How to Integrate Supabase with Flutter: A Comprehensive Step-by-Step Guide
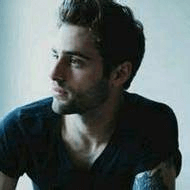
In today's rapidly evolving software development landscape, the demand for powerful yet easy-to-use tools is at an all-time high. Supabase serves as a robust open-source backend as a service (BaaS), providing essential features such as real-time databases, authentication, and storage solutions. Flutter, on the other hand, is a versatile UI toolkit that enables developers to create natively compiled applications for mobile, web, and desktop platforms from a single codebase. Together, Supabase and Flutter create a powerful synergy, allowing developers to build efficient and scalable applications quickly. This guide will walk you through the process of integrating Supabase with Flutter, enhancing your development experience with practical examples and best practices.
The Importance of Supabase and Flutter in Modern App Development
Understanding the critical role that Supabase plays in app development is essential. As an open-source BaaS, it simplifies the backend setup, allowing developers to focus on building features rather than managing server infrastructure. With real-time databases, developers can ensure that their applications reflect changes instantly, improving user engagement and experience.
Flutter, as a UI toolkit, provides an expressive and flexible way to create beautiful interfaces. It leverages a single codebase to produce high-performance applications on multiple platforms. This eliminates the need for separate codebases for iOS and Android, significantly reducing development time and effort. When combined, Supabase and Flutter enable developers to create applications that are not only functional but also visually appealing.
Setting Up Your Development Environment for Supabase and Flutter
Before diving into the integration, it's essential to set up your development environment correctly. This section will guide you through installing necessary software, ensuring you have everything ready for development.
Installing Flutter SDK
- Download Flutter SDK: Head over to the Flutter official website (opens in a new tab) and download the SDK according to your operating system (Windows, macOS, or Linux).
- Extract the SDK: Unzip the downloaded file to a location on your machine.
- Update Path: Add the Flutter
bin
directory to your system's PATH variable.- For Windows, search for "Environment Variables" in the Start menu, and update the PATH variable.
- For macOS and Linux, you can update the
.bash_profile
or.bashrc
file.
Setting Up an IDE
You can use any IDE, but Android Studio and Visual Studio Code are the most recommended for Flutter development.
- Android Studio: Download it from the Android Studio website (opens in a new tab) and install the Flutter and Dart plugins.
- Visual Studio Code: Install it from the Visual Studio Code website (opens in a new tab). Install the Flutter and Dart extensions from the marketplace.
Configuring Supabase CLI
To manage your backend, install the Supabase CLI:
- Install Node.js: Ensure you have Node.js (opens in a new tab) installed on your machine.
- Install Supabase CLI: Run the following command in your terminal:
npm install -g supabase
Now you are ready to start creating your project!
Creating a Supabase Project and Configuring Database
Creating a new project in Supabase is straightforward. Follow these steps to set up your database schema:
- Sign Up: Go to the Supabase website (opens in a new tab) and sign up for a free account.
- Create a New Project: Click on "New Project" and fill in the required details such as project name, password, and database region.
- Access the Dashboard: Once your project is created, you will be redirected to the Supabase dashboard.
Configuring Database Schema
-
Create Tables: Navigate to the "Table editor" section and create a new table. For example, let's create a
users
table:- Table Name: users
- Columns:
- id (integer, primary key, auto-increment)
- email (text, unique)
- password (text)
- created_at (timestamp, default value:
now()
)
-
Using SQL Editor: Alternatively, you can use the SQL editor to run the following SQL command:
CREATE TABLE users ( id SERIAL PRIMARY KEY, email TEXT UNIQUE, password TEXT, created_at TIMESTAMP DEFAULT now() );
Integrating Supabase with Flutter
Now that your Supabase project is set up, it's time to integrate it with your Flutter app.
Adding Supabase Package
Add the Supabase Flutter package to your project by updating the pubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
supabase_flutter: ^0.2.9
Run flutter pub get
to install the package.
Initializing Supabase Client
Now you need to initialize the Supabase client in your Flutter application. Update the main.dart
file as follows:
import 'package:flutter/material.dart';
import 'package:supabase_flutter/supabase_flutter.dart';
void main() async {
await Supabase.initialize(
url: 'YOUR_SUPABASE_URL',
anonKey: 'YOUR_ANON_KEY',
);
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: HomeScreen(),
);
}
}
Replace YOUR_SUPABASE_URL
and YOUR_ANON_KEY
with your actual Supabase project credentials.
Fetching Data from Supabase
To demonstrate fetching data, let’s create a simple screen that displays user data:
class HomeScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Supabase & Flutter')),
body: FutureBuilder(
future: Supabase.instance.client.from('users').select().execute(),
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return Center(child: CircularProgressIndicator());
}
if (snapshot.hasError) {
return Center(child: Text('Error: ${snapshot.error}'));
}
final List<dynamic> users = snapshot.data.data;
return ListView.builder(
itemCount: users.length,
itemBuilder: (context, index) {
return ListTile(title: Text(users[index]['email']));
},
);
},
),
);
}
}
This code snippet fetches the list of users from the Supabase users
table and displays their email addresses in a ListView.
Authentication and User Management in Flutter with Supabase
User authentication is crucial for most applications. Supabase provides a straightforward way to implement authentication mechanisms.
Setting Up Email/Password Authentication
- Sign Up: Implement a sign-up function using the Supabase’s auth feature:
Future<void> signUp(String email, String password) async {
final response = await Supabase.instance.client.auth.signUp(email, password);
if (response.error != null) {
print('Sign up error: ${response.error.message}');
} else {
print('User signed up: ${response.user.email}');
}
}
- Login: Implement a login function:
Future<void> login(String email, String password) async {
final response = await Supabase.instance.client.auth.signIn(email: email, password: password);
if (response.error != null) {
print('Login error: ${response.error.message}');
} else {
print('User logged in: ${response.user.email}');
}
}
- Logout: To log out the user:
Future<void> logout() async {
await Supabase.instance.client.auth.signOut();
print('User logged out');
}
Social Authentication
Supabase supports social authentication as well. For instance, to implement Google login:
final response = await Supabase.instance.client.auth.signInWithProvider(Provider.google);
This method will redirect the user to a Google sign-in page. Successful authentication will return the user's info.
Real-Time Data Handling in Flutter with Supabase
One of the standout features of Supabase is its real-time capabilities. You can set up real-time listeners to react to database changes instantly.
Setting Up Real-Time Listeners
Here’s how you can listen for changes in the users
table:
void listenToUserChanges() {
Supabase.instance.client
.from('users')
.on(SupabaseEventTypes.insert, (payload) {
print('New user added: ${payload.newRecord}');
}).subscribe();
}
This code snippet will print details to the console whenever a new user is added to the users
table.
Real-Time Updates in a Chat Application
To showcase real-time capabilities, consider a collaborative chat application where users can send messages. Set up a messages
table and listen for new messages:
void listenToMessages() {
Supabase.instance.client
.from('messages')
.on(SupabaseEventTypes.insert, (payload) {
print('New message: ${payload.newRecord}');
}).subscribe();
}
Storing and Serving Files with Supabase Storage
Supabase offers a storage feature that allows you to manage and serve files easily. This section will guide you through uploading and retrieving files.
Configuring Storage Buckets
- Create a Bucket: In the Supabase dashboard, navigate to the Storage section and create a new bucket. For example, create a bucket named
uploads
.
Uploading Files
To upload files from your Flutter app, use the following code:
Future<void> uploadFile(String filePath) async {
final file = File(filePath);
final response = await Supabase.instance.client.storage.from('uploads').upload('file_name', file);
if (response.error != null) {
print('Upload error: ${response.error.message}');
} else {
print('File uploaded!');
}
}
Retrieving Files
You can retrieve files using the following code snippet:
Future<void> getFile() async {
final response = await Supabase.instance.client.storage.from('uploads').download('file_name');
if (response.error != null) {
print('Download error: ${response.error.message}');
} else {
print('File downloaded!');
}
}
Testing and Debugging Your Flutter and Supabase Integration
Testing and debugging are crucial steps in the development process. Here are some strategies to ensure your application runs smoothly.
Using Flutter’s Built-in Test Framework
Flutter comes with a built-in test framework that can be utilized for unit and widget tests. Implement tests for your authentication flows and data fetching functions to ensure they work as expected.
Logging and Monitoring
Utilize logging to track errors and monitor the application’s behavior. Supabase’s dashboard also provides monitoring tools to keep an eye on backend activities.
Advanced Supabase Features and Customization
Once you're comfortable with the basics, explore the advanced features Supabase offers, such as:
Edge Functions
Edge Functions enable you to run custom server-side logic, which can be useful for processing data before sending it to your client.
Role-Based Access Control (RBAC)
Implementing RBAC allows you to define user roles and permissions efficiently, enhancing the security of your application.
Custom SQL Queries
Utilize custom SQL queries to perform complex data operations that are not achievable through the standard Supabase client methods.
Enhancing Your App with Third-Party Tools and Libraries
Integrating third-party tools can significantly enhance your app's capabilities. One such tool is Chat2DB (opens in a new tab), an AI-powered database visualization and management tool. It supports over 24 databases and simplifies database management through its intelligent SQL editor and natural language processing capabilities.
With Chat2DB, developers can generate SQL queries, perform data analysis, and create visualizations effortlessly. This tool allows you to spend less time managing the database and more time developing your application.
Comparison of Database Management Tools
Feature | Chat2DB | Other Tools (DBeaver, MySQL Workbench) |
---|---|---|
AI-Powered SQL Editor | Yes | No |
Natural Language Processing | Yes | No |
Multi-Database Support | 24+ Databases | Limited |
Data Visualization | Advanced | Basic |
User-Friendly Interface | Yes | Varies |
By leveraging Chat2DB, you can streamline your development workflow, making it easier to interact with your database while focusing on building features that matter. This tool provides a significant advantage over traditional database management solutions by enhancing productivity and efficiency.
FAQ
1. What is Supabase?
Supabase is an open-source backend as a service (BaaS) that provides features like real-time databases, authentication, and storage solutions.
2. How does Flutter work?
Flutter is a UI toolkit that allows developers to create natively compiled applications for mobile, web, and desktop from a single codebase.
3. What are the advantages of using Supabase with Flutter?
The integration offers a streamlined development process, real-time data handling, and efficient user authentication, allowing developers to create robust applications quickly.
4. What is Chat2DB?
Chat2DB is an AI-powered database visualization and management tool that enhances database management efficiency through intelligent features.
5. How can I integrate Chat2DB with my Flutter application?
While Chat2DB primarily focuses on database management and visualization, it can complement your Flutter app by streamlining database interactions and providing valuable insights during development.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!