How to Efficiently Integrate Flutter with Supabase: A Comprehensive Guide for Beginners
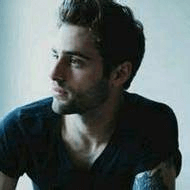
Understanding Flutter and Supabase: A Powerful Combination for App Development
Flutter is an open-source UI toolkit developed by Google (opens in a new tab) that enables developers to build natively compiled applications for mobile, web, and desktop from a single codebase. It streamlines the UI development process with a rich set of pre-designed widgets and tools, thereby enhancing productivity.
Supabase, on the other hand, is an open-source alternative to Firebase that provides a suite of backend tools, including a real-time database, authentication, and storage capabilities. By integrating Flutter's robust UI capabilities with Supabase's backend services, developers can create high-performance applications efficiently.
Combining Flutter with Supabase offers a powerful tech stack that incorporates both a dynamic front-end and a scalable back-end, allowing for rapid development cycles and improved application functionality. As open-source tools, Flutter and Supabase provide advantages over proprietary solutions, such as enhanced flexibility, community support, and cost-effectiveness.
Real-world applications, ranging from social media platforms to e-commerce sites, can significantly benefit from the integration of Flutter and Supabase to improve user experience and streamline development.
Setting Up Your Development Environment for Flutter and Supabase
To begin, you will need to set up your development environment for Flutter and Supabase. Follow these steps:
- Download and install the Flutter SDK (opens in a new tab), ensuring your system meets the necessary specifications.
- Install an IDE such as Android Studio (opens in a new tab) or Visual Studio Code (opens in a new tab) for an enhanced coding experience.
Creating a New Flutter Project
Use the Flutter CLI to create a new Flutter project by running the following command in your terminal:
flutter create my_flutter_app
Navigate into your project directory:
cd my_flutter_app
Setting Up Supabase
Next, sign up for a free account at Supabase (opens in a new tab) and create a new project. After setting up your project, you will receive your API keys and other credentials. Be sure to store these securely, as they will be needed for integrating Supabase with your Flutter application.
Configuring Environment Variables
To manage your API keys securely, you should use environment variables to store your Supabase credentials. Create a .env
file in your project root and add your keys:
SUPABASE_URL=https://your-supabase-url.supabase.co
SUPABASE_ANON_KEY=your-anon-key
Enhancing Your Development Experience
To further enhance your development experience, consider using tools like Chat2DB (opens in a new tab), an AI-powered database visualization management tool. Chat2DB is designed to boost database management efficiency through AI technology. It combines natural language processing with database management functions, enabling developers to interact with databases more intuitively.
Integrating Supabase with Flutter: Step-by-Step Guide
Now that your environment is set up, let's integrate Supabase with your Flutter application. Start by adding the Supabase Flutter package to your project. Include it in your pubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
supabase_flutter: ^0.2.0
Run flutter pub get
to install the package.
Configuring Supabase in Your Flutter App
In your main.dart
file, initialize Supabase using your credentials:
import 'package:flutter/material.dart';
import 'package:supabase_flutter/supabase_flutter.dart';
void main() async {
await Supabase.initialize(
url: 'https://your-supabase-url.supabase.co',
anonKey: 'your-anon-key',
);
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Supabase Demo',
home: HomeScreen(),
);
}
}
Implementing Authentication Features
One of the standout features of Supabase is its built-in authentication, which allows you to implement user sign-up, login, and password recovery easily. Here’s how to create a simple sign-up function:
Future<void> signUp(String email, String password) async {
final response = await Supabase.instance.client.auth.signUp(email, password);
if (response.error == null) {
print('User signed up successfully');
} else {
print('Error signing up: ${response.error!.message}');
}
}
For user login, you can implement the following code:
Future<void> login(String email, String password) async {
final response = await Supabase.instance.client.auth.signIn(email: email, password: password);
if (response.error == null) {
print('User logged in successfully');
} else {
print('Error logging in: ${response.error!.message}');
}
}
Building a Real-Time Application with Supabase
One of Supabase's most notable features is its real-time capabilities, which are essential for modern applications where data synchronization is crucial.
Implementing Real-Time Listeners
To implement real-time data listeners in Flutter, use the following code snippet:
void listenToMessages() {
Supabase.instance.client
.from('messages')
.on(SupabaseEventTypes.insert, (payload) {
print('New message received: ${payload.newRecord}');
}).subscribe();
}
This code listens for new messages in a 'messages' table and automatically updates the UI when new data is inserted.
Use Cases for Real-Time Applications
Real-time applications can include chat apps, collaborative tools, and dynamic dashboards. For example, a chat application can utilize real-time listeners to update the UI immediately when a new message is received, thereby enhancing user engagement.
Enhancing User Experience with Supabase Features
Supabase offers various features that can significantly enhance the user experience in your Flutter application.
Managing Media and File Uploads
Supabase provides storage capabilities for managing media files. You can upload files using the following code:
Future<void> uploadFile(String filePath) async {
final file = File(filePath);
final response = await Supabase.instance.client.storage
.from('uploads')
.upload('my_file.jpg', file.readAsBytesSync());
if (response.error == null) {
print('File uploaded successfully');
} else {
print('Error uploading file: ${response.error!.message}');
}
}
Creating Custom APIs
You can create custom APIs using Supabase functions to execute server-side logic. This allows you to extend your application's functionality without relying on external services.
Role-Based Access Control
Implementing role-based access control within your app is crucial for managing user permissions. With Supabase, you can easily enforce access rules to ensure that only authorized users can access certain data.
Deploying and Maintaining Your Application
Once your Flutter app is ready, careful planning is required for deployment. Continuous integration and deployment (CI/CD) pipelines can streamline this process.
Steps for Deployment
- Build your Flutter app for production:
flutter build web
- Deploy your application to web platforms or app stores, following best practices.
Monitoring and Maintenance
Post-deployment, monitoring your application is vital. Tools like Chat2DB (opens in a new tab) can help manage databases effectively, providing insights into performance and user behavior.
Scaling Your Application
As your application grows, you may need to scale it to handle increased user load. Supabase provides the infrastructure to support scaling, ensuring your app remains responsive even during peak usage.
Comparison Table of Database Management Tools
Feature | Chat2DB | DBeaver | MySQL Workbench | DataGrip |
---|---|---|---|---|
AI-Powered Database Management | Yes | No | No | No |
Natural Language Processing | Yes | No | No | No |
Community Support | Strong | Strong | Moderate | Moderate |
Cost | Free | Free/Open Source | Free | Subscription |
FAQ
-
What is Flutter? Flutter is an open-source UI toolkit by Google used for building natively compiled applications from a single codebase.
-
What is Supabase? Supabase is an open-source alternative to Firebase that offers tools including a real-time database, authentication, and storage.
-
How do I set up Supabase in my Flutter app? Install the Supabase Flutter package, initialize it with your credentials, and utilize its features like authentication and database interactions.
-
Can I use real-time capabilities with Supabase? Yes, Supabase provides real-time data synchronization features that allow your app to react instantly to database changes.
-
Why should I use Chat2DB for database management? Chat2DB is an AI-powered database management tool that enhances efficiency and simplifies database operations, making it an excellent choice for developers working with databases.
By following this comprehensive guide, you can successfully integrate Flutter with Supabase, creating powerful and efficient applications. Consider utilizing tools like Chat2DB (opens in a new tab) to streamline your database management process and take advantage of its advanced AI features!
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!