How to Effectively Implement PostgreSQL Triggers: A Comprehensive Guide
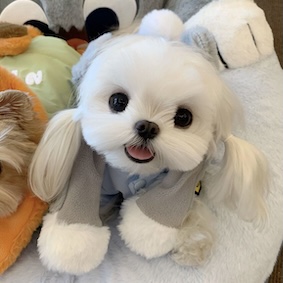
Triggers in PostgreSQL provide a robust mechanism for automating tasks and enforcing business rules within your database. This comprehensive guide will cover everything from the fundamental concepts of PostgreSQL triggers to advanced techniques and best practices. We'll explore how to set up your development environment, create and manage triggers, and debug them effectively. Additionally, we'll examine how tools like Chat2DB (opens in a new tab), an AI-driven database management solution, can significantly enhance your experience with PostgreSQL triggers. This guide is designed for developers and database administrators aiming to boost efficiency and ensure data integrity through effective trigger implementation.
Understanding PostgreSQL Triggers
PostgreSQL triggers are special user-defined functions that execute automatically in response to specific events on a particular table or view. Understanding key terms associated with triggers, such as event and condition, is crucial for effective implementation. In PostgreSQL, triggers can be categorized into several types:
Trigger Type | Description |
---|---|
BEFORE Triggers | Execute before the specified event. |
AFTER Triggers | Execute after the specified event. |
INSTEAD OF Triggers | Used primarily for views; allows executing an alternative action instead of the triggering event. |
Each type of trigger serves a distinct purpose, enabling developers to enforce business rules, maintain data integrity, and automate tasks efficiently. Additionally, triggers can be classified into row-level and statement-level triggers, where row-level triggers execute for each row affected by the triggering event, while statement-level triggers execute once for the entire statement.
Understanding the firing order of triggers is essential when multiple triggers are defined on a single table. The order in which triggers are activated can significantly impact the outcome of your database operations, making it vital to plan accordingly.
Setting Up Your Development Environment for PostgreSQL Triggers
Establishing a robust development environment is key when working with PostgreSQL triggers. Here's a step-by-step guide to setting up your environment:
- Install PostgreSQL: Download the latest version of PostgreSQL from the official website (opens in a new tab). Follow the installation instructions for your operating system.
- Choose a Database Management Tool: Consider using Chat2DB (opens in a new tab) for its AI-driven features that enhance database management. Chat2DB supports natural language SQL generation and offers intelligent SQL editing capabilities.
- Initial Configuration: After installation, configure your PostgreSQL server. Set up user roles, permissions, and any necessary extensions that may be required for your project.
- Version Control: Use version control systems like Git to manage changes in your database schema and trigger scripts effectively.
- Backup Strategy: Implement a backup strategy to protect your data. Regularly back up your database to prevent data loss due to unforeseen events.
Using Chat2DB, you can easily manage your PostgreSQL environment, allowing for efficient testing and implementation of triggers.
Creating Your First PostgreSQL Trigger
Creating a trigger in PostgreSQL involves defining a trigger function and binding it to a specific event. Here's a detailed walkthrough of the process:
- Define the Trigger Function: A trigger function must be created first. It contains the logic that will be executed when the trigger is fired. Below is an example of a trigger function that logs changes to a table:
CREATE OR REPLACE FUNCTION log_changes()
RETURNS TRIGGER AS $$
BEGIN
INSERT INTO change_log (table_name, operation, changed_at)
VALUES (TG_TABLE_NAME, TG_OP, NOW());
RETURN NEW;
END;
$$ LANGUAGE plpgsql;
- Create the Trigger: After defining the function, you can create the trigger that invokes this function. The following SQL statement creates a trigger that logs changes on the
employees
table:
CREATE TRIGGER employees_changes
AFTER INSERT OR UPDATE OR DELETE ON employees
FOR EACH ROW EXECUTE FUNCTION log_changes();
In this example, the trigger employees_changes
is activated after any INSERT
, UPDATE
, or DELETE
operation on the employees
table, logging the change in the change_log
table.
Common Use Cases for Triggers
Triggers are commonly used for various scenarios, including:
- Enforcing Business Rules: Ensure that certain conditions are met before allowing data changes.
- Auditing Changes: Keep track of modifications made to critical tables for compliance and analysis.
- Automatic Data Modification: Automatically modify related data in other tables when a specified event occurs.
Potential Pitfalls
When creating triggers, developers may encounter common errors, such as:
- Incorrect Logic Execution: Ensure that the logic within the trigger function is correct to avoid unexpected results.
- Performance Issues: Inefficient trigger functions can lead to performance degradation. Keep logic simple and avoid extensive computations within triggers.
Best Practices for Implementing Triggers
To ensure efficient and effective implementation of PostgreSQL triggers, consider the following best practices:
- Keep Trigger Functions Simple: Limit the logic within your trigger functions to essential operations. Avoid complex calculations or extensive data manipulations.
- Monitor Performance: Regularly assess the performance impact of triggers on your database operations. Use
EXPLAIN ANALYZE
to analyze query plans and identify bottlenecks. - Implement Error Handling: Use proper error handling within your triggers to maintain data integrity and avoid transaction rollbacks due to unexpected errors.
- Thorough Testing: Before deploying triggers in a production environment, thoroughly test them in a staging environment to ensure they function as intended.
- Regular Audits: Conduct regular audits of your triggers to verify their performance and relevance as your database requirements evolve.
Advanced Trigger Techniques
As you become more comfortable with PostgreSQL triggers, you may want to explore advanced techniques to optimize their usage:
Conditional Logic within Triggers
Incorporate conditional logic to refine trigger execution. For example, you can check specific conditions before executing certain actions within your trigger functions:
CREATE OR REPLACE FUNCTION conditional_trigger()
RETURNS TRIGGER AS $$
BEGIN
IF NEW.salary > 100000 THEN
INSERT INTO high_salary_log (employee_id, salary, logged_at)
VALUES (NEW.id, NEW.salary, NOW());
END IF;
RETURN NEW;
END;
$$ LANGUAGE plpgsql;
Dynamic Triggers
Consider implementing dynamic triggers that can adapt to changing data structures. This allows your triggers to remain relevant as your application evolves.
Integration with Other PostgreSQL Features
Leverage other PostgreSQL features, such as stored procedures and functions, to enhance the functionality of your triggers. This can lead to more complex and powerful data management solutions.
Debugging and Troubleshooting Triggers
Debugging triggers can be challenging. Here are some strategies to help you identify and resolve issues:
- Use PostgreSQL Logs: Analyze PostgreSQL logs to diagnose unexpected behavior or errors related to trigger execution.
- RAISE NOTICE: Utilize the
RAISE NOTICE
command to log intermediate states within your trigger functions for easier debugging. - Isolation of Issues: Isolate trigger issues from other database operations by temporarily disabling triggers or using test data.
- Avoid Deadlocks: Implement strategies to prevent deadlocks that may arise from complex trigger logic.
Regular audits of your triggers can help ensure their ongoing reliability and performance.
Case Study: Implementing Triggers with Chat2DB
To illustrate the practical application of PostgreSQL triggers, let’s consider a case study where we implement triggers to automate a complex data management process using Chat2DB (opens in a new tab).
Scenario Overview
In this scenario, we manage a customer orders database where we want to automatically log changes in order status. Using triggers, we can create an efficient auditing mechanism that logs every status change without manual intervention.
Step-by-Step Implementation
- Define the Trigger Function: We begin by defining a function that logs the status changes:
CREATE OR REPLACE FUNCTION log_order_status_changes()
RETURNS TRIGGER AS $$
BEGIN
INSERT INTO order_status_log (order_id, old_status, new_status, change_time)
VALUES (OLD.id, OLD.status, NEW.status, NOW());
RETURN NEW;
END;
$$ LANGUAGE plpgsql;
- Create the Trigger: Next, we create the trigger that invokes this function whenever an order status is updated:
CREATE TRIGGER order_status_change
AFTER UPDATE OF status ON orders
FOR EACH ROW WHEN (OLD.status IS DISTINCT FROM NEW.status)
EXECUTE FUNCTION log_order_status_changes();
Challenges and Outcomes
During implementation, we faced challenges related to performance as the volume of updates increased. By optimizing our trigger function to focus solely on relevant changes and utilizing the capabilities of Chat2DB for visualization and management, we successfully managed these challenges.
The outcome was a streamlined logging process that improved data consistency and reduced manual intervention significantly.
FAQs
1. What are triggers in PostgreSQL?
Triggers are user-defined functions that automatically execute in response to specific events on a database table.
2. How do I create a trigger in PostgreSQL?
To create a trigger, you first define a trigger function and then bind it to an event using the CREATE TRIGGER
statement.
3. Can triggers affect database performance?
Yes, poorly designed triggers can lead to performance bottlenecks. It's essential to keep trigger logic simple and efficient.
4. What is the difference between row-level and statement-level triggers?
Row-level triggers execute for each row affected by the triggering event, while statement-level triggers execute once for the entire statement.
5. How can Chat2DB help with PostgreSQL triggers?
Chat2DB enhances the management of PostgreSQL triggers with its AI-driven features, simplifying SQL generation and providing intelligent editing capabilities, making it easier to implement and debug triggers.
By leveraging the capabilities of Chat2DB, developers can further streamline their PostgreSQL database management processes, ensuring efficient implementation and maintenance of triggers. For an optimal database management experience, consider switching to Chat2DB today!
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!