How to Seamlessly Integrate Django with PostgreSQL for Scalable Applications
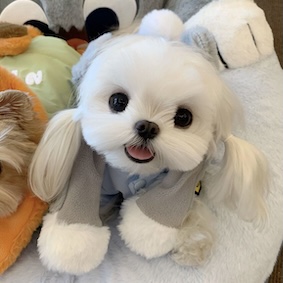
Integrating Django with PostgreSQL empowers developers to build robust, scalable applications. This article explores the core concepts of Django and PostgreSQL, guiding you through their setup, performance optimization, advanced features, and best practices in security. We’ll highlight the benefits of using Django's ORM with PostgreSQL, particularly its support for complex queries and large datasets. Additionally, we will showcase the AI-powered database management tool, Chat2DB (opens in a new tab), which significantly enhances the efficiency of managing PostgreSQL databases within Django applications.
Understanding Django and PostgreSQL
Overview of Django Framework
Django (opens in a new tab) is a high-level Python web framework that promotes rapid development and clean, pragmatic design. It offers a plethora of features that simplify web development, including an integrated admin panel, authentication system, and a powerful ORM (Object-Relational Mapping) for seamless database interactions.
Overview of PostgreSQL Database System
PostgreSQL (opens in a new tab) is a powerful, open-source object-relational database system recognized for its robustness, scalability, and compliance with SQL standards. It supports advanced data types, extensive indexing options, and complex queries, making it an ideal choice for data-intensive applications.
Advantages of Integrating Django with PostgreSQL
Integrating Django with PostgreSQL provides numerous benefits, including:
Benefit | Description |
---|---|
Seamless ORM Integration | Django's ORM works exceptionally well with PostgreSQL, enabling data manipulation without writing raw SQL. |
Support for Advanced Data Types | PostgreSQL's support for JSONB and array fields enhances Django's capabilities for flexible data models. |
Scalability | PostgreSQL's scalability features complement Django's ability to handle high-performance applications, making it suitable for growing data needs. |
Setting Up Django with PostgreSQL
Step 1: Install Required Packages
To start, install the necessary packages, particularly psycopg2
, the PostgreSQL adapter for Python. Use the following command:
pip install psycopg2
Step 2: Create a PostgreSQL Database
Create a new PostgreSQL database using the command line or a GUI tool like pgAdmin (opens in a new tab). Here’s how to do this via the command line:
CREATE DATABASE myprojectdb;
Step 3: Configure Django Settings
Next, configure settings.py
in your Django project to connect to the PostgreSQL database. Add the following configuration to the DATABASES
section:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.postgresql',
'NAME': 'myprojectdb',
'USER': 'your_username',
'PASSWORD': 'your_password',
'HOST': 'localhost',
'PORT': '5432',
}
}
For enhanced security, consider using environment variables for sensitive data:
import os
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.postgresql',
'NAME': os.environ.get('DB_NAME'),
'USER': os.environ.get('DB_USER'),
'PASSWORD': os.environ.get('DB_PASSWORD'),
'HOST': os.environ.get('DB_HOST', 'localhost'),
'PORT': os.environ.get('DB_PORT', '5432'),
}
}
Step 4: Run Initial Migrations
Once the database is configured, run the initial migrations to set up Django's default tables in PostgreSQL:
python manage.py migrate
Step 5: Test the Connection
You can test the connection by running the development server:
python manage.py runserver
If the server starts without errors, the setup is successful.
Optimizing Django Applications with PostgreSQL Features
Full-Text Search Capabilities
Leverage PostgreSQL's robust full-text search capabilities in Django applications. Here's how to create a model that utilizes full-text search:
from django.db import models
from django.contrib.postgres.search import SearchVector
class Article(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
class Meta:
indexes = [
models.Index(fields=['title']),
]
# Querying with full-text search
from django.contrib.postgres.search import SearchQuery
query = SearchQuery('Django')
results = Article.objects.annotate(search=SearchVector('title', 'content')).filter(search=query)
JSONB Fields for Semi-Structured Data
Django models can use PostgreSQL's JSONB fields for storing semi-structured data. Here’s how to define a model with a JSONB field:
from django.contrib.postgres.fields import JSONField
class Product(models.Model):
name = models.CharField(max_length=100)
attributes = JSONField()
# Example usage
product = Product(name='Laptop', attributes={'color': 'black', 'RAM': '16GB'})
product.save()
Array Fields in Django Models
Utilize PostgreSQL's array fields in Django models to efficiently store lists of data:
from django.contrib.postgres.fields import ArrayField
class UserProfile(models.Model):
username = models.CharField(max_length=100)
favorite_colors = ArrayField(models.CharField(max_length=50), blank=True)
# Example usage
user_profile = UserProfile(username='john_doe', favorite_colors=['blue', 'green'])
user_profile.save()
Indexing Strategies
To improve query performance, consider implementing indexing strategies. PostgreSQL supports B-tree and GIN indexes that can significantly enhance data retrieval speed. Here’s how to create a GIN index for a JSONB field:
CREATE INDEX idx_gin_attributes ON myapp_product USING GIN (attributes);
Database Tuning and Configuration
Optimizing PostgreSQL for your Django applications may involve adjusting configuration parameters. Key settings include:
shared_buffers
: Typically set to 15-25% of your system memory.work_mem
: Increased for complex queries to allow more memory for sorting and joining.maintenance_work_mem
: Higher values for maintenance tasks like vacuuming.
Implementing Django ORM with PostgreSQL
Understanding Django ORM
Django's ORM abstracts database interactions, enabling developers to define models that map directly to PostgreSQL tables. Here’s an example:
class BlogPost(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
created_at = models.DateTimeField(auto_now_add=True)
# Creating a new blog post
new_post = BlogPost(title='My first post', content='This is the content of my first post.')
new_post.save()
Using QuerySet API
Django's QuerySet API provides a powerful method to construct complex database queries. Here’s how to filter and order results:
# Filtering posts created after a specific date
from datetime import datetime
recent_posts = BlogPost.objects.filter(created_at__gt=datetime(2023, 1, 1)).order_by('-created_at')
Database Migrations
Django’s migration system allows you to efficiently manage schema changes in your PostgreSQL database. Create a new migration with:
python manage.py makemigrations
And apply it with:
python manage.py migrate
Performance Optimization Techniques
To further enhance performance, consider using select_related
and prefetch_related
to minimize database hits:
# Using select_related for foreign key relationships
posts_with_authors = BlogPost.objects.select_related('author').all()
# Using prefetch_related for many-to-many relationships
posts_with_tags = BlogPost.objects.prefetch_related('tags').all()
Scaling Django Applications with PostgreSQL
Importance of Database Scaling
As the load and traffic increase on your Django applications, database scaling becomes crucial. PostgreSQL offers various scaling strategies, including vertical scaling (upgrading hardware) and horizontal scaling (replication and sharding).
Connection Pooling
Implementing connection pooling can help manage multiple database connections efficiently, reducing overhead. Python libraries like psycopg2
facilitate connection pooling.
Replication Features
PostgreSQL's built-in replication allows you to create read replicas to distribute read traffic effectively. Here’s a simple setup for streaming replication:
- Configure the primary server to allow replication.
- Set up the standby server to connect to the primary.
Caching Strategies
Implementing caching strategies within Django can reduce load on the database and improve response times. Using tools like Redis or Memcached can store frequently accessed data.
Monitoring Tools
Utilizing monitoring tools, including Chat2DB (opens in a new tab), enables you to track performance metrics and identify bottlenecks in your PostgreSQL databases. Chat2DB's AI features facilitate the visualization of database performance and the optimization of queries effortlessly.
Security Best Practices for Django and PostgreSQL
Securing Database Credentials
Securing your database credentials is essential. Always use environment variables to store sensitive data and consider secret management tools for added security.
Configuring SSL Connections
Ensure that PostgreSQL is configured to use SSL connections, encrypting data in transit to protect against eavesdropping.
Implementing Django Security Features
Django provides built-in security features, such as CSRF protection and input validation, which help prevent SQL injection and other attacks.
Setting Up Roles and Permissions
Configure PostgreSQL roles and permissions to enforce least privilege access, ensuring that users have only the access they need.
Regular Audits and Logging
Conduct regular database audits and enable logging to identify suspicious activities. Keeping logs helps in monitoring access and potential security breaches.
Utilizing Chat2DB for Security Monitoring
Chat2DB (opens in a new tab) aids in monitoring security aspects of your PostgreSQL databases, providing insights into user access patterns and potential vulnerabilities.
FAQs
-
What is Django?
- Django is a high-level Python web framework designed for rapid development and clean design.
-
Why use PostgreSQL with Django?
- PostgreSQL's advanced features and scalability complement Django's capabilities, making it ideal for high-performance applications.
-
How do I set up Django with PostgreSQL?
- Install
psycopg2
, create a PostgreSQL database, and configuresettings.py
in your Django project to connect to the database.
- Install
-
What are some performance optimization techniques for Django and PostgreSQL?
- Techniques include advanced indexing, optimizing queries with
select_related
andprefetch_related
, and implementing caching strategies.
- Techniques include advanced indexing, optimizing queries with
-
How can Chat2DB help with database management?
- Chat2DB provides AI-powered tools for visualizing and managing PostgreSQL databases, enhancing efficiency and simplifying complex queries.
By integrating Django with PostgreSQL, developers can harness the power of both technologies to create scalable applications. Emphasizing the role of tools like Chat2DB (opens in a new tab) can further enhance database management and performance, providing an edge over other competing tools. Switch to Chat2DB for an AI-driven database management experience that simplifies your workflow and amplifies productivity.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!