How to Properly Format SQL for Enhanced Readability and Performance
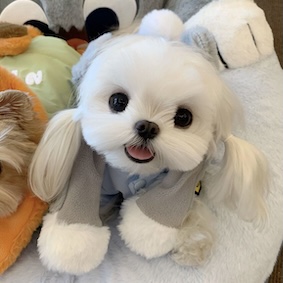
Properly formatting SQL (Structured Query Language) is essential for enhancing both readability and performance in database management. This article explores the critical aspects of SQL formatting, including its significance in writing clear and efficient queries, best practices to follow, and tools to assist developers in maintaining high-quality code. By understanding how to format SQL correctly, developers can improve collaboration, simplify debugging, and optimize performance. This article covers various formatting elements such as indentation, capitalization, line breaks, and whitespace, as well as the roles of tools like Chat2DB (opens in a new tab), an AI-driven database management tool that simplifies SQL formatting and enhances productivity.
Understanding SQL Formatting
SQL serves as the backbone of relational database management systems, enabling developers to interact with data effectively. Properly formatted SQL not only improves readability but also plays a vital role in optimizing performance. When SQL queries are well-structured, they become easier for developers to read, maintain, and debug.
The Importance of SQL Formatting
Misformatted SQL can lead to complex queries that are prone to errors. Developers often face challenges such as inconsistent styling, convoluted logic, and difficulty in collaboration. By adhering to established SQL formatting conventions, developers can ensure their code is consistent and easier to understand. Tools like Chat2DB (opens in a new tab) leverage AI to assist in formatting SQL, making it easier to write and manage queries effectively.
Common SQL Formatting Issues
Some of the most common formatting issues include:
Issue | Description |
---|---|
Complex Queries | Long queries without proper indentation can be hard to follow. |
Inconsistent Styling | Varying styles within a project can confuse team members. |
Lack of Comments | Queries without explanations can lead to misunderstandings. |
Adopting SQL style guides can mitigate these issues, ensuring a unified approach across projects.
Key Elements of SQL Formatting
The clarity and efficiency of SQL code are significantly influenced by several formatting elements. Understanding and implementing these components can greatly enhance the readability of SQL queries.
Indentation
Indentation is a visual aid that separates different parts of a SQL query. By using consistent indentation, developers can make it easier to identify the structure of the query. For example:
SELECT
first_name,
last_name
FROM
employees
WHERE
department = 'Sales'
ORDER BY
last_name;
Capitalization
Capitalizing SQL keywords distinguishes them from other identifiers, such as column and table names. This practice not only improves readability but also makes it clear which parts of the query are instructions. For instance:
SELECT
first_name,
last_name
FROM
Employees
WHERE
Salary > 50000;
Line Breaks
Strategically placing line breaks can improve the readability of long queries. Breaking lines at appropriate points helps in visually separating different clauses. Consider the following example:
SELECT
department,
COUNT(*) AS employee_count
FROM
employees
WHERE
hire_date >= '2020-01-01'
GROUP BY
department
ORDER BY
employee_count DESC;
Whitespace
Whitespace plays a crucial role in separating SQL elements and enhancing clarity. Using spaces effectively can help distinguish different parts of a query, making it easier to read and understand.
Aliasing
Using meaningful aliases for tables and columns can help avoid confusion. For example:
SELECT
e.first_name AS first,
e.last_name AS last
FROM
employees AS e
WHERE
e.department = 'HR';
Comment Usage
Incorporating comments within SQL queries can clarify complex logic and enhance maintainability. For example:
SELECT
first_name,
last_name
FROM
employees
WHERE
department = 'IT' -- Only select IT department employees
ORDER BY
last_name;
SQL Formatting Best Practices
Following best practices in SQL formatting ensures that code remains clear and efficient. Here are some guidelines to adhere to:
Consistent Style
Maintain a consistent style across all SQL queries within a project. This consistency fosters better collaboration and understanding among team members.
Meaningful Names
Use descriptive names for tables, columns, and aliases. This practice aids in understanding the purpose of each element within the query.
Grouping Related Clauses
Grouping related SQL clauses together enhances organization and understanding. For example:
SELECT
first_name,
last_name
FROM
employees
WHERE
department = 'Sales'
AND hire_date > '2020-01-01';
Logical Ordering of Clauses
Ordering SQL clauses logically (e.g., SELECT, FROM, WHERE, GROUP BY, HAVING, ORDER BY) improves clarity and understanding of the query's flow.
Simplifying Logic
Avoid unnecessary complexity in queries. Simplifying logic and breaking down complex operations into smaller steps can lead to clearer and more maintainable code.
Subqueries and Common Table Expressions (CTEs)
Using subqueries and CTEs can help simplify complex queries. For example:
WITH EmployeeCount AS (
SELECT
department,
COUNT(*) AS count
FROM
employees
GROUP BY
department
)
SELECT
department,
count
FROM
EmployeeCount
WHERE
count > 10;
Regular Reviews and Refactoring
Regularly review and refactor SQL code to ensure it remains clean and efficient. This practice helps identify areas for improvement and maintains high standards across the codebase.
Tools and Resources for SQL Formatting
Various tools and resources are available to aid developers in SQL formatting and optimization. Here are several options to consider:
Chat2DB
Chat2DB (opens in a new tab) is a powerful AI-driven database management tool that offers features for managing and formatting SQL databases. Its intelligent SQL editor assists developers in writing clean and well-structured queries, enhancing productivity and performance. The AI capabilities of Chat2DB allow for automated query generation, suggestion of optimizations, and real-time error detection, making it a superior choice for developers looking to streamline their database management tasks.
Popular SQL Formatting Tools
- SQL Formatter: A widely used tool for automatic code formatting.
- SQL Beautifier: Helps improve SQL readability by formatting code.
Integrated Development Environments (IDEs)
IDEs such as DataGrip and SQL Server Management Studio (SSMS) come with built-in SQL formatting support, making it easier for developers to maintain consistent formatting.
Extensions and Plugins
Text editors like Visual Studio Code offer extensions and plugins that enhance SQL formatting capabilities. These tools can assist in maintaining consistent styles and improving overall code quality.
Online Platforms and Communities
Accessing online platforms and communities can provide valuable SQL formatting tips and best practices. Websites like Stack Overflow and GitHub host discussions on SQL formatting challenges and solutions.
SQL Style Guides
Referencing SQL style guides can help standardize formatting across teams. Resources like the SQL Style Guide (opens in a new tab) provide comprehensive guidelines for maintaining clear and consistent SQL code.
Enhancing SQL Performance Through Formatting
Proper SQL formatting contributes significantly to performance optimization. Here are some ways in which formatting can impact performance:
Clear and Concise Queries
Well-formatted queries reduce execution time and resource consumption. By writing clear and concise SQL, developers can improve the efficiency of data retrieval.
Indexing and Query Optimization
Combining well-formatted SQL with indexing and query optimization techniques enhances performance. Properly structured queries can take full advantage of database indexing.
Avoiding Unnecessary Calculations
Minimizing unnecessary calculations and operations within queries is essential for performance. For example:
SELECT
first_name,
last_name
FROM
employees
WHERE
department = 'Sales'
AND YEAR(hire_date) >= 2020; -- Avoid unnecessary date calculations
Query Execution Plans
Understanding query execution plans is crucial for performance analysis. Well-formatted SQL aids in interpreting these plans, allowing developers to optimize queries effectively.
Continuous Monitoring and Profiling
Regularly monitoring and profiling SQL queries help maintain optimal performance. This practice identifies slow queries and areas for improvement.
Common SQL Formatting Pitfalls to Avoid
Developers should be aware of common pitfalls that can compromise SQL formatting. Here are several to avoid:
Inconsistent Formatting Styles
Inconsistent formatting styles can lead to confusion and hinder collaboration among team members. Adopting a unified approach is essential.
Excessively Complex Queries
Complex queries can become difficult to read and maintain. Simplifying logic and breaking queries into manageable parts is critical for clarity.
Hardcoded Values
Avoid using hardcoded values within queries. Opt for parameterized queries instead to enhance security and maintainability.
Improper Use of Aliases
Using aliases improperly can lead to confusion. Ensure they are meaningful and used consistently throughout the query.
Redundant SQL Clauses
Eliminate redundant or unnecessary SQL clauses that complicate queries. Concise queries are easier to read and maintain.
Lack of Comments
Failing to document complex queries can lead to misunderstandings. Always include comments to clarify logic and intent.
Leveraging SQL Formatting for Team Collaboration
Effective SQL formatting enhances collaboration among development teams. Here are some benefits of maintaining consistent formatting styles:
Seamless Code Integration
Consistent formatting facilitates seamless code integration and review processes. Team members can collaborate more effectively when code adheres to established styles.
Onboarding New Team Members
Well-formatted SQL code simplifies the onboarding process for new team members. Clear and understandable queries help them acclimate to the codebase quickly.
Comprehensive Documentation
Maintaining comprehensive documentation and comments is crucial for collaborative projects. This practice ensures that all team members understand the code's intent and functionality.
SQL Style Guides Alignment
Utilizing SQL style guides aligns team members on formatting standards. This alignment fosters consistency and improves overall code quality.
Version Control Systems
Using version control systems to manage and track changes in SQL code is essential. This practice allows teams to collaborate effectively while maintaining a history of modifications.
Regular Team Meetings
Conducting regular team meetings and workshops to address formatting challenges and share best practices can strengthen collaboration. Open discussions about SQL formatting can lead to improved standards and practices across the team.
In conclusion, mastering SQL formatting is vital for developers aiming to write clear, efficient, and maintainable code. By adhering to best practices, utilizing tools like Chat2DB (opens in a new tab), and continuously improving formatting skills, developers can significantly enhance the performance and readability of their SQL queries. Transitioning to Chat2DB not only streamlines the SQL formatting process but also empowers developers with AI capabilities that elevate their database management experience.
FAQ
-
What is SQL formatting? SQL formatting refers to the practice of structuring SQL queries to enhance readability, maintainability, and performance.
-
Why is SQL formatting important? Proper SQL formatting improves collaboration, simplifies debugging, and optimizes query performance.
-
What are some best practices for SQL formatting? Best practices include using consistent indentation, meaningful names, logical ordering of clauses, and maintaining clear comments.
-
How can tools like Chat2DB help with SQL formatting? Chat2DB offers AI-driven features that assist in automatic SQL formatting, making it easier for developers to write clean and efficient queries.
-
What common pitfalls should developers avoid when formatting SQL? Developers should avoid inconsistent formatting styles, excessively complex queries, hardcoded values, and lack of comments to maintain clarity and performance.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!