How to Implement Supabase RPC for Seamless Database Interactions
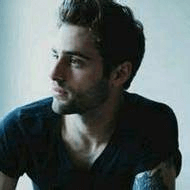
Understanding Supabase RPC and Its Significance in Modern Database Interactions
Remote Procedure Calls (RPC) are pivotal in enhancing the efficiency and performance of database interactions. They enable developers to execute predefined database functions directly from their applications, mitigating the overhead associated with traditional methods. Supabase, an open-source backend as a service (BaaS), utilizes RPC to streamline database operations, making it a preferred choice for developers seeking real-time capabilities and ease of use.
In this guide, we will explore how to implement Supabase RPC effectively for seamless database interactions, which are essential for application development. We will also introduce Chat2DB (opens in a new tab), a powerful tool for managing database interactions, emphasizing its AI-driven features that enhance database management efficiency.
Key Terms Defined
Term | Definition |
---|---|
RPC | Remote Procedure Call is a protocol enabling a program to execute a procedure in another address space. |
Supabase | An open-source Firebase alternative offering various backend services, including database hosting and real-time capabilities. |
BaaS | Backend as a Service provides cloud-based solutions allowing developers to build apps without managing backend infrastructure. |
Setting Up Your Supabase Environment for RPC
To start using Supabase RPC, you must set up your Supabase environment. Here’s how to do it step-by-step:
-
Sign Up for Supabase: Visit the Supabase website (opens in a new tab) and create an account. After logging in, you will be directed to your dashboard.
-
Create a New Project: Click on the "New Project" button. Fill in the project details, including the database password, which you will need later.
-
Configure Your Database: Upon project creation, set up your database by creating the necessary tables and schemas that your RPC functions will interact with. For example, in a task management application, create a table named
tasks
with fields likeid
,title
,description
, andstatus
. -
Secure Your Environment: Ensure your database is secure by using API keys and role-based access controls. These measures protect sensitive data from unauthorized access.
-
Integrate with Development Tools: Consider incorporating Chat2DB (opens in a new tab) into your workflow. This AI database visualization tool allows you to manage and visualize your database schemas effectively, simplifying interactions with your Supabase environment.
Common Pitfalls to Avoid
- Not Securing API Keys: Always keep your API keys private and avoid exposing them in public repositories.
- Ignoring Role Management: Properly manage user roles and permissions to prevent unauthorized access to your database.
Understanding Supabase RPC: An In-Depth Look
Supabase RPC stands out from traditional REST API calls by enabling developers to execute database functions directly. This approach allows batching of multiple operations into a single RPC call, minimizing latency and improving performance.
Advantages of Using Supabase RPC
- Reduced Latency: RPC allows executing multiple database functions in a single call, reducing the time spent on network communication.
- Improved Performance: For complex operations involving multiple queries, RPC can significantly enhance performance.
- Security: By using stored procedures, developers can encapsulate business logic within the database, minimizing exposure to SQL injection attacks.
Use Cases for RPC
- Data Aggregation: Simplifies the process of retrieving complex data from multiple tables.
- Batch Processing: Efficiently manages bulk operations on data through RPC.
Security Implications
While RPC is powerful, it carries security implications. Always validate inputs and implement appropriate security measures to protect against potential threats.
Implementing RPC in Supabase: A Step-by-Step Guide
Now that you understand the significance of RPC, let’s explore how to implement it in Supabase.
Step 1: Create a PostgreSQL Function
To create a PostgreSQL function that can be exposed as an RPC endpoint, use the following SQL code to retrieve tasks based on their status:
CREATE OR REPLACE FUNCTION get_tasks_by_status(status TEXT)
RETURNS TABLE(id INT, title TEXT, description TEXT) AS $$
BEGIN
RETURN QUERY SELECT id, title, description FROM tasks WHERE status = status;
END;
$$ LANGUAGE plpgsql;
Step 2: Expose the Function as an RPC Endpoint
After creating your function, expose it through Supabase. In the Supabase dashboard, navigate to the "SQL" section and run this command:
SELECT create_function('get_tasks_by_status', 'status TEXT');
Step 3: Call the RPC Function from Your Application
To call your new RPC function from a client application, use the Supabase client library. Here’s an example in JavaScript:
const { data, error } = await supabase
.rpc('get_tasks_by_status', { status: 'completed' });
if (error) console.error(error);
else console.log(data);
Using Chat2DB for Managing RPC Functions
Chat2DB (opens in a new tab) offers an intuitive interface for managing and visualizing your RPC functions. With its AI capabilities, you can easily generate SQL queries and visualize your database structure, streamlining the implementation and testing of RPC functions.
Testing and Debugging RPC Functions
Testing RPC functions is essential to ensure they perform as expected. Here are strategies you can use:
- Use Supabase Logs: Monitor logs for any errors or unexpected behavior when calling your RPC functions.
- Write Test Cases: Develop test cases to validate that your functions work under various scenarios.
Example Test Case
Here’s how to test your RPC function:
async function testGetTasksByStatus() {
const { data, error } = await supabase.rpc('get_tasks_by_status', { status: 'pending' });
if (error) {
console.error('Test failed:', error);
} else {
console.log('Test passed:', data);
}
}
testGetTasksByStatus();
Best Practices for Debugging
- Iterative Testing: Regularly test your functions during development to catch issues early.
- Use Chat2DB: Leverage Chat2DB (opens in a new tab) for a comprehensive view of database interactions, which can help quickly identify issues.
Optimizing Performance with Supabase RPC
To maximize the performance of your RPC functions, consider the following strategies:
- Efficient Indexing: Ensure your database tables are properly indexed to speed up query execution.
- Query Optimization: Analyze SQL queries for inefficiencies and optimize them accordingly.
Performance Monitoring
Utilize Supabase’s built-in analytics tools to monitor the performance of your RPC functions, helping you identify bottlenecks and improve response times.
Role of Caching
Implementing caching for frequently called RPC functions can significantly reduce load times and enhance user experience.
Advanced Use Cases and Best Practices for Supabase RPC
As you become more adept with Supabase RPC, explore advanced scenarios such as:
- Real-Time Data Synchronization: Use RPC to keep client applications updated with the latest data.
- Complex Transactions: Manage multi-step transactions efficiently by encapsulating them in RPC functions.
Documentation and Version Control
Maintain clear documentation for your RPC functions and implement version control to manage changes effectively.
Security Best Practices
Protect sensitive data by validating inputs and using prepared statements in your RPC functions to prevent SQL injection attacks.
Discover the Advantages of Using Chat2DB
As you implement Supabase RPC in your application, consider switching to Chat2DB (opens in a new tab) for your database management needs. Its AI-driven features streamline database interactions, including natural language SQL generation and intelligent data visualization. This tool enhances productivity and simplifies complex database operations, making it an excellent choice for developers and database administrators alike.
FAQ
-
What is Supabase RPC?
- Supabase RPC allows developers to execute predefined database functions directly from their applications, improving performance and reducing latency.
-
How do I create an RPC function in Supabase?
- You create a PostgreSQL function and expose it in the Supabase dashboard, allowing it to be called from client applications.
-
What are the benefits of using Chat2DB?
- Chat2DB (opens in a new tab) offers AI-driven features for database visualization, natural language SQL generation, and intelligent data management, enhancing efficiency.
-
How can I test my RPC functions?
- Use Supabase logs to monitor errors and develop test cases to validate your functions under various scenarios.
-
What security measures should I take when using RPC?
- Always validate inputs, use prepared statements, and manage permissions carefully to protect sensitive data from unauthorized access.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!