Effectively Utilizing MySQL LIMIT for Optimal Query Performance
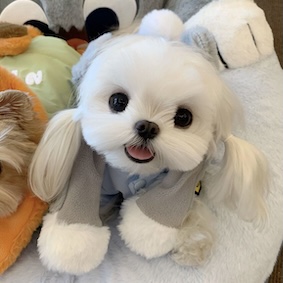
In this comprehensive article, we will delve into the MySQL LIMIT clause, a fundamental tool in database management that enables you to control the number of records returned by a query. Mastering the use of the LIMIT clause, particularly in conjunction with techniques such as OFFSET, can significantly enhance query performance and optimize your database operations. We will provide practical examples, discuss its implications on performance, and highlight best practices. Additionally, we will introduce Chat2DB, an AI-powered database management tool that simplifies these processes and improves overall efficiency.
Understanding the MySQL LIMIT Clause
The LIMIT clause in MySQL is designed to restrict the number of rows returned in a query result. This capability is particularly useful in scenarios such as pagination, where displaying all records at once can overwhelm users and consume unnecessary resources. The basic syntax of the LIMIT clause is straightforward:
SELECT * FROM table_name LIMIT number_of_rows;
In this example, number_of_rows
specifies how many rows you want to retrieve from the dataset. You can also use OFFSET to skip a specified number of rows before starting to return rows:
SELECT * FROM table_name LIMIT number_of_rows OFFSET offset_value;
Use Cases for LIMIT
Use Case | Example SQL Query |
---|---|
Pagination | SELECT * FROM products LIMIT 10 OFFSET 0; |
Data Sampling | SELECT * FROM sales LIMIT 100; |
-
Pagination: In web applications, displaying data in pages rather than all at once improves user experience. For example, if you have a list of products, you might want to show only 10 products per page. This can be achieved with:
SELECT * FROM products LIMIT 10 OFFSET 0; -- First page SELECT * FROM products LIMIT 10 OFFSET 10; -- Second page
-
Sampling Data: When analyzing large datasets, sometimes you only need a subset of the data. Using LIMIT, you can easily extract a sample for your analysis:
SELECT * FROM sales LIMIT 100;
Combining LIMIT with ORDER BY
When using LIMIT, it's crucial to pair it with ORDER BY to ensure consistent and predictable results. Without an explicit order, the results returned can vary, leading to confusion and potential data integrity issues. For instance:
SELECT * FROM customers ORDER BY created_at DESC LIMIT 5;
This query retrieves the latest 5 customers added to the database.
Performance Considerations
Using the LIMIT clause can significantly enhance performance by reducing the workload on the database server. When you limit the number of rows returned, the database engine can execute queries faster and consume fewer memory resources. However, to maximize these benefits, consider the following:
-
Indexing: Proper indexing can further improve query performance when used in conjunction with LIMIT. For example, if you frequently query the latest records, indexing the corresponding columns will speed up retrieval times.
-
Caching: Implementing caching strategies can also boost application performance when using LIMIT. By caching common queries that utilize the LIMIT clause, you can reduce the number of times the database needs to be queried for the same data.
Here’s a simple benchmarking example to illustrate performance with and without LIMIT:
-- Without LIMIT
SELECT * FROM large_table;
-- With LIMIT
SELECT * FROM large_table LIMIT 1000;
In this case, the second query will execute significantly faster due to the reduced data retrieval.
Optimizing Performance with LIMIT
To further enhance query performance with the LIMIT clause, consider the following strategies:
- Avoid Large OFFSET Values: When using OFFSET, large values can slow down the query because the database must process all preceding rows. Instead, consider using keyset pagination, which relies on the last retrieved record's unique identifier to fetch the next set of results:
SELECT * FROM customers WHERE id > last_seen_id LIMIT 10;
- Batch Processing: For operations involving large datasets, breaking your queries into smaller batches can help manage system load:
SELECT * FROM orders LIMIT 100 OFFSET 0; -- First batch
SELECT * FROM orders LIMIT 100 OFFSET 100; -- Second batch
Common Mistakes with LIMIT
One common pitfall when using LIMIT is neglecting to include an ORDER BY clause, which can lead to unpredictable results. Always ensure that your queries are ordered appropriately to maintain consistency.
Another mistake is using LIMIT in write-heavy environments without considering the implications of changing data. If your dataset is constantly being updated, you may want to implement locking mechanisms or use read replicas to avoid inconsistencies.
Advanced Usage Patterns for LIMIT
As you become more comfortable with the LIMIT clause, you can explore advanced usage patterns that can further optimize query performance:
LIMIT in Subqueries
Using LIMIT within subqueries can help streamline complex queries. For instance, you might want to retrieve the top 5 sales representatives based on sales figures:
SELECT * FROM (
SELECT name, SUM(sales) AS total_sales
FROM sales_data
GROUP BY name
ORDER BY total_sales DESC
LIMIT 5
) AS top_sales_reps;
LIMIT with JOIN Operations
When working with JOIN operations, applying LIMIT can help optimize performance. For example, if you want to fetch the top products sold by each category, you can combine JOIN and LIMIT:
SELECT p.name, c.category_name
FROM products p
JOIN categories c ON p.category_id = c.id
ORDER BY p.sales DESC
LIMIT 10;
Pagination with LIMIT and OFFSET
Implementing pagination in web applications is crucial for managing large datasets. The LIMIT and OFFSET combination is commonly used to achieve this:
Best Practices for Pagination
-
Consistent User Experience: Always maintain the order of records to ensure users see the same items in the same order when navigating pages.
-
Handling Data Changes: If underlying data changes while users are paginating, consider strategies for maintaining a consistent view. One approach is to use snapshotting or versioning of data.
-
RESTful APIs: When building APIs that support pagination, utilize LIMIT and OFFSET effectively to enhance data consumption:
GET /api/products?limit=10&offset=20
Code Examples for Pagination
Here’s a code snippet illustrating pagination in a web application:
SELECT * FROM articles ORDER BY published_date DESC LIMIT 10 OFFSET 30; -- Fetch articles for page 4
Common Pitfalls and Solutions
Developers often face challenges when using the LIMIT clause in MySQL queries. Here are some common issues and their solutions:
Lack of ORDER BY
As mentioned earlier, using LIMIT without an ORDER BY can lead to inconsistent results. Always include an order clause to ensure predictable output.
Large OFFSET Values
As discussed, large OFFSET values can severely impact performance. Instead, using keyset pagination can help mitigate this issue.
Data Inconsistency
In distributed databases, data consistency can be a challenge. Consider implementing consistency checks or using more robust data retrieval strategies to ensure accuracy.
Reporting and Analytical Queries
Using LIMIT in reporting queries can sometimes lead to incomplete results. Ensure that your reporting logic accounts for LIMIT and provides a comprehensive view of the data.
Integrating Chat2DB for Enhanced LIMIT Management
Introducing Chat2DB, an AI database visualization management tool that revolutionizes how you manage MySQL queries, including those utilizing the LIMIT clause.
Chat2DB offers a range of features designed to simplify query crafting and enhance performance:
-
Intelligent Query Builder: Easily construct complex queries, including those with LIMIT and OFFSET clauses, using an intuitive interface.
-
Visual Tools: Gain insights into your query performance with visual representations, allowing you to identify bottlenecks quickly.
-
AI-Powered Suggestions: Leverage AI capabilities to receive recommendations on optimizing your queries, including the effective use of LIMIT.
-
Natural Language Processing: Write SQL queries in natural language, making it accessible for users less familiar with SQL syntax.
By integrating Chat2DB into your workflow, you can automate complex query tasks, streamline pagination, and ensure optimal performance across your database management processes. Unlike competitors such as DBeaver, MySQL Workbench, or DataGrip, Chat2DB provides unparalleled AI-powered functionalities that enhance user experience and efficiency.
Explore the capabilities of Chat2DB and see how it can transform your approach to working with the LIMIT clause and beyond. For more information, visit Chat2DB (opens in a new tab).
Exploring Alternatives to LIMIT
While the LIMIT clause is powerful, there are scenarios where alternatives may be more suitable. Here are a few options:
-
Window Functions: For complex analytics, consider using window functions, which can provide similar capabilities without relying solely on LIMIT.
-
Custom Stored Procedures: Implementing stored procedures can achieve similar results, particularly when you need more control over data retrieval.
-
Application-Level Pagination: In some cases, handling pagination at the application level may provide benefits over database-driven methods.
-
Data Warehousing Solutions: For handling massive datasets, consider data warehousing solutions that can manage data efficiently without the need for LIMIT.
-
Database Views: Using views can help pre-aggregate data, allowing for quicker retrieval without needing to apply LIMIT in every query.
By understanding these alternatives, you can choose the best strategy for your specific use case and ensure your database queries remain efficient and effective.
Conclusion
In this article, we have explored the various facets of the MySQL LIMIT clause, from its basic usage to advanced patterns and alternatives. By leveraging these techniques and tools like Chat2DB, you can significantly enhance your database management practices and ensure optimal performance.
FAQ
-
What is the purpose of the LIMIT clause in MySQL?
- The LIMIT clause controls the number of records returned by a query, which helps optimize data retrieval and manage resources.
-
How do I use LIMIT with OFFSET?
- You can use LIMIT with OFFSET to skip a specified number of rows before starting to return results, which is useful for pagination.
-
Can LIMIT be used without ORDER BY?
- While it can be used without ORDER BY, it is not recommended as it can lead to inconsistent and unpredictable results.
-
What are the performance implications of using LIMIT?
- Using LIMIT can enhance performance by reducing the load on the database server and memory usage, especially when working with large datasets.
-
How can Chat2DB help with MySQL LIMIT management?
- Chat2DB provides an intuitive interface, intelligent query building, and AI-powered suggestions to optimize the use of the LIMIT clause and improve overall database performance.
By following the insights shared in this article, you can enhance your understanding and application of the MySQL LIMIT clause, ultimately improving your database management efficiency.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!