How to Effectively Use Inner Join in MySQL: A Step-by-Step Guide
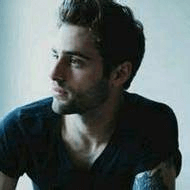
Understanding Inner Join in MySQL
An Inner Join in MySQL is a fundamental concept in relational database management that allows you to retrieve records from two or more tables based on a related column between them. The primary purpose of an Inner Join is to return data that has matching values in both tables. This is essential when you are working with structured data that is spread across multiple tables.
For instance, consider a database with a Customers
table and an Orders
table. If you want to fetch a list of customers along with their corresponding orders, you would use an Inner Join. This query effectively combines the data from both tables based on a common field, such as CustomerID
.
Here’s a simple SQL example of how an Inner Join works:
SELECT Customers.CustomerName, Orders.OrderID
FROM Customers
INNER JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
In this query, the INNER JOIN
statement combines records from the Customers
and Orders
tables where the CustomerID
matches in both, thereby displaying the customer's name alongside their order ID.
The Necessity of Inner Joins
Inner Joins are vital for many common scenarios in database management. For example, if you run a business and need to analyze sales data, you might want to join the Sales
table with the Products
table to identify which products sold best. Inner Joins facilitate this by allowing you to filter and retrieve data that meets specific relational criteria.
How Inner Join Works in MySQL
To understand how Inner Joins function in MySQL, it is important to grasp the syntax and structure of an Inner Join query. The basic structure consists of the SELECT
statement, the FROM
clause where the primary table is specified, followed by the INNER JOIN
clause that indicates the second table to join and the ON
clause that specifies the condition for the join.
Here’s a breakdown of the syntax:
SELECT column1, column2
FROM table1
INNER JOIN table2 ON table1.common_column = table2.common_column;
Example of an Inner Join Query
Let’s take a look at a more detailed example:
SELECT Orders.OrderID, Customers.CustomerName
FROM Orders
INNER JOIN Customers ON Orders.CustomerID = Customers.CustomerID;
In this case, the query returns the order ID from the Orders
table and the customer name from the Customers
table, where the CustomerID
is the linking factor.
The ON
clause is crucial as it defines the condition that must be met for the records to be included in the result set. Without it, the Inner Join would not know how to associate the two tables.
Common Use Cases for Inner Join
Inner Joins are indispensable in various practical scenarios. Here are some common use cases:
Use Case | SQL Example |
---|---|
Combining Employee and Department Data | sql SELECT Employees.EmployeeName, Departments.DepartmentName FROM Employees INNER JOIN Departments ON Employees.DepartmentID = Departments.DepartmentID; |
Analyzing Sales Performance by Product | sql SELECT Products.ProductName, SUM(Sales.Quantity) AS TotalSold FROM Sales INNER JOIN Products ON Sales.ProductID = Products.ProductID GROUP BY Products.ProductName; |
These examples illustrate the efficiency of Inner Joins in filtering and retrieving relevant data that meets specific relational criteria.
Step-by-Step Guide to Implementing Inner Join
Implementing an Inner Join in MySQL involves several steps. Below is a detailed walkthrough:
Step 1: Setting Up Sample Tables
Assuming you have two tables, Customers
and Orders
, set them up as follows:
CREATE TABLE Customers (
CustomerID INT PRIMARY KEY,
CustomerName VARCHAR(255)
);
CREATE TABLE Orders (
OrderID INT PRIMARY KEY,
CustomerID INT,
OrderDate DATE,
FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID)
);
Step 2: Populating the Tables
Next, insert some sample data into both tables:
INSERT INTO Customers (CustomerID, CustomerName) VALUES
(1, 'John Doe'),
(2, 'Jane Smith'),
(3, 'Alice Johnson');
INSERT INTO Orders (OrderID, CustomerID, OrderDate) VALUES
(101, 1, '2023-01-01'),
(102, 1, '2023-01-02'),
(103, 2, '2023-01-03');
Step 3: Writing the Inner Join Query
Now that the data is set up, you can write an Inner Join query to retrieve the desired information:
SELECT Customers.CustomerName, Orders.OrderID, Orders.OrderDate
FROM Customers
INNER JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
Step 4: Executing the Query
Run the query in your MySQL environment, and you will see results that include the customers' names alongside their respective order IDs and order dates.
Step 5: Interpreting the Results
The result set will show only those customers who have placed orders, reflecting the relationship established between the two tables through the Inner Join.
Optimizing Inner Join Queries
While Inner Joins are effective, optimizing these queries can significantly improve performance. Here are some strategies:
Indexing Best Practices
Ensure that the columns used in the join condition are indexed. For example:
CREATE INDEX idx_customer_id ON Orders(CustomerID);
This index will speed up the join operation by allowing MySQL to quickly locate the relevant records.
Analyzing Query Performance
Utilize MySQL’s EXPLAIN
statement to analyze query performance. By prefixing your query with EXPLAIN
, you can gain insights into how MySQL executes the join and identify potential bottlenecks.
EXPLAIN SELECT Customers.CustomerName, Orders.OrderID
FROM Customers
INNER JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
Restructuring Queries
Sometimes, restructuring queries can help reduce complexity and improve execution speed. For instance, avoid using SELECT * and specify only the columns you need.
Advanced Techniques with Inner Join
Joining Multiple Tables
Inner Joins can be extended to combine data from multiple tables. For example, if you also have a Products
table, you can join all three tables to retrieve comprehensive data:
SELECT Customers.CustomerName, Orders.OrderID, Products.ProductName
FROM Customers
INNER JOIN Orders ON Customers.CustomerID = Orders.CustomerID
INNER JOIN Products ON Orders.ProductID = Products.ProductID;
Self-Joins
A self-join is a special case where a table is joined with itself. This can be useful for hierarchical data. For example, if you have an Employees
table with a ManagerID
, you can find the managers of each employee:
SELECT A.EmployeeName AS Employee, B.EmployeeName AS Manager
FROM Employees A
INNER JOIN Employees B ON A.ManagerID = B.EmployeeID;
Using Aliases
To simplify complex queries, you can use aliases. This enhances readability and makes your SQL statements cleaner. For instance:
SELECT C.CustomerName, O.OrderID
FROM Customers AS C
INNER JOIN Orders AS O ON C.CustomerID = O.CustomerID;
Leveraging Chat2DB for Managing Joins
Managing Inner Joins in MySQL can be made significantly easier with tools like Chat2DB (opens in a new tab). Chat2DB is an AI-driven database visualization management tool designed to enhance database management efficiency and intelligence.
Its intuitive user interface allows developers and database administrators to construct queries with ease. The real-time feedback feature helps you understand how your queries perform, while the visual representation of database schemas simplifies the join process.
One of the standout features of Chat2DB is its AI capabilities, which include natural language processing to generate SQL queries automatically. This functionality allows users to interact with their databases using plain language, making it an excellent tool for both novice and experienced database users.
With Chat2DB, you can manage complex joins, and overall productivity is enhanced, making it a superior choice compared to traditional tools like DBeaver, MySQL Workbench, or DataGrip.
Conclusion
To summarize, mastering Inner Joins in MySQL is crucial for anyone working with relational databases. By understanding how Inner Joins work, recognizing their use cases, and utilizing advanced techniques, you can greatly enhance your database querying capabilities. Tools like Chat2DB (opens in a new tab) further empower users to manage their databases more effectively, leveraging AI to streamline operations and improve productivity.
FAQ
-
What is an Inner Join in MySQL?
- An Inner Join is a type of join that returns records with matching values in both tables based on a specified condition.
-
How do I optimize my Inner Join queries?
- You can optimize Inner Join queries by indexing the columns used in join conditions, analyzing performance with the EXPLAIN statement, and restructuring queries for efficiency.
-
Can I join more than two tables using Inner Joins?
- Yes, you can join multiple tables using Inner Joins by chaining additional INNER JOIN statements.
-
What is a self-join?
- A self-join is a join where a table is joined with itself, useful for querying hierarchical data.
-
How can Chat2DB help with Inner Joins?
- Chat2DB offers an intuitive interface for managing Inner Joins, real-time feedback, and AI capabilities for generating SQL queries, making database management more efficient.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!