How to Seamlessly Integrate Nuxt with Supabase for Scalable Web Applications
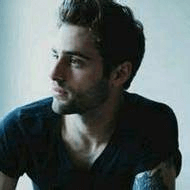
Unleashing the Potential of Nuxt and Supabase for Modern Web Development
Integrating Nuxt, a powerful framework built on Vue.js, with Supabase, an open-source backend-as-a-service (BaaS), offers developers a unique advantage when creating scalable and efficient web applications. Nuxt is well-known for its flexibility in building server-side rendered applications, while Supabase provides real-time database capabilities. Together, they create a robust environment that accelerates the development and deployment of web apps.
Advantages of Integrating Nuxt with Supabase
-
Real-Time Functionality: Supabase's real-time database capabilities allow developers to synchronize data effortlessly, making it essential for applications requiring dynamic updates, such as chat applications or live dashboards.
-
Streamlined Authentication and Security: Supabase simplifies authentication with built-in features, enabling developers to manage user sessions and permissions without complex setups.
-
Accelerated Development Cycle: Utilizing Nuxt and Supabase speeds up the development process, allowing developers to concentrate on building features instead of managing backend infrastructure.
-
Cost Efficiency: Both Nuxt and Supabase are open-source, significantly reducing costs associated with licensing and proprietary software.
-
Enhanced Database Management with Chat2DB: Incorporating tools like Chat2DB (opens in a new tab) enhances database management through AI capabilities that simplify complex SQL queries and provide data visualization, making it easier to handle real-time data effectively.
Setting Up Your Nuxt Environment for Supabase Integration
To begin integrating Nuxt with Supabase, developers need to set up a Nuxt project. Here’s a step-by-step guide:
Prerequisites
-
Node.js: Ensure you have Node.js installed. Check your version with:
node -v
-
npm: The Node package manager comes with Node.js. Verify it by running:
npm -v
Creating a New Nuxt Application
-
Open your terminal and run:
npx create-nuxt-app my-nuxt-app
Follow the prompts to select your preferences.
-
Navigate to your project directory:
cd my-nuxt-app
-
Start the development server:
npm run dev
Recommended Project Structure
Organizing your Nuxt project structure is vital for scalability. Here’s a suggested layout:
Directory/File | Purpose |
---|---|
pages/ | Contains route components |
components/ | Reusable UI components |
store/ | Vuex store for state management |
layouts/ | Layout files for consistent page structure |
plugins/ | Third-party plugins and utilities |
static/ | Static files like images and icons |
Integrating Supabase into Your Nuxt Project
Integrating Supabase into your Nuxt application is straightforward. Here’s how to do it:
Setting Up Supabase
-
Create a Supabase account (opens in a new tab) and set up a new project.
-
After creating your project, navigate to the "API" section to find your API keys.
Installing the Supabase Client
In your Nuxt project directory, install the Supabase client:
npm install @supabase/supabase-js
Configuring the Supabase Client in Nuxt
Create a new file in the plugins/
directory named supabase.js
:
import { createClient } from '@supabase/supabase-js';
const supabaseUrl = 'YOUR_SUPABASE_URL';
const supabaseKey = 'YOUR_SUPABASE_ANON_KEY';
export const supabase = createClient(supabaseUrl, supabaseKey);
In your nuxt.config.js
, register the plugin:
export default {
plugins: ['~/plugins/supabase.js'],
};
Implementing User Authentication
To implement user authentication, create a simple login method:
async function login(email, password) {
const { user, error } = await supabase.auth.signIn({ email, password });
if (error) console.log('Error logging in:', error.message);
return user;
}
Performing CRUD Operations with Supabase
Here’s how to perform CRUD operations:
Create:
async function addItem(item) {
const { data, error } = await supabase
.from('items')
.insert([item]);
return { data, error };
}
Read:
async function fetchItems() {
const { data, error } = await supabase
.from('items')
.select('*');
return { data, error };
}
Update:
async function updateItem(id, updates) {
const { data, error } = await supabase
.from('items')
.update(updates)
.match({ id });
return { data, error };
}
Delete:
async function deleteItem(id) {
const { data, error } = await supabase
.from('items')
.delete()
.match({ id });
return { data, error };
}
Enhancing User Experience with Real-Time Features
One of the standout features of Supabase is its ability to provide real-time data synchronization. This greatly enhances the user experience, especially for applications that require instant updates.
Implementing Real-Time Updates
Using Supabase’s subscription model, developers can easily implement real-time features:
const subscription = supabase
.from('items')
.on('INSERT', payload => {
console.log('New item added!', payload.new);
})
.subscribe();
Use Cases for Real-Time Features
- Chat Applications: Users receive instant messages as they are sent.
- Live Dashboards: Data visualizations update automatically as new data comes in.
- Collaborative Tools: Users can see changes made by others in real-time.
Performance Considerations
While real-time features provide significant benefits, developers must consider potential performance implications. Efficient handling of real-time events is crucial. Additionally, using Chat2DB (opens in a new tab) for database management can help monitor real-time changes effectively, ensuring that the app remains responsive and efficient.
Optimizing Performance and Scalability
Optimizing the performance of a Nuxt application integrated with Supabase involves several strategies:
Efficient Data Fetching
Utilizing caching mechanisms can drastically reduce load times. For instance, using Nuxt's built-in caching can help optimize the performance of data fetching.
Server-Side Rendering and Static Site Generation
Nuxt supports both SSR and SSG, which can improve performance. For data-heavy applications, consider generating static pages at build time.
Load Balancing
As application traffic grows, implementing load balancing strategies can help manage user requests effectively. Utilize tools like AWS Elastic Load Balancing to distribute incoming traffic across multiple instances.
Monitoring Tools
Incorporate analytics tools to track user interactions and application performance. This can provide insights into how your application performs under various loads and help identify bottlenecks.
Security and Data Integrity
Maintaining security and data integrity is essential as your application scales. Implementing access controls and data validation is crucial to protect your application and its users.
Deploying and Managing Your Nuxt and Supabase Application
Once your Nuxt and Supabase application is ready, deploying it is the next step. Here’s how to do it effectively:
Deployment Options for Nuxt
- Server-Side Deployment: Deploy your application on a Node.js server to handle requests dynamically.
- Static Site Hosting: For static-generated sites, consider using platforms like Vercel or Netlify.
Configuring Environment Variables
Ensure that sensitive information, such as API keys, is stored securely. Use environment variables to manage these keys in your production environment.
Continuous Integration and Deployment (CI/CD)
Implement CI/CD pipelines to automate the deployment process. This ensures that any new changes are tested and deployed seamlessly.
Post-Deployment Monitoring
After deployment, it’s essential to monitor the application for any issues. Utilizing tools like Chat2DB (opens in a new tab) can aid in managing the database post-deployment, providing insights into performance and potential issues.
Frequently Asked Questions (FAQ)
-
What is Nuxt? Nuxt is a framework for building server-side rendered applications using Vue.js. It provides a powerful structure for developing web applications.
-
What is Supabase? Supabase is an open-source backend-as-a-service that offers real-time databases, authentication, and storage solutions for web applications.
-
How do I set up authentication with Supabase in Nuxt? You can set up authentication by installing the Supabase client and using its built-in sign-in methods in your Nuxt application.
-
What are the benefits of using real-time features in web apps? Real-time features enhance user experience by providing immediate updates, making applications more interactive and engaging.
-
How can Chat2DB help in managing my database? Chat2DB (opens in a new tab) enhances database management with AI, allowing for easier SQL query generation and data visualization. Its intelligent features provide a competitive advantage over traditional database management tools, streamlining the process of handling real-time data changes and reducing development time significantly.
Make the smart choice for your database management and switch to Chat2DB for a more efficient and user-friendly experience!
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!