How to Seamlessly Integrate Supabase with React: A Comprehensive Guide
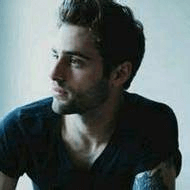
Integrating Supabase with React can significantly enhance your web development workflow. This powerful combination empowers developers to take advantage of a real-time database alongside a dynamic front-end library, enabling the creation of responsive applications. In this guide, we will explore how to effectively integrate Supabase with React, detailing everything from setup to deployment.
Understanding Supabase and React
What is Supabase?
Supabase (opens in a new tab) is an open-source alternative to Firebase that offers a comprehensive suite of backend services, including real-time databases, authentication, and storage. It simplifies the backend development process, allowing developers to concentrate on building their applications without the complexities of server management. With features like Postgres database support and real-time subscriptions, Supabase provides a scalable solution for modern web applications.
What is React?
React (opens in a new tab) is a popular JavaScript library designed for building user interfaces, particularly single-page applications. Its component-based architecture enables developers to create reusable UI components, simplifying the management of complex applications. By leveraging React, developers can craft dynamic interfaces that respond to user interactions instantaneously.
The Synergy Between Supabase and React
Integrating Supabase with React yields numerous benefits:
Benefit | Description |
---|---|
Real-Time Data | Supabase's real-time capabilities enable React applications to update instantly as data changes, enhancing user experience. |
Simplified Authentication | Supabase offers built-in authentication solutions that integrate seamlessly with React, streamlining user management. |
CRUD Operations | Supabase provides an intuitive API for performing CRUD (Create, Read, Update, Delete) operations, making data management straightforward. |
The combination of Supabase and React is increasingly favored by developers, particularly for applications such as real-time chat systems and collaborative tools. As the adoption of these technologies grows, mastering their integration becomes essential for contemporary web development.
Setting Up Your Development Environment
To start integrating Supabase with React, you'll need to prepare your development environment. Follow these steps:
Prerequisites
- Node.js: Ensure you have Node.js (opens in a new tab) installed on your machine.
- npm: This package manager comes with Node.js and helps manage dependencies.
- Code Editor: Utilize a code editor like Visual Studio Code (opens in a new tab) for an efficient development experience.
Creating a New React Project
To create a new React project, execute the Create React App command:
npx create-react-app my-supabase-app
cd my-supabase-app
Installing Supabase Client Library
Next, install the Supabase client library using npm:
npm install @supabase/supabase-js
Setting Up Environment Variables
Create a .env
file in the root of your project to securely manage your API keys:
REACT_APP_SUPABASE_URL=your_supabase_url
REACT_APP_SUPABASE_ANON_KEY=your_supabase_anon_key
Ensure you replace your_supabase_url
and your_supabase_anon_key
with the actual values from your Supabase project settings.
Creating a Supabase Account
- Visit the Supabase (opens in a new tab) website.
- Sign up for a free account.
- Create a new project and note down your Supabase URL and API keys.
Best Practices for Organizing Your Project
Organize your project directories effectively to maintain readability and manageability. Here’s a suggested structure:
my-supabase-app/
├── public/
├── src/
│ ├── components/
│ ├── hooks/
│ ├── pages/
│ ├── App.js
│ └── index.js
├── .env
└── package.json
Version Control with Git
Initialize a Git repository to track your changes:
git init
This allows you to collaborate with other developers and manage version history effectively.
Integrating Supabase with React
Now that your environment is set up, let's integrate Supabase into your React application.
Connecting React App to Supabase
Begin by creating a Supabase client instance in your src/index.js
file:
import { createClient } from '@supabase/supabase-js';
const supabaseUrl = process.env.REACT_APP_SUPABASE_URL;
const supabaseAnonKey = process.env.REACT_APP_SUPABASE_ANON_KEY;
export const supabase = createClient(supabaseUrl, supabaseAnonKey);
Configuring Authentication
Setting up user authentication with Supabase is a breeze. Create a simple authentication component as follows:
import React, { useState } from 'react';
import { supabase } from '../index';
const Auth = () => {
const [email, setEmail] = useState('');
const handleSignUp = async () => {
const { user, error } = await supabase.auth.signUp({ email });
if (error) console.error(error.message);
else console.log('User signed up:', user);
};
return (
<div>
<input
type="email"
onChange={(e) => setEmail(e.target.value)}
placeholder="Your email"
/>
<button onClick={handleSignUp}>Sign Up</button>
</div>
);
};
export default Auth;
Setting Up a Real-Time Database
To create a real-time database, set up a simple table in Supabase and connect it to your React application. Here’s how to fetch data from the database:
import React, { useEffect, useState } from 'react';
import { supabase } from '../index';
const DataDisplay = () => {
const [data, setData] = useState([]);
useEffect(() => {
const fetchData = async () => {
const { data: tableData, error } = await supabase
.from('your_table_name')
.select('*');
if (error) console.error(error.message);
else setData(tableData);
};
fetchData();
}, []);
return (
<div>
{data.map(item => (
<div key={item.id}>{item.name}</div>
))}
</div>
);
};
export default DataDisplay;
Implementing CRUD Operations
Implement CRUD operations to manage your data effectively:
const addItem = async (item) => {
const { data, error } = await supabase
.from('your_table_name')
.insert([{ name: item }]);
if (error) console.error(error.message);
else console.log('Item added:', data);
};
Managing State with Context API or Redux
For applications that require state management across multiple components, consider using the React Context API or Redux. Here’s a simple example utilizing Context API:
import React, { createContext, useContext, useReducer } from 'react';
const AppContext = createContext();
const initialState = {
user: null,
data: [],
};
const reducer = (state, action) => {
switch (action.type) {
case 'SET_USER':
return { ...state, user: action.payload };
case 'SET_DATA':
return { ...state, data: action.payload };
default:
return state;
}
};
export const AppProvider = ({ children }) => {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<AppContext.Provider value={{ state, dispatch }}>
{children}
</AppContext.Provider>
);
};
export const useAppContext = () => useContext(AppContext);
Real-Time Features with Supabase Subscriptions
Utilize Supabase's subscription model to provide real-time updates in your application. Here’s an example:
useEffect(() => {
const subscription = supabase
.from('your_table_name')
.on('*', payload => {
console.log('Change received!', payload);
// Update local state or perform actions based on the change
})
.subscribe();
return () => {
supabase.removeSubscription(subscription);
};
}, []);
Enhancing User Experience with Chat2DB
Chat2DB (opens in a new tab) is an AI-powered database visualization management tool that enhances the development experience when working with databases. Supporting over 24 databases, it provides a user-friendly interface for managing database interactions.
Features of Chat2DB
- Natural Language Queries: Effortlessly craft and execute complex queries using natural language processing, making database interactions intuitive.
- Table Management Simplified: Manage your tables without the hassle of cumbersome SQL commands, allowing you to focus on development.
- Data Insights: Leverage AI-driven analytics to gain insights into your data quickly.
By integrating Chat2DB with Supabase, developers can monitor database changes in real-time, improving productivity. Its intuitive interface simplifies complex database interactions, making it an ideal choice for React developers working with Supabase.
Setting Up Chat2DB with Supabase
To connect Chat2DB with Supabase, follow these steps:
- Download and install Chat2DB on your system.
- Configure it to connect with your Supabase database using the connection string provided in your Supabase dashboard.
- Utilize Chat2DB's features to visualize data changes and manage your database intelligently.
Leveraging the AI capabilities of Chat2DB allows developers to streamline their workflow, enhance data management, and ultimately improve application performance.
Deploying and Scaling Your Application
After integrating Supabase with your React application and utilizing tools like Chat2DB for database management, it’s time to deploy your application.
Preparing Your Application for Production
Before deployment, optimize your application by running:
npm run build
This command generates a production-ready build of your application.
Deployment Options
You can deploy your application on platforms like Vercel (opens in a new tab), Netlify (opens in a new tab), or AWS (opens in a new tab). Each platform offers unique features, allowing you to select the best fit for your project.
Ensuring Application Security
When deploying a live application, ensure that you:
- Use HTTPS to secure data transmission.
- Set up proper authentication and authorization for users.
- Regularly monitor for vulnerabilities and apply patches as necessary.
Setting Up Monitoring and Logging
For production applications, establish monitoring and logging to track user interactions and performance. This can help you identify issues promptly and enhance user experience.
Strategies for Scaling Your Application
As your application experiences increased traffic, consider:
- Database Optimization: Regularly analyze and optimize your database queries for efficiency.
- Load Balancing: Distribute incoming traffic across multiple servers to ensure reliability.
Continuous Improvement
Post-deployment, harness analytics tools to track user interactions and refine application features based on user feedback. Continuously monitor performance and make necessary adjustments to provide a seamless experience.
By seamlessly integrating Supabase with React and utilizing tools like Chat2DB, you can create powerful web applications that are efficient, scalable, and user-friendly.
FAQs
-
What is Supabase?
- Supabase is an open-source Firebase alternative offering real-time databases and backend services.
-
How does React work with Supabase?
- React can easily integrate with Supabase's API to manage data and user authentication.
-
What are the benefits of using Chat2DB?
- Chat2DB provides AI-powered database management, simplifying data visualization and management tasks.
-
Can I use Supabase for real-time applications?
- Yes, Supabase supports real-time data synchronization, making it ideal for applications like chat systems.
-
How do I deploy my Supabase-React application?
- Deploy your application on platforms like Vercel, Netlify, or AWS, following their deployment guidelines.
In conclusion, while there are tools like DBeaver, MySQL Workbench, and DataGrip available for database management, we encourage you to explore Chat2DB. Its AI features and user-friendly interface set it apart, making it an exceptional choice for developers looking to enhance their database management experience.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!