How to Effectively Integrate Supabase REST API into Your Web Applications
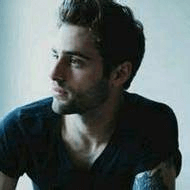
Understanding Supabase and REST APIs
Supabase is a powerful open-source alternative to Firebase, providing developers with essential tools to build scalable applications effortlessly. As a backend-as-a-service solution, it harnesses the capabilities of PostgreSQL—a robust relational database. With Supabase (opens in a new tab), developers can manage their databases, handle user authentication, and implement real-time features without requiring extensive backend expertise.
REST APIs (Representational State Transfer Application Programming Interfaces) play a vital role in facilitating communication between various software applications. They enable developers to interact with web services using standard HTTP protocols. The effectiveness of Supabase is largely attributed to its RESTful architecture, which automatically generates API endpoints based on the database schema via PostgREST (opens in a new tab). This feature simplifies performing operations such as Create, Read, Update, and Delete (CRUD) on data stored in PostgreSQL.
The growing popularity of Supabase stems from its user-friendliness and flexibility. Developers can swiftly set up projects and leverage the power of SQL for querying their data, making it an invaluable tool for modern web applications.
Setting Up a Supabase Project
Creating a Supabase project is a straightforward process. Start by visiting the Supabase (opens in a new tab) website and signing up for an account. Once logged in, follow these steps to create your first project:
-
Create a New Project: After logging in, click on the "New Project" button. Fill in your project details, including the project name, password, and database region.
-
Navigate the Dashboard: Familiarize yourself with the Supabase dashboard, where you can manage your database, user authentication, and API settings.
-
Database Setup: Use the intuitive interface to create a new database. Define your tables and columns according to your application's requirements. For example, to create a simple user table, you can run the following SQL command in the SQL editor:
CREATE TABLE users ( id SERIAL PRIMARY KEY, username VARCHAR(50) UNIQUE NOT NULL, email VARCHAR(100) UNIQUE NOT NULL, created_at TIMESTAMP DEFAULT NOW() );
-
Manage API Keys: Securely store your API keys and environment variables. These keys are crucial for interacting with the Supabase REST API.
-
Configure Authentication: Set up authentication methods, such as email/password or third-party providers like Google and GitHub.
By following these steps, you will have a fully functional Supabase project ready for integration with your web application.
Exploring Supabase REST API Features
The Supabase REST API provides several core features that simplify data interaction. To perform CRUD operations, you construct HTTP requests that interact with your API. Here’s a breakdown of common tasks:
Operation | Method | Example Code |
---|---|---|
Create | POST | javascript<br>const response = await fetch('https://your-project-ref.supabase.co/rest/v1/users', {<br> method: 'POST',<br> headers: {<br> 'Content-Type': 'application/json',<br> 'Authorization': `Bearer YOUR_API_KEY`<br> },<br> body: JSON.stringify({<br> username: 'john_doe',<br> email: 'john@example.com'<br> })<br>}); |
Read | GET | javascript<br>const response = await fetch('https://your-project-ref.supabase.co/rest/v1/users', {<br> method: 'GET',<br> headers: {<br> 'Authorization': `Bearer YOUR_API_KEY`<br> }<br>});<br>const users = await response.json(); |
Update | PATCH | javascript<br>const response = await fetch('https://your-project-ref.supabase.co/rest/v1/users?id=eq.1', {<br> method: 'PATCH',<br> headers: {<br> 'Content-Type': 'application/json',<br> 'Authorization': `Bearer YOUR_API_KEY`<br> },<br> body: JSON.stringify({<br> email: 'john_doe_updated@example.com'<br> })<br>}); |
Delete | DELETE | javascript<br>const response = await fetch('https://your-project-ref.supabase.co/rest/v1/users?id=eq.1', {<br> method: 'DELETE',<br> headers: {<br> 'Authorization': `Bearer YOUR_API_KEY`<br> }<br>}); |
Understanding the API endpoints and how they relate to your database schema is crucial for effective data management. Supabase’s use of PostgREST ensures that these endpoints are automatically generated, reducing the need for manual configuration.
Integrating Supabase REST API into Your Web Application
When integrating the Supabase REST API into your web application, it is essential to choose a front-end framework. Popular frameworks include React, Vue.js, and Angular. Below, we will demonstrate how to fetch and display data using Supabase in a React application.
Setting Up a React Application
-
Create a new React application using Create React App:
npx create-react-app my-app cd my-app
-
Install Axios for making HTTP requests:
npm install axios
-
In your
App.js
, you can fetch user data and display it:import React, { useEffect, useState } from 'react'; import axios from 'axios'; const App = () => { const [users, setUsers] = useState([]); useEffect(() => { const fetchUsers = async () => { const response = await axios.get('https://your-project-ref.supabase.co/rest/v1/users', { headers: { 'Authorization': `Bearer YOUR_API_KEY` } }); setUsers(response.data); }; fetchUsers(); }, []); return ( <div> <h1>User List</h1> <ul> {users.map(user => ( <li key={user.id}>{user.username} - {user.email}</li> ))} </ul> </div> ); }; export default App;
This code sets up a basic React application that fetches user data from your Supabase API and displays it in a list.
Real-time Capabilities and Webhooks
One of the standout features of Supabase is its ability to provide real-time capabilities through the REST API. This allows applications to react to changes in the database instantly. By implementing real-time subscriptions, developers can significantly enhance user experience.
For example, to enable real-time updates for the users table, you can use the following code snippet:
import { createClient } from '@supabase/supabase-js';
const supabase = createClient('https://your-project-ref.supabase.co', 'YOUR_API_KEY');
const subscription = supabase
.from('users')
.on('INSERT', payload => {
console.log('New user added:', payload.new);
})
.subscribe();
This code listens for new user entries and logs them to the console. Such real-time features are particularly beneficial for collaborative applications or live dashboards.
Additionally, configuring webhooks in Supabase can trigger actions based on specific database events. For example, you can set up a webhook to notify users when a new record is added.
Security Considerations and Best Practices
Securing your Supabase REST API is crucial to protect sensitive data. Here are some strategies to ensure security:
-
Use JWT Tokens: Implement JSON Web Tokens (JWT) for authentication. This adds an extra layer of security by ensuring that only authorized users can access certain resources.
-
Role-Based Access Control (RBAC): Configure RBAC within Supabase to restrict access to specific tables based on user roles.
-
Set Up Policies: Utilize Supabase’s policy management to define rules that govern who can access or modify data.
-
Secure API Keys: Keep your API keys confidential and avoid exposing them in client-side code.
-
Regular Updates: Regularly update your dependencies and monitor for security patches to reduce vulnerabilities.
By following these best practices, you can create a secure environment for your Supabase REST API.
Leveraging Chat2DB for Enhanced Database Management
When working with Supabase, consider integrating Chat2DB (opens in a new tab), an AI-powered database management tool that significantly enhances your database management experience. Chat2DB offers a user-friendly interface that helps developers interact with their Supabase database more efficiently. Its AI capabilities streamline database management tasks, allowing users to generate complex SQL queries naturally and visualize data intuitively.
For instance, you can use Chat2DB to quickly draft SQL queries without extensive SQL knowledge. The tool employs natural language processing to understand user input and generate the appropriate SQL commands. Here’s a simple example of creating a new user using Chat2DB:
-- Using Chat2DB to create a new user
CREATE TABLE users (
id SERIAL PRIMARY KEY,
username VARCHAR(50) UNIQUE NOT NULL,
email VARCHAR(100) UNIQUE NOT NULL,
created_at TIMESTAMP DEFAULT NOW()
);
Additionally, Chat2DB provides features like intelligent SQL editors and data analysis tools, making it an invaluable asset for developers and database administrators. By leveraging Chat2DB alongside Supabase, you can enhance productivity and streamline your database management processes, setting it apart from other tools like DBeaver, MySQL Workbench, and DataGrip.
Frequently Asked Questions
-
What is Supabase?
- Supabase is an open-source backend-as-a-service that provides developers with tools to build scalable applications. It is a powerful alternative to Firebase.
-
How do I set up a Supabase project?
- To set up a Supabase project, sign up on the Supabase website, create a new project, and configure your database and authentication settings.
-
What are REST APIs?
- REST APIs allow different software applications to communicate with each other using standard HTTP protocols, facilitating data exchange and interaction.
-
How can I secure my Supabase REST API?
- Use JWT for authentication, implement role-based access control, set up policies, and regularly update dependencies to enhance security.
-
What is Chat2DB?
- Chat2DB is an AI-powered database visualization management tool that enhances database management efficiency and smartens operations through natural language processing capabilities.
By understanding how to effectively integrate the Supabase REST API into your web applications, you can leverage its powerful features and capabilities. Additionally, utilizing Chat2DB can significantly enhance your database management experience, allowing you to focus on building innovative applications.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!