How to Master MySQL Date Format: A Comprehensive Guide for Developers
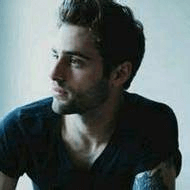
Understanding MySQL Date Format and Time Data Types
When working with MySQL, understanding the different date and time data types is crucial for effective data management. MySQL supports various types, including DATE (opens in a new tab), DATETIME (opens in a new tab), TIMESTAMP (opens in a new tab), TIME (opens in a new tab), and YEAR (opens in a new tab). Each of these types has unique characteristics and specific use cases.
Key Differences and Use Cases
Data Type | Format | Use Case |
---|---|---|
DATE | 'YYYY-MM-DD' | Best for storing dates without time, e.g., birthdates. |
DATETIME | 'YYYY-MM-DD HH:MM:SS' | Ideal for timestamps where both date and time are required. |
TIMESTAMP | 'YYYY-MM-DD HH:MM:SS' | Adjusts for time zones, suitable for applications with global users. |
TIME | 'HH:MM:SS' | Represents durations or times of day without a date. |
YEAR | 2 or 4 digits | Used for specific applications needing year-only information. |
Understanding how MySQL stores these values internally affects both storage size and retrieval speed. The default output format for MySQL date and time values is 'YYYY-MM-DD' for dates and 'YYYY-MM-DD HH:MM:SS' for datetime values.
Handling Time Zones and Leap Years
MySQL accounts for leap years and time adjustments, such as Daylight Saving Time (opens in a new tab), ensuring accurate date calculations. Utilizing the CONVERT_TZ()
function allows you to convert datetime values from one time zone to another, ensuring accurate timestamp representation across regions.
SELECT CONVERT_TZ(NOW(), 'UTC', 'America/New_York');
This query converts the current UTC time to Eastern Time.
Exploring Date Format Functions in MySQL
MySQL offers an extensive range of functions to format and manipulate date values effectively. One of the primary functions is DATE_FORMAT()
, which formats a date according to a specified format string.
Using DATE_FORMAT()
The syntax for DATE_FORMAT()
is:
DATE_FORMAT(date, format)
Common format specifiers include:
%Y
: Year, numeric, four digits%y
: Year, numeric (two digits)%m
: Month, numeric (01..12)%b
: Abbreviated month name%d
: Day of the month (01..31)
Here’s an example using DATE_FORMAT()
to format a date:
SELECT DATE_FORMAT(NOW(), '%Y-%m-%d %H:%i:%s') AS formatted_date;
Converting Strings to Date Values
If dates are stored as strings, the STR_TO_DATE()
function converts these strings into date values.
SELECT STR_TO_DATE('2023-10-15', '%Y-%m-%d') AS date_value;
Extracting Date Components
MySQL provides functions such as YEAR()
, MONTH()
, and DAY()
to extract specific components from date values.
SELECT YEAR(NOW()) AS current_year, MONTH(NOW()) AS current_month, DAY(NOW()) AS current_day;
Locale Settings in Date Formatting
Locale settings significantly affect date formatting. You can set or change these settings using the SET lc_time_names
command.
SET lc_time_names = 'fr_FR'; -- Set to French
Manipulating Dates with MySQL
MySQL allows various operations on date values, including adding or subtracting intervals. The DATE_ADD()
and DATE_SUB()
functions enable you to adjust dates easily.
Adding and Subtracting Intervals
The syntax for DATE_ADD()
is:
DATE_ADD(date, INTERVAL value unit)
Example:
SELECT DATE_ADD(NOW(), INTERVAL 1 MONTH) AS next_month;
This adds one month to the current date.
To subtract an interval, use DATE_SUB()
:
SELECT DATE_SUB(NOW(), INTERVAL 7 DAY) AS one_week_ago;
Calculating Differences Between Dates
To find the difference between two dates, use DATEDIFF()
:
SELECT DATEDIFF('2023-12-31', '2023-01-01') AS days_difference;
For time differences, TIMEDIFF()
is the suitable function:
SELECT TIMEDIFF('2023-10-15 10:00:00', '2023-10-15 08:30:00') AS time_difference;
Handling Edge Cases
When adding or subtracting intervals, be mindful of month or year boundaries. MySQL handles these cases gracefully, but it’s crucial to understand how your application logic interacts with these operations.
Best Practices for Storing and Retrieving Dates in MySQL
Storing date and time data properly in MySQL is essential for application performance and reliability. Here are some best practices to consider:
Choosing the Correct Data Type
Selecting the appropriate data type for your application's needs is fundamental. Use DATETIME
for timestamps and DATE
for simple date storage.
Indexing Date Columns
Indexing date columns can significantly improve query performance. Creating an index on a date column allows MySQL to quickly locate records based on date criteria.
CREATE INDEX idx_date ON events (event_date);
Managing Time Zone Settings
Ensure your application consistently handles time zone settings. Store datetime values in UTC and convert them to the user's local time for display. This approach prevents issues with daylight saving time and time zone differences.
Avoiding Implicit Conversions
Implicit conversions can lead to unexpected behavior. Always ensure that your date values are in the correct format before performing operations.
Using UTC for Global Applications
For applications serving users across multiple time zones, storing datetime values in UTC is a best practice. This ensures that time calculations remain consistent regardless of user location.
Integrating MySQL Date Formats with Applications
Integrating MySQL date formats into your application is crucial for effective data management. Different programming languages have varying approaches to handling date formats.
Using MySQL Date Functions in Programming
When using MySQL with languages like Python, Java, or PHP, ensure you incorporate MySQL date functions correctly. For instance, in Python's MySQL connector:
import mysql.connector
from datetime import datetime
db = mysql.connector.connect(user='user', password='password', host='host', database='database')
cursor = db.cursor()
cursor.execute("SELECT DATE_FORMAT(NOW(), '%Y-%m-%d %H:%i:%s')")
print(cursor.fetchone())
Validating Date Input in Web Applications
When developing web applications, it’s critical to validate date input on the client side. This practice ensures that only valid dates are sent to the server, reducing errors and improving user experience.
Handling User Preferences for Date Formats
For applications catering to a global audience, handling user preferences for date formats is essential. Allow users to select their preferred format, and ensure your application respects this choice when displaying dates.
Troubleshooting Common MySQL Date Format Issues
Developers often face challenges with MySQL date formats. Understanding common errors and their solutions can streamline your development process.
Common Error Messages
Errors such as "Incorrect date value" frequently occur due to invalid date formats. Always validate your inputs before executing queries.
Debugging Date-Related Issues
To troubleshoot date-related issues, check for null values or incorrect data types. Using IS NULL
or IS NOT NULL
can be helpful in identifying problematic records.
Handling Date Arithmetic Errors
When performing date arithmetic, watch for overflow or underflow errors. Ensure that your date calculations do not exceed the valid range for MySQL date types.
Advanced Techniques and Tools for Managing MySQL Date Formats
As you master MySQL date formats, explore advanced techniques and tools that enhance your capabilities.
Using Stored Procedures
Stored procedures can facilitate complex date manipulations. For instance, a stored procedure might automate monthly reporting based on date calculations.
MySQL Event Scheduler
The MySQL Event Scheduler (opens in a new tab) can automate date-based tasks, such as archiving records based on their timestamps.
Utilizing Chat2DB for Enhanced Management
For those seeking a more efficient way to manage and visualize date data in MySQL, consider using Chat2DB (opens in a new tab). This AI-powered database management tool simplifies operations by providing features like natural language SQL generation, intelligent SQL editing, and intuitive data visualization.
With Chat2DB, you can quickly manipulate date formats and perform complex queries with ease, making it an invaluable asset in your database management toolkit.
JSON Data Type for Date-Time Information
MySQL's JSON data type allows you to store date-time information alongside additional metadata. This approach can offer flexibility in managing date-related data.
Custom Functions for Unique Date Requirements
Creating custom functions can help handle specific date format requirements unique to your application.
CREATE FUNCTION CustomDateFormat(date_value DATE)
RETURNS VARCHAR(10)
BEGIN
RETURN DATE_FORMAT(date_value, '%d-%m-%Y');
END;
FAQ
-
What is the difference between DATE and DATETIME in MySQL?
- DATE stores only the date, while DATETIME includes both date and time components.
-
How can I convert a string to a date in MySQL?
- Use the
STR_TO_DATE()
function to convert strings into date values.
- Use the
-
What is the best way to handle time zones in MySQL?
- Store timestamps in UTC and convert them to the user's local time when displaying.
-
How do I index a date column in MySQL?
- You can create an index on date columns using the
CREATE INDEX
statement.
- You can create an index on date columns using the
-
What tools can help me manage MySQL date formats efficiently?
- Consider using Chat2DB (opens in a new tab) for advanced management and visualization of date data.
Explore these concepts further, and leverage tools like Chat2DB to enhance your MySQL date format mastery!
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!