PL/SQL in Oracle: Complete Guide to Database Procedures & Functions
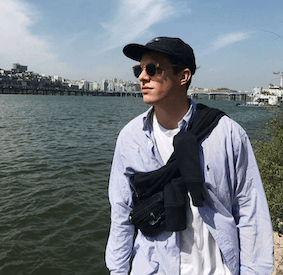
Oracle PL/SQL is a robust procedural language extension for SQL, specifically designed to manage databases effectively. It integrates SQL with procedural constructs, making it a powerful tool for developers looking to handle complex database interactions. PL/SQL allows for the automation of routine tasks and helps maintain data integrity across various applications.
One of the key aspects of PL/SQL is its block structure, which encapsulates variables, constants, and code logic. This organization enhances readability and maintainability of code, enabling developers to write clear and concise programs. Additionally, PL/SQL supports error handling, which is critical for ensuring reliability in database applications. It allows developers to catch and manage exceptions, thus preventing the application from crashing due to unhandled errors.
Another advantage of PL/SQL is its flexibility in embedding SQL commands within procedural code. This capability boosts performance and allows for more complex operations that traditional SQL cannot achieve alone. PL/SQL is extensively used in Oracle Database applications, making it a staple for Oracle developers looking to streamline their database management tasks.
Key Components and Features of PL/SQL
PL/SQL consists of several fundamental components, each playing a vital role in database programming. These include:
Anonymous Blocks
Anonymous blocks are unnamed PL/SQL code blocks that can be executed immediately. They are useful for quick tests or one-off operations.
DECLARE
v_employee_name VARCHAR2(100);
BEGIN
SELECT employee_name INTO v_employee_name FROM employees WHERE employee_id = 101;
DBMS_OUTPUT.PUT_LINE('Employee Name: ' || v_employee_name);
END;
/
Stored Procedures and Functions
Stored procedures are named PL/SQL blocks that can be stored in the database and executed as needed. Functions are similar but always return a value.
CREATE OR REPLACE FUNCTION get_employee_name(emp_id IN NUMBER) RETURN VARCHAR2 IS
v_employee_name VARCHAR2(100);
BEGIN
SELECT employee_name INTO v_employee_name FROM employees WHERE employee_id = emp_id;
RETURN v_employee_name;
END;
/
Triggers
Triggers are special types of stored procedures that automatically execute in response to certain events on a particular table or view.
CREATE OR REPLACE TRIGGER update_salary
AFTER UPDATE OF salary ON employees
FOR EACH ROW
BEGIN
DBMS_OUTPUT.PUT_LINE('Salary updated for employee: ' || :OLD.employee_id);
END;
/
PL/SQL Packages
Packages group logically related PL/SQL types, items, and subprograms, providing better organization and encapsulation.
Cursors
Cursors are used to process individual rows returned by database queries. They allow for row-by-row processing, which is especially useful in complex data handling scenarios.
DECLARE
CURSOR employee_cursor IS SELECT employee_id, employee_name FROM employees;
v_employee employees%ROWTYPE;
BEGIN
OPEN employee_cursor;
LOOP
FETCH employee_cursor INTO v_employee;
EXIT WHEN employee_cursor%NOTFOUND;
DBMS_OUTPUT.PUT_LINE('Employee ID: ' || v_employee.employee_id || ', Name: ' || v_employee.employee_name);
END LOOP;
CLOSE employee_cursor;
END;
/
Error Handling
PL/SQL has a robust error handling mechanism through exceptions, allowing developers to manage errors gracefully.
BEGIN
-- Some PL/SQL operations
EXCEPTION
WHEN NO_DATA_FOUND THEN
DBMS_OUTPUT.PUT_LINE('No data found for the given criteria.');
WHEN OTHERS THEN
DBMS_OUTPUT.PUT_LINE('An unexpected error occurred: ' || SQLERRM);
END;
/
Through its various components, PL/SQL provides a comprehensive framework for database programming, enabling developers to create efficient, reliable, and maintainable database applications.
Optimizing Database Performance with PL/SQL
Optimizing database performance is essential for any application that relies on a database. PL/SQL offers several strategies to enhance performance:
Bulk Processing Techniques
Using bulk processing techniques such as BULK COLLECT
and FORALL
can significantly reduce context switching between SQL and PL/SQL.
DECLARE
TYPE emp_tab IS TABLE OF employees%ROWTYPE;
v_employees emp_tab;
BEGIN
SELECT * BULK COLLECT INTO v_employees FROM employees;
FORALL i IN 1..v_employees.COUNT
UPDATE employees SET salary = salary * 1.1 WHERE employee_id = v_employees(i).employee_id;
END;
/
Caching Query Results
PL/SQL can cache query results to allow for faster data retrieval. By storing frequently accessed data in collections, applications can reduce database hits.
Efficient Algorithms
Optimizing PL/SQL code by avoiding unnecessary computations and utilizing efficient algorithms can lead to better performance.
Bind Variables
Using bind variables helps reduce parsing overhead, which is crucial for performance in applications that run similar queries repeatedly.
Data Types and Indexing
Choosing appropriate data types and implementing effective indexing strategies can significantly enhance query performance.
Minimizing I/O Operations
Minimizing I/O operations through effective PL/SQL programming techniques can lead to better performance.
Built-in Performance Tuning Tools
Oracle provides built-in performance tuning tools for PL/SQL, allowing developers to analyze and optimize their code effectively.
Efficient Error Handling in PL/SQL
Error handling is a critical aspect of PL/SQL development. A robust error handling mechanism ensures that applications remain stable even when unexpected issues arise.
Exception Handling Model
PL/SQL's exception handling model allows developers to define custom error messages and manage errors effectively.
Predefined Exceptions
Using predefined exceptions for common Oracle errors can simplify error handling and make the code cleaner.
User-defined Exceptions
Creating user-defined exceptions for specific scenarios can enhance the flexibility of error management.
Logging Errors
Logging error information is vital for debugging and auditing purposes. It allows developers to analyze issues and improve application reliability.
Graceful Error Handling
Implementing strategies for gracefully handling errors ensures that applications can continue to function even in the presence of errors.
Reusable Error Handling
Encapsulating error handling within PL/SQL packages promotes reusability and consistency across applications.
Integrating PL/SQL with Chat2DB for Enhanced Functionality
Chat2DB is a powerful tool designed for efficient database management that seamlessly integrates with PL/SQL. This integration enhances functionality and simplifies the development process.
Executing and Testing PL/SQL Code
Chat2DB facilitates the execution and testing of PL/SQL code, allowing developers to verify their logic quickly.
User-friendly Interface
The user-friendly interface of Chat2DB makes managing PL/SQL scripts and database objects more accessible, reducing the learning curve for new developers.
Debugging Features
Chat2DB's debugging features help developers identify and fix issues in PL/SQL code effectively, leading to more robust applications.
Automation Capabilities
Using Chat2DB's automation capabilities can streamline PL/SQL development workflows, making it easier to manage
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!