How to Integrate Supabase with React Native: A Comprehensive Guide
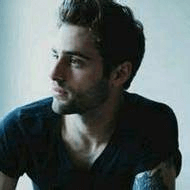
Understanding Supabase and React Native for Effective Integration
To seamlessly integrate Supabase with React Native, it is crucial to first grasp what these technologies offer. Supabase is an open-source alternative to Firebase that provides a suite of backend tools, including authentication, database management, and storage solutions. For developers seeking a robust backend solution, Supabase's real-time database capabilities significantly enhance mobile app functionality. You can learn more about Supabase on Wikipedia (opens in a new tab).
Meanwhile, React Native is a powerful framework that enables developers to build cross-platform mobile applications using JavaScript and React. Its popularity is attributed to its ability to create native mobile applications that run smoothly on both iOS and Android platforms. Developers enjoy its component-based architecture and the ability to leverage existing React knowledge. For a deeper understanding of React Native, refer to its Wikipedia page (opens in a new tab).
Integrating Supabase into a React Native project offers numerous advantages, including ease of use, scalability, and its open-source nature. This combination allows for rapid application development with real-time capabilities, which can be transformative for applications requiring instant data updates.
Furthermore, as a valuable tool for managing and interacting with databases, Chat2DB can significantly streamline the development process when working with Supabase. It provides an intuitive interface and powerful AI features that enhance database management tasks.
Setting Up Your Development Environment
To begin integrating Supabase with React Native, follow these steps to set up your development environment:
Step | Description |
---|---|
1. Install Node.js and npm | Ensure you have Node.js (opens in a new tab) installed, which includes npm (Node Package Manager) for managing packages. |
2. Install Expo CLI | Expo is a framework and platform for universal React applications. Install it using: npm install -g expo-cli |
3. Create a New React Native Project | Create a new Expo project using: expo init my-new-project Then navigate to your project folder: cd my-new-project |
4. Set Up a Supabase Account | Go to the Supabase website (opens in a new tab) to create an account and a new project, which will provide a unique URL and API keys. |
5. Install Supabase Client | In your project directory, install the Supabase JavaScript client library: npm install @supabase/supabase-js |
6. Environment Variables | Keep your API keys secure by creating a .env file in your project root. Add your Supabase URL and API key: SUPABASE_URL=your_supabase_url SUPABASE_ANON_KEY=your_supabase_anon_key |
7. Using Chat2DB | For managing your Supabase database, consider utilizing Chat2DB (opens in a new tab). It simplifies database operations and helps visualize data structures and queries effortlessly. |
Integrating Supabase with React Native
Now that your development environment is ready, let’s dive into integrating Supabase into your React Native app with the following steps and code snippets.
Installing and Initializing Supabase Client
To initialize the Supabase client, create a new file named supabaseClient.js
in your project and add the following code:
import { createClient } from '@supabase/supabase-js';
const supabaseUrl = process.env.SUPABASE_URL;
const supabaseAnonKey = process.env.SUPABASE_ANON_KEY;
export const supabase = createClient(supabaseUrl, supabaseAnonKey);
Implementing Authentication Features
Supabase offers various authentication methods. Below is an example of implementing email/password authentication:
- Sign Up Functionality:
const signUp = async (email, password) => {
const { user, session, error } = await supabase.auth.signUp({
email,
password,
});
if (error) {
console.error('Error signing up: ', error);
} else {
console.log('User signed up: ', user);
}
};
- Login Functionality:
const login = async (email, password) => {
const { user, session, error } = await supabase.auth.signIn({
email,
password,
});
if (error) {
console.error('Error logging in: ', error);
} else {
console.log('User logged in: ', user);
}
};
- Password Recovery:
const recoverPassword = async (email) => {
const { data, error } = await supabase.auth.api.resetPasswordForEmail(email);
if (error) {
console.error('Error sending recovery email: ', error);
} else {
console.log('Recovery email sent: ', data);
}
};
Real-Time Database Capabilities
Supabase’s real-time capabilities can be leveraged to sync data in your application. Below is an example of subscribing to changes in a table:
const subscribeToTable = () => {
const subscription = supabase
.from('your_table_name')
.on('INSERT', payload => {
console.log('New row added: ', payload);
})
.subscribe();
return () => {
subscription.unsubscribe();
};
};
CRUD Operations
Supabase simplifies CRUD operations. Here's how to implement them in your React Native app:
- Create:
const createRow = async (data) => {
const { data: newData, error } = await supabase
.from('your_table_name')
.insert([data]);
if (error) {
console.error('Error creating row: ', error);
} else {
console.log('Row created: ', newData);
}
};
- Read:
const readRows = async () => {
const { data, error } = await supabase
.from('your_table_name')
.select('*');
if (error) {
console.error('Error reading rows: ', error);
} else {
console.log('Rows read: ', data);
}
};
- Update:
const updateRow = async (id, newData) => {
const { data, error } = await supabase
.from('your_table_name')
.update(newData)
.eq('id', id);
if (error) {
console.error('Error updating row: ', error);
} else {
console.log('Row updated: ', data);
}
};
- Delete:
const deleteRow = async (id) => {
const { data, error } = await supabase
.from('your_table_name')
.delete()
.eq('id', id);
if (error) {
console.error('Error deleting row: ', error);
} else {
console.log('Row deleted: ', data);
}
};
Incorporating error handling is crucial for a smooth user experience. Use try-catch blocks or check for errors in each operation to provide appropriate notifications to users.
Implementing Authentication
Supabase supports multiple methods for user authentication. Below are examples of setting up authentication flows in your React Native app.
Email/Password Authentication
To implement email/password authentication, refer to the previous sections for the sign-up and login functions.
Third-Party Providers
Supabase allows third-party authentication through providers like Google and GitHub. Here’s how to implement Google authentication:
const signInWithGoogle = async () => {
const { user, session, error } = await supabase.auth.signIn({
provider: 'google',
});
if (error) {
console.error('Error signing in with Google: ', error);
} else {
console.log('User logged in with Google: ', user);
}
};
Managing User Sessions
Utilize the following code to check user authentication status:
const user = supabase.auth.user();
if (user) {
console.log('User is logged in: ', user);
} else {
console.log('No user is currently logged in.');
}
Password Reset and Email Verification
For password resets and email verification, use the built-in functions provided by Supabase.
Monitoring User Data with Chat2DB
While developing your application, consider using Chat2DB (opens in a new tab) to monitor user data and authentication logs. Chat2DB's AI capabilities enable you to analyze user behavior and streamline database management.
Working with Supabase Database
Effectively utilizing Supabase’s PostgreSQL database is crucial for your React Native application. Here are steps to manage your database and perform operations:
Database Structure and Schema
A typical Supabase database consists of tables that define your application's data structure. Use the Supabase dashboard to create and manage your tables.
Creating Tables
In the Supabase dashboard, create tables by defining their schemas. For example, you might create a users
table with fields such as id
, email
, and password
.
Performing CRUD Operations in React Native
Utilize the previously defined CRUD functions to interact with your Supabase database from your React Native app.
Data Subscriptions for Real-Time Updates
Subscribing to data changes is a powerful feature of Supabase, allowing your app to stay updated with the latest data without manual refreshes.
Row Level Security (RLS)
To ensure data privacy and security, use Supabase’s Row Level Security (RLS). Define policies that control data access based on user roles and permissions.
Visualizing Database Schemas with Chat2DB
Using Chat2DB (opens in a new tab), you can effectively visualize and manage your database schemas. Its AI capabilities enhance your ability to analyze data and optimize queries.
Utilizing Supabase Storage for Media Management
Supabase offers robust storage solutions for managing media files in your React Native application. Below are steps to integrate media management.
Setting Up Storage Buckets
In the Supabase dashboard, create storage buckets to organize your media files.
Uploading and Downloading Media Files
Here’s how to upload and download images using Supabase storage:
- Upload Media:
const uploadMedia = async (file) => {
const { data, error } = await supabase.storage
.from('your_bucket_name')
.upload('path/to/file', file);
if (error) {
console.error('Error uploading media: ', error);
} else {
console.log('Media uploaded: ', data);
}
};
- Download Media:
const downloadMedia = async (path) => {
const { data, error } = await supabase.storage
.from('your_bucket_name')
.download(path);
if (error) {
console.error('Error downloading media: ', error);
} else {
const url = URL.createObjectURL(data);
console.log('Media downloaded: ', url);
}
};
Securing Access to Storage Files
Utilize Supabase’s permissions settings to secure access to your storage files. Define who can view or edit files in your storage buckets.
Enhancing Performance and Security
Optimizing performance and security in your Supabase-integrated React Native app is essential for a smooth user experience. Here are key considerations:
Reducing API Calls
Minimize the number of API calls by caching results where possible. Use local storage to save frequently accessed data.
Using Caching Mechanisms
Implement caching strategies to enhance performance and significantly reduce application load times.
Securing API Keys and Sensitive Data
Always keep your API keys secure. Use environment variables and ensure they are not hard-coded in your application.
Supabase Security Features
Leverage Supabase’s security features, including SSL encryption and database encryption, to protect your data.
Monitoring App Performance
Utilize tools to monitor app performance and track errors. This helps identify issues early and optimize your application.
Chat2DB for Monitoring
Consider using Chat2DB (opens in a new tab) for monitoring your database performance. Its AI features assist in identifying potential security issues and optimizing queries.
Exploring Advanced Features of Supabase and React Native
Once you have a basic understanding of integrating Supabase with React Native, explore advanced features that can enhance your application.
Using Supabase Functions
Supabase Functions allow you to execute server-side logic in response to database changes, useful for complex operations without overloading the client.
Implementing Complex Data Queries
Leverage Supabase's PostgreSQL capabilities for complex data queries and aggregations, helping you derive valuable insights from your data.
Integrating Third-Party Services
Explore integrating third-party services with Supabase, such as payment gateways or external APIs, to enrich your application’s functionality.
Utilizing Supabase’s Analytics Features
Supabase provides analytics features to track user behavior and app usage. Utilize these insights to refine your application further.
Advanced Query Testing with Chat2DB
Using Chat2DB (opens in a new tab), you can conduct advanced query testing and performance analysis to optimize your database interactions.
By embracing the capabilities of Supabase and React Native, while utilizing tools like Chat2DB, you can develop efficient and scalable mobile applications that meet the demands of today's users.
FAQ
-
What is Supabase?
- Supabase is an open-source Firebase alternative that provides backend tools like authentication and a real-time database.
-
How does React Native work?
- React Native allows developers to build mobile applications using JavaScript and React, enabling cross-platform compatibility.
-
Can I use Supabase for authentication?
- Absolutely! Supabase offers various authentication methods including email/password and third-party providers.
-
What is Chat2DB?
- Chat2DB is an AI-driven database visualization and management tool that simplifies database operations and enhances productivity.
-
How do I optimize my Supabase-integrated app?
- Implement caching, secure API keys, and utilize Supabase's security features while monitoring performance with tools like Chat2DB.
Consider switching to Chat2DB for managing your database interactions effectively. Its AI capabilities not only enhance your workflow but also provide insights that traditional tools lack, making it a superior choice for developers looking for efficiency and reliability.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!