How to Efficiently Format SQL for Improved Readability and Performance
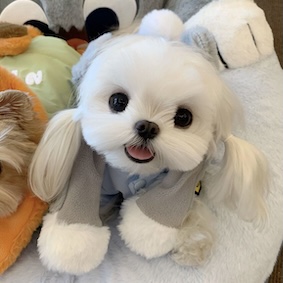
Efficiently formatting SQL is crucial for enhancing both readability and performance. Well-structured SQL code significantly reduces errors, facilitates collaboration among team members, and ensures maintainability. Moreover, clear formatting aids in debugging and error detection, while also improving query performance. In this article, we will explore various aspects of SQL formatting, including best practices, tools, techniques, and common mistakes to avoid. We will also introduce Chat2DB (opens in a new tab), an AI-driven database management tool that streamlines SQL formatting, making it easier for developers and database administrators to manage their data effectively.
Understanding the Importance of SQL Formatting
SQL formatting is not just about aesthetics; it plays a vital role in the development process. A well-formatted SQL query enhances readability (opens in a new tab), making it easier for developers to understand and modify code. This is especially important in collaborative environments where multiple team members may work on the same codebase.
Benefits of SQL Formatting
Benefit | Description |
---|---|
Reduced Errors | Proper formatting helps in identifying syntax errors and logical issues, reducing the likelihood of runtime errors. |
Improved Collaboration | A standardized formatting approach ensures that all team members can read and understand each other’s code, leading to better collaboration. |
Maintainability | Well-structured code is easier to maintain and update, which is essential for long-term projects. |
Debugging | Clear formatting makes it easier to spot errors, facilitating quicker debugging. |
SQL Beautification
The concept of SQL beautification (opens in a new tab) refers to the process of transforming SQL code into a more readable format. This often involves consistent indentation, capitalization of keywords, and organizing code into logical sections. The benefits of SQL beautification are manifold and include enhanced readability and reduced complexity in understanding the code.
SQL Formatting Best Practices
To ensure your SQL code is as readable and efficient as possible, follow these best practices:
Consistent Indentation and Alignment
Indenting your SQL code properly is crucial for readability. Here’s an example:
SELECT
employee_id,
first_name,
last_name
FROM
employees
WHERE
department_id = 10
ORDER BY
last_name;
In this example, the SELECT statement is aligned neatly, making it easier to scan for the necessary components.
Capitalization of SQL Keywords
Using uppercase for SQL keywords enhances readability. For example:
SELECT COUNT(*) AS total_employees
FROM employees
WHERE department_id = 10;
In this case, SELECT
, FROM
, and WHERE
are capitalized, making it clear that these are SQL commands.
Breaking Down Complex Queries
Complex queries should be broken down into smaller, manageable parts. Here’s a more complicated example:
SELECT
e.first_name,
e.last_name,
d.department_name
FROM
employees e
JOIN
departments d ON e.department_id = d.department_id
WHERE
d.location_id IN (SELECT location_id FROM locations WHERE city = 'New York')
ORDER BY
e.last_name;
This query is easier to read because it is structured in a way that separates different components clearly.
Use of Whitespace
Whitespace can be effectively used for visual separation of code elements. For example:
SELECT
employee_id,
first_name,
last_name
FROM
employees
WHERE
hire_date BETWEEN '2020-01-01' AND '2020-12-31';
Adding spaces between different clauses can help in visually distinguishing them.
Organizing Code with Comments
Using comments can clarify complex SQL code. For example:
-- This query retrieves employees hired in 2020
SELECT
employee_id,
first_name,
last_name
FROM
employees
WHERE
hire_date BETWEEN '2020-01-01' AND '2020-12-31';
Comments explain what the code does, making it easier for others to understand the intent.
Formatting Functions and Subqueries
Subqueries and functions should also be formatted for clarity. Here’s an example:
SELECT
department_id,
(SELECT COUNT(*) FROM employees WHERE department_id = d.department_id) AS employee_count
FROM
departments d;
In this case, the subquery is clearly defined, making it easier to follow.
Tools and Techniques for Efficient SQL Formatting
There are many tools available to assist with SQL formatting, but few offer the comprehensive capabilities of Chat2DB (opens in a new tab). This AI-driven database visualization management tool enhances SQL readability and performance through several innovative features.
Features of Chat2DB
- Natural Language SQL Generation: Chat2DB allows users to generate SQL queries using natural language, making it easier for non-technical users to interact with databases.
- Intelligent SQL Editor: The intelligent SQL editor provides real-time suggestions and corrections to help developers write better SQL code.
- Data Visualization: Users can visualize data directly from their SQL queries, which aids in understanding complex datasets.
- Automated Formatting: Chat2DB can automatically format SQL code, ensuring consistency and readability across all queries.
Benefits of Using Chat2DB over Other Tools
While other tools like DBeaver, MySQL Workbench, and DataGrip provide SQL formatting features, they often lack the advanced AI capabilities of Chat2DB. For instance, Chat2DB’s ability to understand natural language queries and generate SQL on-the-fly provides developers with a significant advantage, allowing for faster and more intuitive database management.
Real-Time Formatting
Real-time formatting is a crucial feature that allows developers to see the effects of their formatting changes immediately. This is particularly useful in collaborative environments where multiple team members may be working on the same code.
Plugins and Extensions
Many development environments support plugins and extensions that enhance SQL formatting capabilities. However, relying on Chat2DB's built-in features can offer a more streamlined and efficient experience.
Optimizing SQL for Improved Performance
The relationship between SQL formatting and query performance is significant. Properly formatted SQL can lead to more efficient execution plans, ensuring that your database operates at optimal levels.
Efficient Execution Plans
An efficient execution plan is crucial for database performance. Proper formatting helps databases generate better execution plans, which in turn leads to faster query execution. For example:
EXPLAIN SELECT
employee_id,
first_name,
last_name
FROM
employees
WHERE
department_id = 10;
Using the EXPLAIN
command allows developers to analyze how the SQL engine processes the query, highlighting areas for optimization.
Importance of Indexing
Indexing is a critical aspect of SQL performance. Properly formatted SQL queries make it easier to identify which columns should be indexed. For example:
CREATE INDEX idx_department_id
ON employees(department_id);
This statement creates an index on the department_id
column, which can significantly speed up queries filtering by that column.
Optimizing Joins and Subqueries
When dealing with joins and subqueries, formatting can help identify opportunities for optimization. For instance:
SELECT
e.first_name,
e.last_name
FROM
employees e
JOIN
departments d ON e.department_id = d.department_id
WHERE
d.location_id = 100;
In this example, the join condition is clearly laid out, making it easier to see how the tables are connected.
Query Refactoring
Refactoring queries for performance improvement is easier with well-formatted SQL. This process involves restructuring queries to improve efficiency without changing the results. For example, simplifying nested queries can enhance readability and performance.
Analyzing Execution Plans
Regularly analyzing execution plans can help identify performance bottlenecks. By using formatted SQL, developers can pinpoint which areas of their queries are causing slowdowns.
Database Resource Utilization
Properly formatted SQL can optimize database resource utilization. Efficient queries consume fewer resources, which is essential for maintaining performance as data volumes grow.
Common SQL Formatting Mistakes to Avoid
Understanding common formatting mistakes can help developers produce better SQL code. Some typical errors include:
Inconsistent Naming Conventions
Using inconsistent naming conventions can lead to confusion. For example, mixing singular and plural forms in table names can create ambiguity. Adhering to a consistent naming convention simplifies code.
Neglecting Whitespace and Indentation
Poor use of whitespace and indentation can make SQL code difficult to read. For instance, consider the following poorly formatted query:
SELECT employee_id,first_name,last_name FROM employees WHERE hire_date > '2020-01-01';
This query lacks clarity and is hard to follow.
Overly Complex and Nested Queries
Overly complex queries can lead to confusion and increased debugging time. Breaking down complex queries into simpler components is advisable for easier understanding.
Inconsistent Keyword Capitalization
Inconsistently capitalizing SQL keywords can hinder readability. Adopting a standard approach—such as capitalizing all SQL keywords—helps maintain clarity.
Lack of Comments and Documentation
Failing to document SQL code can lead to misunderstandings among team members. Adding comments to explain complex logic or assumptions can greatly enhance code clarity.
Consequences of Formatting Mistakes
These common mistakes can lead to increased debugging time, errors, and reduced collaboration among team members. By avoiding these pitfalls, developers can enhance their SQL coding practices.
Case Studies and Real-world Examples
Analyzing real-world examples of well-formatted SQL code can provide valuable insights. For instance, consider a case where a poorly formatted query was transformed into a well-structured one, leading to improved performance and team collaboration.
Success Stories with Chat2DB
Many developers have reported success using Chat2DB (opens in a new tab) for SQL formatting. The intuitive interface and AI-driven features have made it easier for teams to manage their databases effectively.
Industry Practices and Standards
Adhering to industry practices and standards for SQL formatting can enhance collaboration and reduce errors. Organizations that implement standardized formatting guidelines see improved productivity and fewer misunderstandings.
Lessons from Large-scale Database Projects
Large-scale projects often highlight the importance of SQL formatting. Teams that prioritize clear formatting tend to experience fewer issues during development and maintenance phases.
Developer Insights
Developers who emphasize SQL formatting often report increased efficiency and reduced debugging time. The ability to work with well-structured queries allows teams to focus on delivering value rather than fixing formatting issues.
Further Enhancing SQL Skills
To continue improving your SQL formatting skills, consider the following resources:
Books and Online Courses
Numerous books and online courses focus on SQL best practices. These resources can provide deeper insights into effective SQL formatting techniques.
Community Forums and Discussion Groups
Engaging with community forums and discussion groups can foster knowledge sharing and collaboration. Platforms like Stack Overflow and Reddit offer valuable insights from experienced developers.
Continuous Learning
Staying updated with industry trends and best practices is essential for any SQL developer. Continuous learning can lead to improved coding practices and a deeper understanding of SQL.
Certification Programs
Consider pursuing certification programs that emphasize SQL proficiency. These programs can enhance your resume and demonstrate your commitment to mastering SQL.
Mentorship and Peer Reviews
Seeking mentorship and participating in peer reviews can provide constructive feedback on your SQL code. This collaborative approach can help you develop better coding habits.
Contributing to Open-source Projects
Engaging with open-source projects allows you to apply your skills in real-world scenarios. This hands-on experience can significantly boost your SQL formatting abilities.
By adopting these practices and leveraging tools like Chat2DB (opens in a new tab), you can enhance your SQL skills and produce more efficient, readable code.
FAQ
-
What is SQL formatting? SQL formatting is the practice of structuring SQL code to improve its readability and maintainability.
-
Why is SQL formatting important? Proper SQL formatting reduces errors, enhances collaboration, and improves query performance.
-
What are the best practices for SQL formatting? Best practices include consistent indentation, capitalization of keywords, breaking down complex queries, and using comments.
-
How can Chat2DB help with SQL formatting? Chat2DB offers an intelligent SQL editor, natural language query generation, and automated formatting to streamline SQL management.
-
What common mistakes should I avoid in SQL formatting? Common mistakes include inconsistent naming conventions, neglecting whitespace, and failing to document code effectively.
By choosing Chat2DB (opens in a new tab), you can experience a superior SQL formatting tool that integrates AI features, setting a new standard in database management.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!