How to Do Full Join in MySQL
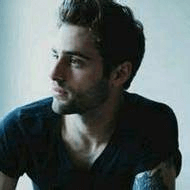
Understanding Joins in SQL
Before we dive into the intricacies of full joins in MySQL, it's essential to grasp the fundamental concepts of joins in SQL. Joins allow us to retrieve data from two or more tables based on related columns between them. The most common types of joins are INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN. Each join type serves a specific purpose and is used depending on the data retrieval requirements.
- INNER JOIN returns the rows with matching values in both tables.
- LEFT JOIN returns all rows from the left table and matched rows from the right table.
- RIGHT JOIN does the opposite, returning all rows from the right table and matched rows from the left.
The FULL JOIN, which is the focus of this tutorial, returns all rows from both tables, inserting NULLs where there is no match. Understanding these concepts is crucial for effectively working with databases and retrieving comprehensive datasets.
Introduction to Full Joins
A full join, or full outer join, combines the effects of both LEFT JOIN and RIGHT JOIN. It retrieves records when there is a match in either left or right table records. If there is no match, the result will contain NULL on the side that lacks a matching row.
Full joins are particularly beneficial when you need a complete dataset from two tables, including all unmatched rows from both sides. For instance, in data warehouse reporting, when merging datasets from different sources, full joins allow you to ensure that no data is lost in the process.
Executing Full Joins in MySQL
Unlike other SQL databases like PostgreSQL or SQL Server, MySQL does not directly support the FULL JOIN keyword. However, you can achieve the same result by combining LEFT JOIN and RIGHT JOIN using a UNION. This section provides a step-by-step guide on how to do a full join in MySQL.
Example Scenario
Let’s consider two sample tables, customers
and orders
.
CREATE TABLE customers (
customer_id INT PRIMARY KEY,
customer_name VARCHAR(100)
);
CREATE TABLE orders (
order_id INT PRIMARY KEY,
customer_id INT,
order_amount DECIMAL(10, 2)
);
Step 1: Populate Sample Data
First, we need to populate these tables with some sample data.
INSERT INTO customers (customer_id, customer_name) VALUES
(1, 'Alice'),
(2, 'Bob'),
(3, 'Charlie');
INSERT INTO orders (order_id, customer_id, order_amount) VALUES
(101, 1, 250.00),
(102, 2, 150.00),
(103, NULL, 300.00);
Step 2: Perform a LEFT JOIN
Now, let’s perform a LEFT JOIN to get all records from the customers
table and matched records from the orders
table.
SELECT c.customer_id, c.customer_name, o.order_id, o.order_amount
FROM customers c
LEFT JOIN orders o ON c.customer_id = o.customer_id;
This query will return:
customer_id | customer_name | order_id | order_amount |
---|---|---|---|
1 | Alice | 101 | 250.00 |
2 | Bob | 102 | 150.00 |
3 | Charlie | NULL | NULL |
Step 3: Perform a RIGHT JOIN
Next, we will execute a RIGHT JOIN to fetch all records from the orders
table and matching entries from the customers
table.
SELECT c.customer_id, c.customer_name, o.order_id, o.order_amount
FROM customers c
RIGHT JOIN orders o ON c.customer_id = o.customer_id;
This query will yield:
customer_id | customer_name | order_id | order_amount |
---|---|---|---|
1 | Alice | 101 | 250.00 |
2 | Bob | 102 | 150.00 |
NULL | NULL | 103 | 300.00 |
Step 4: Combine with UNION
Finally, we use a UNION to combine the results of the LEFT JOIN and RIGHT JOIN, effectively simulating a full join.
SELECT c.customer_id, c.customer_name, o.order_id, o.order_amount
FROM customers c
LEFT JOIN orders o ON c.customer_id = o.customer_id
UNION
SELECT c.customer_id, c.customer_name, o.order_id, o.order_amount
FROM customers c
RIGHT JOIN orders o ON c.customer_id = o.customer_id;
The result will be:
customer_id | customer_name | order_id | order_amount |
---|---|---|---|
1 | Alice | 101 | 250.00 |
2 | Bob | 102 | 150.00 |
3 | Charlie | NULL | NULL |
NULL | NULL | 103 | 300.00 |
Handling NULL Values in Full Joins
Full joins often result in NULL values in columns where there is no match between the tables. Handling these NULL values effectively is crucial for maintaining data integrity and accuracy in your results.
One common strategy for dealing with NULL values is using the COALESCE
function. This function returns the first non-null value in the list of arguments.
SELECT c.customer_id, c.customer_name,
COALESCE(o.order_id, 'No Order') AS order_id,
COALESCE(o.order_amount, 0) AS order_amount
FROM customers c
LEFT JOIN orders o ON c.customer_id = o.customer_id
UNION
SELECT c.customer_id, c.customer_name,
COALESCE(o.order_id, 'No Order') AS order_id,
COALESCE(o.order_amount, 0) AS order_amount
FROM customers c
RIGHT JOIN orders o ON c.customer_id = o.customer_id;
This query will replace NULL values with user-defined defaults, enhancing clarity in your results.
Performance Considerations for Full Joins
Execution of full joins can be resource-intensive, especially when dealing with large datasets. Factors such as indexing and query optimization play significant roles in improving performance.
Indexing
Creating indexes on the columns used in the join condition can significantly speed up the execution of join queries. For instance, adding an index on the customer_id
column in both tables can lead to faster query performance.
CREATE INDEX idx_customer_id ON customers(customer_id);
CREATE INDEX idx_order_customer_id ON orders(customer_id);
Query Optimization
Always assess whether a full join is necessary. In some cases, data denormalization or using other join types may provide better performance. Analyze your query execution plans to understand how your database engine processes the query.
Using Chat2DB for SQL Query Optimization
For those looking to enhance their SQL query performance, consider using Chat2DB. Chat2DB is an AI-powered database visualization management tool that assists in optimizing SQL queries, including full joins.
Key Features of Chat2DB
- Natural Language Query Generation: Convert your queries into natural language requests, making it easier to retrieve the data you need without deep SQL knowledge.
- Intelligent SQL Editor: Chat2DB provides an intelligent SQL editor that suggests optimizations and highlights potential issues in your queries.
- Data Visualization: Generate visual representations of your data effortlessly, allowing you to understand patterns and insights at a glance.
- AI-Powered Insights: Leverage AI capabilities to gain insights into your query performance and optimize your SQL statements effectively.
By utilizing Chat2DB, users can analyze SQL queries, suggest optimizations, and provide insights into query performance. Its AI capabilities make it a powerful ally in managing and optimizing full join operations in MySQL, surpassing other tools in efficiency and ease of use.
FAQ
1. What is a full join in SQL?
A full join returns all records from both tables involved in the join, with NULLs in places where there is no match.
2. How do I execute a full join in MySQL?
MySQL does not support the FULL JOIN keyword directly. You can simulate it by combining LEFT JOIN and RIGHT JOIN using a UNION.
3. Why are NULL values present in the result of a full join?
NULL values appear in the result when there is no matching record in one of the tables.
4. How can I handle NULL values in MySQL?
You can use the COALESCE function to replace NULL values with default values.
5. What are the advantages of using Chat2DB?
Chat2DB offers AI-powered features like natural language query generation, intelligent SQL editing, and data visualization, making database management more efficient and user-friendly. Transitioning to Chat2DB can significantly enhance your database management experience compared to traditional tools like DBeaver, MySQL Workbench, or DataGrip.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!