How to Seamlessly Integrate Supabase with Next.js
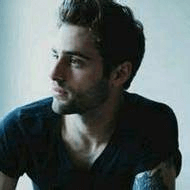
Understanding Supabase and Next.js: The Perfect Pair for Modern Web Development
Supabase is an open-source backend-as-a-service (BaaS) that harnesses the power of PostgreSQL for efficient database management. It equips developers with a plethora of features, such as authentication, real-time subscriptions, and storage solutions, which streamline the application-building process. By utilizing Supabase, developers can concentrate on crafting their applications without the burden of managing the underlying infrastructure.
Next.js, a robust React-based framework, supports server-side rendering (SSR) and static site generation (SSG), allowing developers to build optimized, high-performance web applications. The integration of Supabase (opens in a new tab) with Next.js (opens in a new tab) creates a powerful environment for developing scalable, full-stack applications effortlessly.
This combination enables developers to manage both backend and frontend seamlessly, facilitating real-time data updates, secure authentication, and enhanced user experiences.
Setting Up Your Development Environment for Supabase and Next.js
To kickstart your integration journey, follow these steps to set up your development environment:
Step | Action |
---|---|
1. | Install Node.js and npm: Ensure you have Node.js (opens in a new tab) installed, as npm (Node Package Manager) is included and essential for managing project dependencies. |
2. | Create a New Next.js App: Open your terminal and run the command below to set up a new Next.js application: npx create-next-app my-supabase-app cd my-supabase-app |
3. | Set Up Environment Variables: For security, handle sensitive data like your Supabase API keys through environment variables. Create a .env.local file in your project root and include your Supabase URL and API key: NEXT_PUBLIC_SUPABASE_URL=your_supabase_url NEXT_PUBLIC_SUPABASE_ANON_KEY=your_supabase_anon_key |
This setup ensures that your sensitive data remains secure while being accessible within your application.
Creating a Supabase Project and Database
Follow these steps to create a new Supabase project:
-
Create a Supabase Account
Sign up at the Supabase website (opens in a new tab). -
Create a New Project
After logging in, create a new project in the Supabase dashboard. Assign a name to your project and set a database password. -
Configure Your PostgreSQL Database
Navigate to the SQL editor in the Supabase dashboard to define your database schema. For instance, you can create a simple "users" table:CREATE TABLE users ( id serial PRIMARY KEY, username VARCHAR (50) UNIQUE NOT NULL, email VARCHAR (100) UNIQUE NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
-
Securely Integrate API Keys
Use the API keys stored in your.env.local
file for interactions with your Supabase project, ensuring your keys remain secure and accessible only during runtime.
Integrating Supabase with Next.js
Now that your Supabase project is ready, let’s integrate it with your Next.js application:
-
Install Supabase Client
Execute the following command to install the Supabase JavaScript client:npm install @supabase/supabase-js
-
Initialize Supabase Client
Create a new file namedsupabaseClient.js
in your project directory and initialize the Supabase client:import { createClient } from '@supabase/supabase-js'; const supabaseUrl = process.env.NEXT_PUBLIC_SUPABASE_URL; const supabaseAnonKey = process.env.NEXT_PUBLIC_SUPABASE_ANON_KEY; export const supabase = createClient(supabaseUrl, supabaseAnonKey);
-
Setting Up API Routes
Create a directory namedpages/api
and add a file calledusers.js
to handle user-related requests. Here’s an example of fetching users from your Supabase database:import { supabase } from '../../supabaseClient'; export default async function handler(req, res) { if (req.method === 'GET') { const { data: users, error } = await supabase.from('users').select('*'); if (error) return res.status(400).json({ error: error.message }); return res.status(200).json(users); } }
Handling Data with Supabase and Next.js
With Supabase integrated into your Next.js application, you can perform CRUD (Create, Read, Update, Delete) operations seamlessly. Here’s an example of how to create a new user:
-
Creating a User
Create an API route for adding new users:export default async function handler(req, res) { if (req.method === 'POST') { const { username, email } = req.body; const { data, error } = await supabase.from('users').insert([{ username, email }]); if (error) return res.status(400).json({ error: error.message }); return res.status(201).json(data); } }
-
Reading Users
To display users, create a simple component that fetches data from your API route:import { useEffect, useState } from 'react'; const UserList = () => { const [users, setUsers] = useState([]); useEffect(() => { const fetchUsers = async () => { const response = await fetch('/api/users'); const data = await response.json(); setUsers(data); }; fetchUsers(); }, []); return ( <ul> {users.map(user => ( <li key={user.id}>{user.username} - {user.email}</li> ))} </ul> ); }; export default UserList;
-
Updating a User
To update a user, use a similar API route:export default async function handler(req, res) { if (req.method === 'PATCH') { const { id, username } = req.body; const { data, error } = await supabase.from('users').update({ username }).eq('id', id); if (error) return res.status(400).json({ error: error.message }); return res.status(200).json(data); } }
-
Deleting a User
Finally, create an API route for deleting a user:export default async function handler(req, res) { if (req.method === 'DELETE') { const { id } = req.body; const { data, error } = await supabase.from('users').delete().eq('id', id); if (error) return res.status(400).json({ error: error.message }); return res.status(204).send(null); } }
Deploying Your Application
After developing your application, it's time to deploy it. Here’s how:
-
Choose a Hosting Provider
Consider using Vercel (opens in a new tab) for deploying your Next.js application, known for seamless integration and features like automatic scaling and serverless functions. -
Deploying to Vercel
Link your GitHub repository and follow the on-screen instructions to deploy your application. Vercel allows you to set environment variables, ensuring your Supabase keys remain secure. -
Troubleshooting Deployment Issues
If you encounter deployment issues, check the Vercel logs for error messages. Common pitfalls include misconfigured environment variables or problems with your Supabase integration.
Exploring Advanced Features and Third-party Integrations
Once your basic application is up and running, consider exploring advanced features:
-
Real-time Subscriptions
Supabase enables real-time subscriptions, allowing your application to receive updates whenever data changes in the database. This feature is particularly beneficial for chat applications or collaboration tools.import { useEffect } from 'react'; import { supabase } from '../../supabaseClient'; const RealTimeUsers = () => { useEffect(() => { const subscription = supabase .from('users') .on('INSERT', payload => { console.log('User added:', payload.new); }) .subscribe(); return () => { supabase.removeSubscription(subscription); }; }, []); return null; }; export default RealTimeUsers;
-
Edge Functions
Edge functions allow you to run serverless functions closer to users, reducing latency for applications that require quick responses. -
Enhancing Database Management with Chat2DB
For developers seeking to simplify database management, Chat2DB (opens in a new tab) is an exceptional tool. Chat2DB is an AI-driven database visualization management tool that supports over 24 databases and combines natural language processing with intelligent database functionalities.With features such as natural language query generation and intuitive data visualization, Chat2DB significantly enhances your database management experience. By leveraging its intelligent capabilities, developers can tackle complex tasks with ease, making data management more efficient and user-friendly.
Best Practices and Security Considerations
When developing applications with Supabase and Next.js, adhering to best practices is crucial:
-
Regular Database Backups
Ensure you regularly back up your Supabase database. Supabase offers automated backup options, vital for data recovery. -
Monitoring and Logging
Implement monitoring and logging mechanisms to track your application’s performance and identify potential issues. Tools like Sentry can help monitor errors in real time. -
Securing API Keys
Always keep your API keys and sensitive data secure by using environment variables, avoiding hardcoding sensitive information in your code. -
Performance Optimization
Optimize your application’s performance using caching strategies and lazy loading techniques to improve load times and user experience.
Frequently Asked Questions (FAQ)
-
What is Supabase?
Supabase is an open-source backend-as-a-service (BaaS) that provides features like authentication, real-time subscriptions, and storage solutions using PostgreSQL. -
How does Next.js enhance web applications?
Next.js enables server-side rendering and static site generation, resulting in faster and more SEO-friendly web applications. -
What are the advantages of integrating Supabase with Next.js?
This integration allows developers to manage backend and frontend seamlessly, providing real-time updates, secure authentication, and a scalable architecture. -
How can I secure my API keys in a Next.js application?
Secure your API keys by using environment variables stored in a.env.local
file, keeping sensitive information out of your codebase. -
What is Chat2DB, and how can it assist developers?
Chat2DB is an AI-powered database visualization management tool that enhances database management through natural language processing, simplifying SQL generation and data visualization for efficient database handling.
Integrating Chat2DB (opens in a new tab) into your development workflow can significantly improve your database management experience. Explore its features to streamline your development process and boost productivity!
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!