Top Tips for Effortlessly Formatting SQL Queries: A Step-by-Step Guide
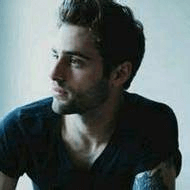
SQL queries are fundamental for interacting with databases, and their readability is crucial for effective collaboration among developers and database administrators. In this guide, we will explore essential tips and techniques for formatting SQL queries to enhance their clarity and maintainability. We will also showcase the powerful capabilities of Chat2DB (opens in a new tab), an AI-driven database management tool that simplifies the process of formatting SQL queries effortlessly.
Understanding the Importance of Proper SQL Query Formatting
Proper SQL query formatting is vital for several reasons:
-
Readability: Well-formatted queries improve understanding, making it easier for developers to grasp the logic behind a query. This is especially important in collaborative environments.
-
Maintainability: Correctly formatted queries are easier to modify and extend, which is essential in dynamic environments where requirements frequently change.
-
Performance: The structure of queries can impact execution plans, leading to improved performance.
Key Principles of SQL Query Formatting
When formatting SQL queries, several key principles can guide developers toward effective practices:
Principle | Description |
---|---|
Consistent Indentation | Helps visually distinguish different levels of query logic. |
Capitalization of Keywords | Improves visual clarity by differentiating SQL commands from user-defined elements. |
Line Breaks and Whitespace | Separates different clauses distinctly, making the query easier to scan. |
Aligning Related Elements | Enhances the visual structure of a query, making it easier to follow. |
Using Comments | Clarifies the purpose of complex queries, aiding in code reviews and future reference. |
Examples:
-
Consistent Indentation:
SELECT first_name, last_name FROM employees WHERE department = 'Sales';
-
Capitalization of SQL Keywords:
SELECT first_name, last_name FROM employees WHERE department = 'Sales';
-
Use of Line Breaks and Whitespace:
SELECT first_name, last_name FROM employees WHERE department = 'Sales' ORDER BY last_name;
-
Aligning Related Elements:
SELECT first_name, last_name FROM employees WHERE department = 'Sales' AND hire_date > '2020-01-01' ORDER BY last_name;
-
Using Comments:
-- Select employees from the Sales department who were hired after January 1, 2020 SELECT first_name, last_name FROM employees WHERE department = 'Sales' AND hire_date > '2020-01-01';
By adhering to these principles, developers can craft SQL queries that are not only functional but also visually appealing.
Step-by-Step Guide to Formatting SQL Queries
Formatting SQL queries effectively requires a systematic approach. Here is a step-by-step guide to help you format your queries like a pro:
1. Set Up a Preferred SQL Editor
Choose a SQL editor that supports formatting features. Tools like Chat2DB (opens in a new tab) offer advanced SQL formatting capabilities, making it simple to manage and format queries.
2. Write Simple SELECT Statements
Start with basic SQL statements and apply consistent indentation and capitalization.
SELECT
first_name,
last_name
FROM
employees;
3. Format Complex Queries
As queries grow in complexity, maintain readability by structuring JOINs and subqueries carefully.
SELECT
e.first_name,
e.last_name,
d.department_name
FROM
employees e
JOIN
departments d ON e.department_id = d.id
WHERE
d.location = 'New York';
4. Structure WHERE Clauses
Organize WHERE clauses for better readability, especially when using multiple conditions.
SELECT
first_name,
last_name
FROM
employees
WHERE
department = 'Sales'
AND hire_date > '2020-01-01'
AND status = 'Active';
5. Format GROUP BY and ORDER BY Clauses
Ensure that GROUP BY and ORDER BY clauses are clearly structured.
SELECT
department,
COUNT(*) AS employee_count
FROM
employees
GROUP BY
department
ORDER BY
employee_count DESC;
6. Handling Long SQL Scripts
When managing long SQL scripts, divide them into logical sections and use comments to describe each part.
-- Retrieve active employees from Sales department
SELECT
first_name,
last_name
FROM
employees
WHERE
department = 'Sales'
AND status = 'Active';
-- Retrieve employees hired in the last year
SELECT
first_name,
last_name
FROM
employees
WHERE
hire_date >= DATEADD(year, -1, GETDATE());
By following these steps, developers can create SQL queries that are structured, readable, and easy to maintain.
Utilizing Tools for SQL Formatting
Several tools are available to help developers format SQL queries efficiently. One standout tool is Chat2DB (opens in a new tab), which integrates AI capabilities to streamline SQL query management and formatting. Here are some additional tools worth considering:
Tool Type | Recommendations |
---|---|
Integrated Development Environments (IDEs) | IDEs like Visual Studio Code and DataGrip offer built-in SQL formatting features that enhance productivity. |
SQL Formatter Plugins | Plugins for popular editors can provide advanced formatting options. |
Online SQL Formatting Services | For quick formatting needs, online services can provide immediate results. |
Automatic Formatting Features | Many modern SQL editors come equipped with automatic formatting features, reducing manual adjustments. |
Common Mistakes to Avoid in SQL Query Formatting
When formatting SQL queries, awareness of common pitfalls can help enhance best practices:
-
Inconsistent Indentation: Failing to maintain consistent indentation can lead to confusion among team members.
-
Neglecting Capitalization: Not capitalizing SQL keywords can make queries harder to read and understand.
-
Excessive Nesting: Overly complex nested queries without comments can obscure logic.
-
Readability Issues: Long scripts can become unreadable if not formatted properly.
-
Over-reliance on Automated Formatting: While tools can help, understanding the principles behind formatting is crucial.
By avoiding these mistakes, developers can produce cleaner, more maintainable SQL code.
Advanced Formatting Techniques for Complex Queries
As queries grow more complex, advanced formatting techniques become essential. Here are some strategies for handling intricate SQL statements:
1. Efficiently Handling Multiple JOINs
When dealing with multiple JOINs, format them clearly to ensure each relationship is easy to follow.
SELECT
e.first_name,
e.last_name,
d.department_name,
m.first_name AS manager_first_name
FROM
employees e
JOIN
departments d ON e.department_id = d.id
JOIN
employees m ON e.manager_id = m.id;
2. Breaking Down Complex Logic
Breaking complex logic into manageable parts can make queries more understandable.
WITH SalesEmployees AS (
SELECT
first_name,
last_name
FROM
employees
WHERE
department = 'Sales'
)
SELECT
*
FROM
SalesEmployees
WHERE
hire_date > '2020-01-01';
3. Formatting Stored Procedures and Functions
When formatting stored procedures, maintain a clear structure for parameters and logic.
CREATE PROCEDURE GetEmployeeDetails
@Department NVARCHAR(50)
AS
BEGIN
SELECT
first_name,
last_name
FROM
employees
WHERE
department = @Department;
END;
4. Handling Recursive Queries
Recursive queries can be tricky; formatting them clearly is essential for understanding.
WITH RecursiveCTE AS (
SELECT
employee_id,
manager_id,
first_name,
last_name
FROM
employees
WHERE
manager_id IS NULL
UNION ALL
SELECT
e.employee_id,
e.manager_id,
e.first_name,
e.last_name
FROM
employees e
INNER JOIN
RecursiveCTE cte ON e.manager_id = cte.employee_id
)
SELECT * FROM RecursiveCTE;
Utilizing tools like Chat2DB (opens in a new tab) can significantly aid in formatting complex queries, providing intelligent suggestions and automations that enhance productivity.
Ensuring Consistency and Collaboration in SQL Formatting
Maintaining consistency in SQL query formatting across teams and projects is crucial. Here are some methods to achieve this:
-
Establishing SQL Style Guides: Create and adhere to a style guide that outlines formatting standards.
-
Code Review Processes: Implement code review practices to enforce formatting standards.
-
Version Control Systems: Use version control systems to manage formatted queries effectively.
-
Team Education: Educate team members about formatting best practices through workshops or training sessions.
-
Integrating Formatting Guidelines into Onboarding: Include formatting guidelines in the onboarding process for new team members.
-
Regular Team Discussions: Hold discussions about formatting approaches to ensure everyone is on the same page.
By fostering a culture of collaboration and consistency, teams can enhance the quality of their SQL queries significantly.
FAQ
-
What is the importance of formatting SQL queries? Formatting SQL queries improves readability, maintainability, and collaboration among team members.
-
How can I format SQL queries effectively? Use consistent indentation, capitalize SQL keywords, and separate clauses with line breaks and whitespace.
-
What tools can help with SQL query formatting? Tools like Chat2DB (opens in a new tab), IDEs, and SQL formatter plugins can assist in formatting SQL queries.
-
What are common mistakes in SQL query formatting? Common mistakes include inconsistent indentation, neglecting capitalization, and excessive nesting.
-
How can I ensure consistency in SQL query formatting across my team? Establish style guides, implement code review processes, and educate team members about best practices.
By leveraging these tips and techniques, developers can format SQL queries effortlessly, enhancing their effectiveness and overall collaboration within their teams. Don't forget to explore the powerful features of Chat2DB (opens in a new tab) to streamline your SQL formatting process! With its AI capabilities, Chat2DB not only simplifies SQL query management but also enhances your overall productivity compared to tools like DBeaver, MySQL Workbench, and DataGrip. Switch to Chat2DB today for a more efficient SQL experience!
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!