How to Implement Secure Authentication in Next.js with Supabase Auth
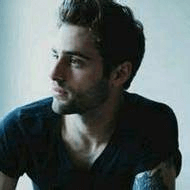
In the realm of web development, secure authentication is crucial for protecting user data and enhancing user experience. With the increasing popularity of frameworks like Next.js and backend services like Supabase, mastering secure authentication is essential for developers. This guide will delve into the intricacies of implementing secure authentication in Next.js applications using Supabase Auth, equipping you with the tools and knowledge to build secure web applications.
Understanding Secure Authentication in Next.js
To start, it’s vital to grasp what secure authentication means, particularly in the context of Next.js. Authentication verifies a user's identity, granting access to specific resources or functionalities within an application. Distinguishing between authentication and authorization is vital—authentication confirms identity, while authorization dictates access levels for users.
A cornerstone of secure authentication is the use of JSON Web Tokens (JWT). JWTs are compact, URL-safe tokens that represent claims exchanged between two parties, suitable for authentication and information exchange. Understanding common vulnerabilities outlined in the OWASP Authentication Cheat Sheet (opens in a new tab) is also crucial, as is ensuring user data protection through encryption and hashing.
Implementing two-factor authentication (2FA) can strengthen security by requiring users to provide two distinct authentication factors. Additionally, environmental variables are essential for safeguarding sensitive information, such as API keys and database credentials, preventing hard-coded exposure within the application.
Setting Up a Next.js Project
Before diving into secure authentication, you need to establish a Next.js project. Follow these steps:
-
Prerequisites: Ensure Node.js (opens in a new tab) and npm are installed on your machine.
-
Create a New Next.js Application:
npx create-next-app@latest my-nextjs-app cd my-nextjs-app
-
Project Directory Structure: Your project directory should resemble the following:
my-nextjs-app/ ├── node_modules/ ├── pages/ ├── public/ ├── styles/ ├── .env.local ├── package.json └── ...
-
Version Control: Initialize a Git repository to manage your code changes.
git init
-
Environment Variables: Create a
.env.local
file to store sensitive information.SUPABASE_URL=your_supabase_url SUPABASE_ANON_KEY=your_supabase_anon_key
-
Next.js Features: Utilize Next.js features like API routes and static site generation (SSG), which are advantageous for implementing secure authentication.
Introducing Supabase for Authentication
Supabase is a powerful alternative to Firebase, offering an open-source backend for developers. It includes a PostgreSQL (opens in a new tab) database and built-in authentication features.
Key Features of Supabase for Authentication:
Feature | Description |
---|---|
Pre-built UI Components | Offers pre-built authentication UI components, reducing development time for authentication forms. |
Social Logins | Easily integrate social login options like Google and GitHub to enhance user experience. |
Realtime Functionality | Manage user sessions and interactions effectively with real-time capabilities. |
When comparing Supabase with other authentication solutions like Auth0 (opens in a new tab) and Firebase Auth (opens in a new tab), its open-source nature and strong community support make it a compelling choice.
Implementing Supabase Authentication in Next.js
Let’s walk through the steps to integrate Supabase authentication within your Next.js application.
Step 1: Setting Up Supabase
- Create a Supabase Account: Visit supabase.io (opens in a new tab) and create an account.
- Create a New Project: After logging in, create a new project and set up your database.
Step 2: Configure Authentication
-
Enable Email and Password Authentication:
- Navigate to the "Authentication" section in your Supabase dashboard.
- Activate email/password logins and any desired social logins.
-
Install Supabase Client: Use the following command to install the Supabase client in your Next.js project:
npm install @supabase/supabase-js
Step 3: Create Authentication Pages
-
Sign Up Page: Create a new file in
pages/signup.js
:import { createClient } from '@supabase/supabase-js'; const supabase = createClient(process.env.SUPABASE_URL, process.env.SUPABASE_ANON_KEY); const SignUp = () => { const handleSignUp = async (event) => { event.preventDefault(); const { email, password } = event.target.elements; const { user, error } = await supabase.auth.signUp({ email: email.value, password: password.value, }); if (error) console.error(error); else console.log('User signed up:', user); }; return ( <form onSubmit={handleSignUp}> <input name="email" type="email" placeholder="Email" required /> <input name="password" type="password" placeholder="Password" required /> <button type="submit">Sign Up</button> </form> ); }; export default SignUp;
-
Login Page: Create a new file in
pages/login.js
:import { createClient } from '@supabase/supabase-js'; const supabase = createClient(process.env.SUPABASE_URL, process.env.SUPABASE_ANON_KEY); const Login = () => { const handleLogin = async (event) => { event.preventDefault(); const { email, password } = event.target.elements; const { user, error } = await supabase.auth.signIn({ email: email.value, password: password.value, }); if (error) console.error(error); else console.log('User logged in:', user); }; return ( <form onSubmit={handleLogin}> <input name="email" type="email" placeholder="Email" required /> <input name="password" type="password" placeholder="Password" required /> <button type="submit">Login</button> </form> ); }; export default Login;
Step 4: Handling User Sessions
Managing user sessions is crucial for any application. Use the following code to manage session state:
import { useEffect, useState } from 'react';
import { createClient } from '@supabase/supabase-js';
const supabase = createClient(process.env.SUPABASE_URL, process.env.SUPABASE_ANON_KEY);
const UserProfile = () => {
const [user, setUser] = useState(null);
useEffect(() => {
const userSession = supabase.auth.user();
setUser(userSession);
}, []);
return (
<div>
{user ? <p>Welcome, {user.email}</p> : <p>Please log in.</p>}
</div>
);
};
export default UserProfile;
Step 5: Error Handling
Provide user feedback during authentication errors:
const handleLogin = async (event) => {
event.preventDefault();
const { email, password } = event.target.elements;
const { user, error } = await supabase.auth.signIn({
email: email.value,
password: password.value,
});
if (error) {
alert('Login failed: ' + error.message);
} else {
console.log('User logged in:', user);
}
};
Securing Authentication with Advanced Techniques
To further secure authentication in your Next.js applications using Supabase, consider these advanced techniques:
Role-Based Access Control (RBAC)
Implementing RBAC allows for effective user role and permission management. By assigning roles, you can control access to specific features within your application.
Securing API Routes
Utilize middleware to secure your API routes, ensuring only authenticated users can access specific APIs:
import { createClient } from '@supabase/supabase-js';
const supabase = createClient(process.env.SUPABASE_URL, process.env.SUPABASE_ANON_KEY);
export default async function handler(req, res) {
const { user, error } = await supabase.auth.api.getUserByCookie(req);
if (error || !user) {
return res.status(401).send('Unauthorized');
}
res.status(200).json({ message: 'Authorized access' });
}
Using NextAuth.js
Consider integrating NextAuth.js (opens in a new tab) for enhanced security features. NextAuth.js offers a flexible authentication solution and can complement Supabase for managing user sessions.
Storing JWTs Securely
Securely storing JWTs on the client-side is vital. Use HTTP-only cookies to prevent XSS attacks and ensure tokens are transmitted securely.
Monitoring Authentication Events
Implement webhooks to monitor authentication events such as sign-ups, logins, and password resets, enabling quick responses to suspicious activity.
Integrating Chat2DB for Enhanced Database Management
Incorporating Chat2DB into your Next.js application can significantly elevate your database management capabilities. Chat2DB is an AI-powered database visualization management tool that simplifies database interactions. It supports over 24 databases and streamlines data management for developers.
Unique Features of Chat2DB:
Feature | Description |
---|---|
Natural Language SQL Generation | Users can generate SQL queries using natural language, enhancing accessibility for non-technical team members. |
Intelligent SQL Editor | Provides insights and suggestions while writing queries, improving efficiency and accuracy. |
Data Visualization | Easily create visual representations of data, simplifying analysis and presentation. |
Integration Process
Integrating Chat2DB with your Next.js and Supabase setup is straightforward. You can execute SQL queries directly from Chat2DB, making data management seamless.
Here’s an example of using Chat2DB to execute a SQL query:
SELECT * FROM users WHERE active = true;
This command retrieves all active users from your Supabase database, showcasing how Chat2DB simplifies your workflow.
Benefits of Using Chat2DB
Utilizing Chat2DB not only streamlines database management but also enhances collaboration within development teams. With its intuitive interface and real-time collaboration features, teams can work together efficiently while managing their databases.
Best Practices for Maintaining Secure Authentication
To ensure ongoing security in your Next.js application, adhere to the following best practices:
- Keep Dependencies Updated: Regularly update your libraries and dependencies to mitigate security vulnerabilities.
- Conduct Security Audits: Perform regular audits and assessments to identify potential security risks.
- User Education: Educate users on best practices for maintaining account security, such as strong passwords and recognizing phishing attempts.
- Monitor Authentication Logs: Implement logging to track authentication attempts and detect unusual patterns.
- Incident Response Plan: Develop a robust incident response plan to address potential security breaches effectively.
For further resources, consider visiting the OWASP Foundation (opens in a new tab) to stay informed about security trends and best practices.
FAQs
-
What is the difference between authentication and authorization?
- Authentication verifies a user's identity, while authorization determines which resources an authenticated user can access.
-
How does Supabase handle user authentication?
- Supabase provides built-in authentication features, allowing developers to easily implement sign-up, login, and session management.
-
What are JWTs, and why are they important?
- JSON Web Tokens are a compact method for securely transmitting information between parties, commonly used in authentication processes.
-
Can I integrate Chat2DB with any database?
- Yes, Chat2DB supports over 24 databases, making it a versatile tool for database management.
-
What are the advantages of using Chat2DB?
- Chat2DB offers AI-powered SQL generation, an intuitive interface, and real-time collaboration features, streamlining database management tasks.
Implementing secure authentication in Next.js with Supabase not only enhances the security of your application but also improves the overall user experience. By integrating tools like Chat2DB (opens in a new tab), you can further optimize your database management and development workflow. Switch to Chat2DB today for a superior database management experience!
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!