conditional Insert with MERGE Statement
The MERGE statement allows for conditional Insert operations, enabling simultaneous inserts and updates based on certain conditions:
MERGE INTO employees e
USING temp_employees t
ON (e.email = t.email)
WHEN MATCHED THEN
UPDATE SET e.first_name = t.first_name, e.last_name = t.last_name
WHEN NOT MATCHED THEN
INSERT (first_name, last_name, email) VALUES (t.first_name, t.last_name, t.email);
Insert All for Multiple Tables
The Insert All feature enables inserting data into multiple tables with a single statement, streamlining data loading processes:
INSERT ALL
INTO employees (first_name, last_name, email) VALUES ('Emma', 'Davis', 'emma.davis@example.com')
INTO contractors (first_name, last_name, email) VALUES ('Sam', 'Wilson', 'sam.wilson@example.com')
SELECT * FROM dual;
PL/SQL Blocks for Complex Logic
For complex Insert logic, PL/SQL blocks can be beneficial. They allow for more advanced programming constructs while performing Insert operations.
Insert Triggers
Insert triggers automate post-insertion tasks, such as logging changes or enforcing business rules. These can enhance data integrity and application logic.
Materialized Views for Reporting
Using materialized views can optimize Insert operations for reporting and analytics, improving overall performance.
Temporary Tables
Temporary tables can be beneficial in Insert operations, particularly for staging data before final insertion into production tables.
Global vs. Local Indexes
Understanding the implications of using global versus local indexes in Insert-heavy applications can guide you in optimizing your database design for performance.
By implementing these advanced techniques, database professionals can significantly enhance the efficiency and functionality of their Oracle SQL Insert operations.
To further enhance your knowledge and streamline your Oracle SQL Insert operations, consider utilizing Chat2DB. This tool not only simplifies the process but also provides resources to help you master your database management tasks.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!