How to Use CASE Statements in SQL: A Comprehensive Guide
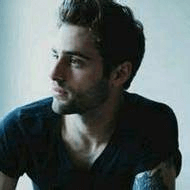
Understanding and implementing CASE statements in SQL is a crucial skill for anyone working with databases. This powerful feature allows you to perform conditional logic within your SQL queries, transforming your data and generating insightful reports. In this comprehensive guide, we will explore the basics of CASE statements, how to implement simple and searched CASE statements, and advanced techniques. Additionally, we will introduce Chat2DB, an AI-powered database management tool that significantly enhances your SQL query development experience.
Understanding the Basics of CASE Statements in SQL
CASE statements provide a way to implement conditional logic in SQL queries, allowing developers to execute different actions depending on the data values. The syntax of a basic CASE statement consists of the CASE
, WHEN
, THEN
, and ELSE
clauses.
Basic Syntax
Here's the basic syntax of a CASE statement:
SELECT column_name,
CASE
WHEN condition1 THEN result1
WHEN condition2 THEN result2
ELSE result3
END AS alias_name
FROM table_name;
Purpose and Utility
The primary purpose of CASE statements is to create calculated fields, handle NULL values, and categorize data. For example, consider a scenario where you want to categorize employees based on their salary:
SELECT employee_id, salary,
CASE
WHEN salary < 30000 THEN 'Low'
WHEN salary BETWEEN 30000 AND 70000 THEN 'Medium'
ELSE 'High'
END AS salary_category
FROM employees;
This example categorizes employees into three groups based on their salary, which can be helpful in reporting and analysis.
Comparison with Other Control Flow Tools
It's essential to compare CASE statements with other SQL control flow tools, such as IF statements. While both serve similar purposes, CASE statements are more versatile as they can be used directly in SELECT statements, whereas IF statements are typically used in stored procedures or functions.
Common Misconceptions
A common misconception is that CASE statements are only for simple conditions. In reality, they can handle complex scenarios involving multiple conditions and even nested CASE statements.
Step-by-Step Guide to Implementing Simple CASE Statements
Implementing simple CASE statements involves identifying the condition you want to evaluate and the corresponding actions. Let’s walk through the process.
Constructing a Simple CASE Statement
- Identify the Condition: Determine the field to be evaluated.
- Define the Actions: Specify what should happen when each condition is met.
Here’s a straightforward example of a simple CASE statement:
SELECT product_id, product_name,
CASE product_status
WHEN 'A' THEN 'Available'
WHEN 'O' THEN 'Out of Stock'
ELSE 'Discontinued'
END AS product_availability
FROM products;
Handling Multiple Conditions
You can handle multiple conditions within a single CASE statement by adding more WHEN clauses. For example:
SELECT student_id, grade,
CASE
WHEN grade >= 90 THEN 'A'
WHEN grade >= 80 THEN 'B'
WHEN grade >= 70 THEN 'C'
ELSE 'D or F'
END AS letter_grade
FROM students;
Best Practices for Writing CASE Statements
When writing CASE statements, keep these best practices in mind:
- Maintain Readability: Ensure your CASE statement is easy to read and understand.
- Include ELSE Clause: Always include an ELSE clause to handle unexpected values.
- Test Thoroughly: Validate your CASE statements to ensure they return the correct results.
Implementing Searched CASE Statements for Complex Conditions
Searched CASE statements are suitable for handling complex conditions that involve non-equality checks. The syntax is similar but allows for more flexibility.
Definition and Use Cases
A searched CASE statement evaluates conditions that can include comparisons beyond simple equality. Here’s an example:
SELECT employee_id, hours_worked,
CASE
WHEN hours_worked > 40 THEN 'Overtime'
WHEN hours_worked = 40 THEN 'Full-time'
ELSE 'Part-time'
END AS employee_type
FROM employee_hours;
Logical Operators
You can use logical operators to create more complex conditions. For example, categorizing products based on price ranges:
SELECT product_id, price,
CASE
WHEN price < 20 THEN 'Budget'
WHEN price BETWEEN 20 AND 50 THEN 'Mid-range'
WHEN price > 50 THEN 'Premium'
END AS price_category
FROM products;
Advantages of Searched CASE Statements
Using searched CASE statements enhances your query's flexibility and simplifies implementing intricate logic. They also facilitate efficient data transformation, particularly useful in reporting scenarios.
Advanced Techniques and Best Practices for CASE Statements
Integration with Other SQL Functions
CASE statements can be integrated with other SQL functions and clauses, such as JOINs and GROUP BY. This integration enhances the data processing capabilities of your queries.
Nested CASE Statements
Sometimes, you might need to nest CASE statements for more intricate data processing. Here’s an example:
SELECT employee_id, salary,
CASE
WHEN salary < 30000 THEN
CASE
WHEN performance_rating = 'Excellent' THEN 'Promotion Eligible'
ELSE 'Not Eligible'
END
ELSE 'No Action Needed'
END AS promotion_status
FROM employees;
Dynamic Query Generation
CASE statements are powerful in dynamic query generation, allowing you to build queries based on user input or other variables.
Debugging and Refining CASE Expressions
When working with complex CASE expressions, debugging can be challenging. Utilize SQL tools to test and refine your queries, ensuring they behave as expected.
Leveraging Chat2DB for Efficient SQL Query Development
Chat2DB is an innovative AI-powered database management tool that greatly enhances SQL query development. With features designed to support developers in writing and optimizing SQL queries, Chat2DB can significantly improve your experience when working with CASE statements.
Features of Chat2DB
Feature | Description |
---|---|
Code Suggestions | Provides intelligent code suggestions as you write your SQL queries, helping you craft accurate CASE statements quickly. |
Syntax Validation | Validates your SQL syntax in real-time, ensuring your queries are error-free before execution. |
Natural Language Processing | Allows you to generate SQL queries using natural language, making it easier to implement complex logic without deep SQL knowledge. |
By utilizing Chat2DB, you can streamline the development process for complex SQL queries, including those that leverage CASE statements.
Advantages of Using Chat2DB
Users have reported significant improvements in productivity and accuracy when using Chat2DB for SQL development. The AI capabilities allow for faster query generation and better handling of complex conditions.
Getting Started with Chat2DB
To get started with Chat2DB, simply download the client for your operating system and begin exploring its powerful features. With support for over 24 databases, Chat2DB is designed to meet the needs of developers, database administrators, and data analysts alike.
FAQs
Q1: What is a CASE statement in SQL?
A1: A CASE statement is a conditional expression in SQL that allows you to execute different actions based on varying conditions within your queries.
Q2: How do you write a simple CASE statement?
A2: A simple CASE statement follows this structure: CASE WHEN condition1 THEN result1 WHEN condition2 THEN result2 ELSE result3 END
.
Q3: What is the difference between simple and searched CASE statements?
A3: Simple CASE statements evaluate a single expression against multiple values, while searched CASE statements evaluate multiple conditions that can include complex logical expressions.
Q4: Can I use CASE statements with other SQL functions?
A4: Yes, CASE statements can be integrated with other SQL functions and clauses, such as JOINs and GROUP BY, to enhance query functionality.
Q5: How can Chat2DB assist with SQL query development?
A5: Chat2DB provides AI-powered features like code suggestions, syntax validation, and natural language processing to streamline SQL query development, making it easier to implement complex logic, including CASE statements.
By mastering CASE statements in SQL and leveraging tools like Chat2DB, you can enhance your data manipulation and reporting capabilities, ultimately leading to more insightful analyses and decisions. If you are currently using tools like DBeaver, MySQL Workbench, or DataGrip, consider switching to Chat2DB for a more efficient and user-friendly SQL development experience.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!