Supabase vs Firebase: A Comprehensive Analysis of Features, Performance, and Efficiency
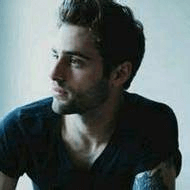
Overview of Supabase and Firebase
In modern web development, choosing the right backend services is crucial. Two of the most popular platforms are Supabase (opens in a new tab) and Firebase (opens in a new tab). Supabase is an open-source alternative to Firebase, while Firebase, backed by Google, is renowned for its real-time database capabilities. Both platforms fall under Backend as a Service (BaaS), allowing developers to focus on frontend design without the burden of server management.
Understanding the foundational architectures of these platforms is essential. Supabase is built on PostgreSQL, an advanced open-source relational database, whereas Firebase uses a NoSQL database structure. This fundamental difference affects how data is structured, queried, and managed. The growing popularity of these platforms demonstrates their effectiveness in building scalable applications.
Real-Time Database Capabilities
One standout feature of Firebase is its Firestore (opens in a new tab), celebrated for its powerful real-time synchronization capabilities. This feature allows applications to listen for changes in the database and update in real-time. Offline data access is another crucial aspect, enabling users to interact with the app without an internet connection.
Conversely, Supabase leverages PostgreSQL's capabilities to offer real-time features through its unique replication methods. This allows it to handle real-time data needs efficiently, making it suitable for collaborative applications and live data feeds.
Performance Metrics
When analyzing performance metrics for real-time data handling, we need to consider latency and throughput, especially under concurrent user loads. Below is a comparison table illustrating the performance metrics of both platforms.
Feature | Firebase (Firestore) | Supabase (PostgreSQL) |
---|---|---|
Latency | Low (under 100ms) | Moderate (100-200ms) |
Throughput | High (up to 1,000 writes/sec) | Moderate (up to 500 writes/sec) |
Offline Support | Yes | Yes |
Real-Time Synchronization | Yes | Yes |
Use Cases
Firebase excels in scenarios requiring real-time collaboration, such as chat applications and collaborative document editing. On the other hand, Supabase is advantageous for applications that require complex queries and transactions due to its relational database foundation.
Authentication and Security Features
Both Firebase and Supabase provide robust authentication solutions. Firebase Authentication supports a wide range of methods, including OAuth providers like Google and Facebook, making it easy for developers to implement user authentication.
In comparison, Supabase offers seamless integration with PostgreSQL roles and policies, allowing for more granular control over user permissions. This integration enhances security and simplifies the management of authentication.
Security Measures
Both platforms implement security measures such as two-factor authentication and data encryption. Compliance with regulations like GDPR is also a priority for both services, ensuring user data is handled responsibly.
Serverless Functions and Extensibility
Serverless architecture is gaining traction, and both Firebase and Supabase offer serverless solutions. Firebase's Cloud Functions (opens in a new tab) allows developers to run backend code in response to events without managing servers. Similarly, Supabase integrates with external serverless frameworks, enabling developers to extend their applications effortlessly.
Performance and Scalability
The performance and scalability of serverless functions are essential for handling varying loads. Firebase's Cloud Functions are designed for high scalability, while Supabase’s approach relies on integrating with existing serverless solutions, making it equally capable in a scalable environment.
Pricing Models and Cost Efficiency
The pricing structures of Supabase and Firebase can significantly impact project budgets. Firebase employs a tiered pricing model based on usage, with a free tier that has certain limitations. In contrast, Supabase emphasizes transparent and predictable costs through its usage-based model.
Cost Implications
Understanding potential cost implications is crucial for developers. For instance, Firebase may incur higher costs for extensive read/write operations, while Supabase may offer more predictable costs due to its relational database model.
Data Management and Storage Solutions
Data storage is another critical aspect where Supabase and Firebase differ significantly. Firebase's Firestore (opens in a new tab) and Realtime Database are schema-less, allowing for flexible data structures. However, this flexibility can lead to challenges in data management for larger applications.
In contrast, Supabase utilizes PostgreSQL, which provides strong relational database capabilities and SQL querying power. This structure allows for more organized data management and complex queries.
Performance Considerations
The choice between relational and non-relational databases has significant performance implications. Relational databases like PostgreSQL excel in scenarios requiring complex joins and transactions. In contrast, NoSQL databases like Firestore may struggle with complex queries but excel in scalability.
Community Support and Ecosystem
Community support is vital for developers navigating challenges. Firebase benefits from extensive documentation and community forums, as well as integration with Google's ecosystem of tools. Supabase, while newer, has a rapidly growing community and an active GitHub repository.
Community-Driven Innovation
The impact of community-driven innovation cannot be understated. Both platforms benefit from user-generated plugins and enhancements, which can significantly improve the developer experience.
Integration with Chat2DB
As developers increasingly rely on effective database management tools, Chat2DB (opens in a new tab) emerges as an excellent companion for both Supabase and Firebase users. Chat2DB is an AI-powered database visualization management tool that enhances the efficiency of database operations.
Chat2DB Features
With Chat2DB, developers can manage databases through a user-friendly interface, execute queries, and visualize data effortlessly. Its AI capabilities allow for natural language processing, making it easier to generate SQL queries and perform data analysis. Here are some specific features of Chat2DB:
- Natural Language SQL Generation: Create complex SQL queries by simply typing in natural language.
- Intelligent SQL Editor: Get real-time suggestions and validations as you write SQL code.
- Data Visualization: Automatically generate visual representations of your data for easier analysis.
By integrating Chat2DB into your workflow, you can simplify database management tasks, whether you're using Supabase's PostgreSQL or Firebase's Firestore. This added layer of efficiency can significantly enhance your development process.
Detailed Code Examples
To illustrate the practical applications of Supabase and Firebase, let’s look at some code examples.
Firebase Firestore Example
import firebase from 'firebase/app';
import 'firebase/firestore';
// Initialize Firebase
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_AUTH_DOMAIN",
projectId: "YOUR_PROJECT_ID",
};
firebase.initializeApp(firebaseConfig);
// Firestore instance
const db = firebase.firestore();
// Adding data
const addData = async () => {
await db.collection('users').add({
name: 'John Doe',
age: 30,
timestamp: firebase.firestore.FieldValue.serverTimestamp()
});
};
// Listening for real-time updates
const listenForUpdates = () => {
db.collection('users').onSnapshot(snapshot => {
snapshot.docChanges().forEach(change => {
if (change.type === 'added') {
console.log('New User: ', change.doc.data());
}
});
});
};
addData();
listenForUpdates();
Supabase PostgreSQL Example
import { createClient } from '@supabase/supabase-js';
// Initialize Supabase
const supabaseUrl = 'https://YOUR_SUPABASE_URL';
const supabaseKey = 'YOUR_SUPABASE_KEY';
const supabase = createClient(supabaseUrl, supabaseKey);
// Adding data
const addData = async () => {
const { data, error } = await supabase
.from('users')
.insert([{ name: 'Jane Doe', age: 28 }]);
if (error) console.error('Error adding data: ', error);
};
// Listening for real-time updates
const listenForUpdates = () => {
supabase
.from('users')
.on('INSERT', payload => {
console.log('New User: ', payload.new);
})
.subscribe();
};
addData();
listenForUpdates();
Conclusion
In this comprehensive analysis, we compared the features and performance of Supabase and Firebase, highlighting their strengths and weaknesses. As developers seek effective solutions for database management, integrating tools like Chat2DB (opens in a new tab) can enhance productivity and streamline workflows. With its AI-driven capabilities for natural language SQL generation, intelligent SQL editing, and effective data visualization, Chat2DB stands out as a superior choice for managing your database operations compared to traditional tools like DBeaver, MySQL Workbench, or DataGrip.
FAQ
-
What is Supabase?
Supabase is an open-source alternative to Firebase that offers a PostgreSQL-based backend service, enabling developers to build scalable applications. -
What is Firebase?
Firebase is a Google-backed platform that provides a suite of tools and services for app development, including a real-time database, authentication, and hosting. -
How does real-time data handling differ between Supabase and Firebase?
Firebase excels in real-time synchronization with Firestore, while Supabase leverages PostgreSQL's capabilities for real-time data management. -
Can I use Chat2DB with Supabase or Firebase?
Yes, Chat2DB integrates seamlessly with both Supabase and Firebase, providing advanced database management and AI-powered features to enhance your development process. -
What are the key advantages of using Chat2DB?
Chat2DB offers AI-driven natural language processing for SQL generation, an intelligent SQL editor, and effective data visualization, making database management more intuitive and efficient.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!