How to Efficiently Perform Joins in Supabase: A Comprehensive Guide
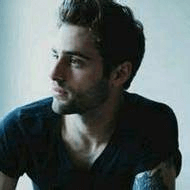
Understanding Supabase and SQL Joins
Supabase (opens in a new tab) is an open-source backend-as-a-service platform that serves as a powerful alternative to Firebase. Built on PostgreSQL, it provides developers with the tools they need to create scalable applications rapidly. One of the essential concepts in relational databases, including Supabase, is the use of joins. Joins enable you to combine rows from two or more tables based on related columns, facilitating complex data retrieval.
The primary types of joins include INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL OUTER JOIN. Each type serves different purposes:
Join Type | Description |
---|---|
INNER JOIN | Retrieves records with matching values in both tables. |
LEFT JOIN | Fetches all records from the left table and matched records from the right, returning NULL for unmatched rows. |
RIGHT JOIN | Returns all records from the right table and matched records from the left. |
FULL OUTER JOIN | Combines results from both LEFT and RIGHT joins, returning all records with NULL where there is no match. |
Understanding these join types is vital for efficient querying in relational databases. For instance, in a social media app, joining user data with posts can help fetch all posts by a particular user. Here’s an example of an INNER JOIN using SQL syntax:
SELECT users.id, users.username, posts.title
FROM users
INNER JOIN posts ON users.id = posts.user_id;
This query retrieves user IDs and usernames along with their post titles by matching user_id
from the posts table with the id
from the users table.
Setting Up Your Supabase Project
To start utilizing Supabase, follow these steps:
- Sign Up: Go to Supabase (opens in a new tab) and create an account.
- Create a New Project: Click on “New Project,” give it a name, and set a database password.
- Familiarize with the Dashboard: Explore the dashboard where you can manage your database, authentication, and storage.
- Define Schemas: Create a new database schema to house your tables.
Creating tables with defined relationships is crucial for efficient joins. Here’s how to set up tables for users and posts:
CREATE TABLE users (
id SERIAL PRIMARY KEY,
username VARCHAR(100) NOT NULL
);
CREATE TABLE posts (
id SERIAL PRIMARY KEY,
title VARCHAR(255) NOT NULL,
user_id INTEGER REFERENCES users(id)
);
After creating the tables, populate them with sample data:
INSERT INTO users (username) VALUES ('user1'), ('user2');
INSERT INTO posts (title, user_id) VALUES ('Post 1', 1), ('Post 2', 1), ('Post 3', 2);
To connect to Supabase, use client libraries or RESTful APIs. The Supabase documentation (opens in a new tab) provides detailed guidance.
To enhance your database management experience in Supabase, consider using Chat2DB (opens in a new tab), an AI-powered database visualization management tool. Chat2DB simplifies database interactions through intelligent features like natural language SQL generation and smart SQL editing.
Executing Basic Join Operations in Supabase
Now that your Supabase project is set up, let’s explore how to perform basic join operations.
INNER JOIN
The INNER JOIN operation selects records with matching values in both tables:
SELECT users.username, posts.title
FROM users
INNER JOIN posts ON users.id = posts.user_id;
This query returns a list of usernames along with their corresponding post titles.
LEFT JOIN
Next, let’s explore a LEFT JOIN, which retrieves all records from the left table and matched records from the right:
SELECT users.username, posts.title
FROM users
LEFT JOIN posts ON users.id = posts.user_id;
If user 2 has not posted anything, the query will return NULL
for their title.
RIGHT JOIN
Conversely, a RIGHT JOIN returns all records from the right table:
SELECT users.username, posts.title
FROM users
RIGHT JOIN posts ON users.id = posts.user_id;
This query fetches all posts, including those that may not have corresponding users.
FULL OUTER JOIN
A FULL OUTER JOIN combines results from both LEFT and RIGHT joins:
SELECT users.username, posts.title
FROM users
FULL OUTER JOIN posts ON users.id = posts.user_id;
This query returns all users and all posts, filling in NULL
where there is no match.
CROSS JOIN
Lastly, a CROSS JOIN retrieves the Cartesian product of both tables:
SELECT users.username, posts.title
FROM users
CROSS JOIN posts;
Common Errors with Joins
While working with joins, you might encounter common errors such as mismatched data types or incorrect join conditions. Always review your SQL syntax and ensure the columns you are joining on are compatible.
Optimizing Join Performance in Supabase
Optimizing join performance in Supabase applications is crucial for maintaining speed and efficiency. Here are several strategies to consider:
Indexing
Creating indexes on frequently joined columns can significantly improve query performance. For example, if you often join on user_id
, consider creating an index:
CREATE INDEX idx_user_id ON posts(user_id);
Query Optimization
Understand how Supabase's PostgreSQL engine handles complex queries. Analyze query execution plans to identify bottlenecks and adjust your queries accordingly.
Restructuring Queries
Sometimes restructuring your SQL queries can lead to performance improvements. Using subqueries wisely can help streamline join operations.
Database Normalization
Database normalization reduces redundancy and enhances join performance. By organizing your data efficiently, you can minimize the amount of data processed during joins.
Monitoring Tools
Utilize Supabase's built-in monitoring tools to track query performance. This will help you identify slow queries and take action to optimize them.
In addition, Chat2DB (opens in a new tab) offers capabilities to optimize and analyze database queries, making it a valuable asset in your toolkit.
Advanced Join Techniques in Supabase
As you become more comfortable with basic joins, consider exploring advanced techniques to enhance your data retrieval capabilities:
Self Joins
A self-join allows you to join a table to itself. This is useful in hierarchical data situations:
SELECT a.username AS Employee, b.username AS Manager
FROM users a
INNER JOIN users b ON a.manager_id = b.id;
Joining Multiple Tables
You can join multiple tables in a single query:
SELECT users.username, posts.title, comments.content
FROM users
INNER JOIN posts ON users.id = posts.user_id
INNER JOIN comments ON posts.id = comments.post_id;
Lateral Joins
Lateral joins allow subqueries to reference columns from preceding tables in the FROM clause, useful in advanced data retrieval scenarios.
Window Functions
Integrating window functions with joins allows for calculations across a set of rows related to the current row, providing deeper insights into your data.
Recursive Joins
Recursive joins are helpful for handling hierarchical data structures, allowing for querying parent-child relationships, common in organizational charts.
Common Table Expressions (CTEs)
Using CTEs with joins simplifies complex queries and improves readability:
WITH UserPosts AS (
SELECT users.username, posts.title
FROM users
INNER JOIN posts ON users.id = posts.user_id
)
SELECT * FROM UserPosts;
Integrating Supabase Joins in Applications
Integrating Supabase joins into real-world applications involves best practices for utilizing join operations effectively:
Backend Services
Use Supabase's client libraries in backend services to incorporate join operations, allowing efficient data fetching and manipulation. For example, when creating a RESTful API, implement joins to streamline data retrieval.
Frontend Applications
Frontend applications can consume join operations via Supabase's API. Frameworks like React or Vue.js can integrate these queries to display joined data efficiently.
Optimizing Network Calls
When dealing with joined data, optimize network calls to reduce latency. Batch requests and minimize the amount of data sent over the network.
Testing Join Queries
Thoroughly test your join queries to ensure accuracy and performance. Utilize Supabase's features to validate your queries before deploying them in production.
Chat2DB (opens in a new tab) serves as a powerful tool to visualize and manage join queries, enhancing your overall development experience with its intuitive interface and AI-powered features.
Troubleshooting and Debugging Join Queries
Common issues may arise while working with join queries in Supabase. Addressing these challenges effectively is crucial for successful database management.
Troubleshooting Strategies
When encountering errors related to join conditions or mismatched data types, reviewing your SQL syntax and data types is essential.
Debugging Techniques
Utilize debugging techniques to identify and fix inefficient queries. Supabase provides logging and monitoring features that help track query performance.
Interpreting Error Messages
Understanding the role of error messages can aid in quickly diagnosing problems. Pay attention to error codes and descriptions to pinpoint issues.
Using Chat2DB for Debugging
Chat2DB (opens in a new tab) can assist in debugging and optimizing join queries with its AI capabilities, providing insights into query performance and enhancing database management.
FAQs
-
What is Supabase?
- Supabase is an open-source alternative to Firebase, offering backend services like database management, authentication, and storage.
-
What are joins in SQL?
- Joins in SQL allow combining rows from two or more tables based on related columns, facilitating complex data retrieval.
-
How do I optimize join performance in Supabase?
- Optimize join performance by creating indexes, restructuring queries, and utilizing Supabase's monitoring tools.
-
Can I use Chat2DB with Supabase?
- Yes, Chat2DB is an AI-powered database visualization management tool that enhances database interactions and works seamlessly with Supabase.
-
What are advanced join techniques?
- Advanced join techniques include self-joins, joining multiple tables, lateral joins, and using Common Table Expressions (CTEs) for improved query management.
Embrace the power of Chat2DB in your database management tasks, leveraging its AI capabilities to streamline your workflows and optimize queries more efficiently than competing tools like DBeaver, MySQL Workbench, or DataGrip.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!