How to Effectively Implement the IF Statement in MySQL Queries: A Step-by-Step Guide
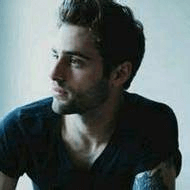
Understanding the IF Statement in MySQL
Before diving into the implementation of the IF statement in MySQL, it's crucial to understand its functionality and primary purpose. The IF statement is a fundamental control structure in MySQL that allows you to perform conditional operations within your SQL queries. The standard syntax for an IF statement follows the format: IF(condition, true_value, false_value)
. This structure enables you to execute different actions based on whether a specified condition evaluates to true or false.
The Role of the IF Statement
The IF statement allows for conditional data retrieval or manipulation. For example, if you want to display a message based on a user's score, you could use an IF statement to return "Pass" if the score is above 50 and "Fail" otherwise. This makes IF statements particularly useful for enhancing query efficiency and readability.
In database management, understanding the IF statement is essential for ensuring accurate data processing and decision-making within your queries. It’s also important to differentiate between the IF statement and other conditional statements like CASE (opens in a new tab) and IFNULL (opens in a new tab) in MySQL, as they serve different purposes and contexts.
Example Scenarios
Consider the scenario where you have a database of students and their scores. You might want to categorize their performance using an IF statement:
SELECT student_name,
score,
IF(score >= 50, 'Pass', 'Fail') AS result
FROM students;
This query returns each student's name, score, and whether they passed or failed based on their score. Using IF statements in this manner simplifies your queries while enhancing their clarity.
Setting Up Your Environment for MySQL Querying
To effectively implement IF statements, a well-configured MySQL environment is essential. Here, we’ll guide you through installing and setting up MySQL on common operating systems like Windows, macOS, and Linux.
Installation Steps
Operating System | Steps |
---|---|
Windows | 1. Download the MySQL installer from the official MySQL website (opens in a new tab). 2. Follow the installation prompts to install MySQL Server and MySQL Workbench. |
macOS | 1. Use Homebrew to install MySQL. 2. Start the MySQL server. |
Linux | 1. For Ubuntu, run the following commands: sudo apt update sudo apt install mysql-server 2. Secure the installation: sudo mysql_secure_installation |
Utilizing Chat2DB for Streamlined Management
To enhance your MySQL querying experience, consider using a robust database management tool like Chat2DB (opens in a new tab). Chat2DB is an AI-powered database visualization management tool that allows developers and database administrators to operate their databases more efficiently. It provides features such as natural language SQL generation, an intelligent SQL editor, and the ability to generate visual charts from data analysis.
Creating a Sample Database
Once MySQL is installed, create a sample database and populate it with data for testing your IF statement queries. Here’s how to create a database and a sample table:
CREATE DATABASE school;
USE school;
CREATE TABLE students (
student_id INT AUTO_INCREMENT PRIMARY KEY,
student_name VARCHAR(100),
score INT
);
INSERT INTO students (student_name, score) VALUES
('Alice', 85),
('Bob', 45),
('Charlie', 70);
Implementing IF Statements in MySQL Queries
Now that your environment is set up, let’s dive into practical steps for incorporating IF statements into MySQL queries.
Writing Basic IF Statement Queries
The simplest application of the IF statement is in SELECT queries. Here’s a basic example:
SELECT student_name,
score,
IF(score >= 50, 'Pass', 'Fail') AS result
FROM students;
This query uses the IF statement to evaluate whether each student has passed or failed.
Using IF Statements in INSERT, UPDATE, and DELETE Operations
IF statements can also be used in other SQL operations such as INSERT, UPDATE, and DELETE. For instance, you can utilize an IF statement in an UPDATE query to change the value of a column based on a condition:
UPDATE students
SET result = IF(score >= 50, 'Pass', 'Fail');
In this case, the result
column is updated to reflect whether students passed or failed based on their scores.
Nested IF Statements
You can also create nested IF statements for more complex conditions. For example:
SELECT student_name,
score,
IF(score >= 85, 'Excellent',
IF(score >= 50, 'Good', 'Needs Improvement')) AS performance
FROM students;
This query categorizes students into three performance levels based on their scores.
Common Pitfalls to Avoid
When using IF statements, it’s important to avoid common mistakes such as:
- Forgetting to include the necessary commas and parentheses.
- Using incorrect data types in conditions.
- Overcomplicating the logic, which may lead to unreadable queries.
Performance Considerations
For complex queries involving IF statements, consider performance implications. Optimizing your queries can significantly improve execution time, especially when working with large datasets.
Advanced Techniques and Best Practices
To elevate your use of IF statements, explore advanced techniques and best practices.
Integration with Stored Procedures
You can integrate IF statements with stored procedures for modular and reusable code. For example:
DELIMITER //
CREATE PROCEDURE CheckStudentPerformance(IN student_id INT)
BEGIN
DECLARE student_score INT;
SELECT score INTO student_score FROM students WHERE student_id = student_id;
SELECT IF(student_score >= 50, 'Pass', 'Fail') AS result;
END //
DELIMITER ;
Using IF Statements within Triggers
IF statements can automate database operations based on conditions by using triggers:
CREATE TRIGGER before_insert_students
BEFORE INSERT ON students
FOR EACH ROW
BEGIN
SET NEW.result = IF(NEW.score >= 50, 'Pass', 'Fail');
END;
Documentation and Code Comments
Maintaining clear documentation and code comments can help manage complex conditional logic effectively. This is especially important when collaborating with other developers or revisiting your code after some time.
Real-World Applications and Case Studies
To illustrate the practical applications of IF statements, consider various scenarios where they have been effectively utilized.
E-commerce Platforms
E-commerce platforms often use IF statements to manage inventory and pricing dynamically. For example, a query can automatically adjust prices based on stock levels:
UPDATE products
SET price = IF(stock < 10, price * 1.2, price)
WHERE product_id = 1;
Financial Institutions
Financial institutions leverage IF statements for personalized customer data processing, such as determining credit eligibility based on income levels.
Healthcare Systems
In healthcare, IF statements are used to manage patient data, such as categorizing patient risk levels based on various health metrics.
Business Intelligence
IF statements play a crucial role in optimizing business intelligence and reporting systems by allowing dynamic data analysis.
CRM Systems
Customer Relationship Management (CRM) systems utilize IF statements for targeted marketing and customer engagement, tailoring messages based on individual customer behavior.
Explore Chat2DB for Enhanced Database Management
As you continue to explore the capabilities of IF statements in MySQL, consider switching to Chat2DB (opens in a new tab) for a more streamlined database management experience. Its AI features allow for natural language SQL generation, intelligent suggestions, and visual data analysis tools, significantly enhancing your productivity and efficiency compared to other tools like DBeaver, MySQL Workbench, or DataGrip.
FAQ
-
What are IF statements in MySQL?
- IF statements are conditional expressions used in MySQL queries to return different values based on specified conditions.
-
How do I implement an IF statement in a SELECT query?
- You can use the syntax
IF(condition, true_value, false_value)
within a SELECT statement to return values based on conditions.
- You can use the syntax
-
Can I use IF statements in INSERT and UPDATE operations?
- Yes, IF statements can be utilized in INSERT and UPDATE operations to conditionally manipulate data.
-
What are the advantages of using Chat2DB?
- Chat2DB offers AI-powered features such as natural language SQL generation, an intelligent SQL editor, and visual data analysis tools, enhancing database management efficiency.
-
Are there any common mistakes to avoid when using IF statements?
- Common mistakes include incorrect syntax, using the wrong data types, and overcomplicating the logic, which can lead to confusion and errors in queries.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!