How to use CASE WHEN in SQL for Dynamic Data Manipulation
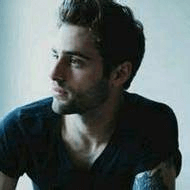
The 'CASE WHEN' statement in SQL is a powerful tool for dynamic data manipulation, enabling users to create complex queries that can return different values based on specific conditions. This article will explore the foundational aspects of 'CASE WHEN', its applications in dynamic data manipulation, advanced techniques, integration with other SQL features, and practical use cases. By understanding how to effectively utilize 'CASE WHEN', you can enhance your SQL queries and make your data more actionable. We will also introduce Chat2DB, an AI-driven database management tool that simplifies SQL operations, including the use of 'CASE WHEN'.
Understanding 'CASE WHEN' in SQL
The 'CASE WHEN' statement is a pivotal feature in SQL that allows for conditional logic within queries. It provides a way to return different values based on the evaluation of specific conditions, making it an essential tool for data transformation and enhancing query flexibility.
Syntax and Basic Structure
The basic syntax of a 'CASE WHEN' statement is as follows:
SELECT column_name,
CASE
WHEN condition1 THEN result1
WHEN condition2 THEN result2
ELSE result_default
END AS alias_name
FROM table_name;
This structure allows SQL developers to define multiple conditions that can lead to various outcomes. In scenarios where none of the conditions are met, the 'ELSE' clause provides a default value to be returned.
Comparison with IF-ELSE Statements
While 'CASE WHEN' is similar to IF-ELSE statements in programming, it is specifically designed for use within SQL queries. The primary difference lies in its ability to handle multiple conditions in a more concise manner.
Importance in Data Transformation
Using 'CASE WHEN' enhances the readability of datasets by allowing for conditional formatting. For example, consider a dataset containing employee salaries. By utilizing 'CASE WHEN', you can categorize employees based on their salary ranges:
SELECT employee_name,
salary,
CASE
WHEN salary < 50000 THEN 'Low'
WHEN salary BETWEEN 50000 AND 100000 THEN 'Medium'
ELSE 'High'
END AS salary_category
FROM employees;
In this example, employees are categorized as 'Low', 'Medium', or 'High' based on their salary. This categorization makes it easier to analyze salary distributions and identify trends.
Dynamic Data Manipulation with 'CASE WHEN'
The 'CASE WHEN' statement allows for dynamic data manipulation by enabling users to categorize and modify data outputs based on specific conditions. This capability is particularly useful in scenarios where data needs to be analyzed or transformed on-the-fly.
Categorizing Data
One of the most common applications of 'CASE WHEN' is in categorizing data into distinct groups. For example, in a sales dataset, you may want to classify sales performance as 'Above Average', 'Average', or 'Below Average':
SELECT salesperson,
sales_amount,
CASE
WHEN sales_amount > 100000 THEN 'Above Average'
WHEN sales_amount BETWEEN 50000 AND 100000 THEN 'Average'
ELSE 'Below Average'
END AS performance_category
FROM sales_data;
Modifying Data Outputs
'CASE WHEN' can also be utilized to dynamically modify data outputs. For instance, you might want to apply discounts based on customer loyalty:
SELECT customer_name,
purchase_amount,
CASE
WHEN loyalty_points > 100 THEN purchase_amount * 0.9 -- 10% discount
ELSE purchase_amount
END AS final_amount
FROM purchases;
In this scenario, loyal customers receive a discount, while others pay the full amount.
Updating Data with Conditional Logic
You can also use 'CASE WHEN' in conjunction with the UPDATE statement to conditionally modify records in a table:
UPDATE products
SET price = CASE
WHEN category = 'Electronics' THEN price * 0.9 -- 10% discount for electronics
ELSE price
END;
This query updates the prices of products in the 'Electronics' category, applying a discount while leaving other categories unchanged.
Combining with Aggregate Functions
The versatility of 'CASE WHEN' extends to its combination with aggregate functions, facilitating advanced data analysis. For instance, when calculating total sales by category:
SELECT category,
SUM(CASE
WHEN sale_date >= '2022-01-01' THEN sales_amount
ELSE 0
END) AS total_sales_2022
FROM sales
GROUP BY category;
Here, the total sales for each category are calculated based on whether the sale occurred in 2022.
Advanced Techniques and Nested 'CASE WHEN'
As users become more proficient with SQL, they can leverage advanced techniques involving nested 'CASE WHEN' statements. This method allows for handling multiple layers of conditions, adding complexity and depth to queries.
Constructing Nested 'CASE WHEN' Statements
Nested 'CASE WHEN' statements can be structured to manage intricate logic. For instance, consider a scenario where you want to classify grades based on a range of scores:
SELECT student_name,
score,
CASE
WHEN score >= 90 THEN 'A'
WHEN score >= 80 THEN
CASE
WHEN score = 89 THEN 'A-'
ELSE 'B'
END
ELSE 'C'
END AS grade
FROM student_scores;
In this example, students are graded based on their scores, with additional conditions for specific scores.
Performance Considerations
While nested 'CASE WHEN' statements can enhance functionality, they may also introduce performance considerations. It’s essential to optimize queries to avoid excessive computational overhead.
Best Practices for Readability and Performance
To maintain the readability and efficiency of queries, follow these best practices:
- Keep Logic Simple: Avoid overly complex nested conditions.
- Use Aliases: Provide clear aliases for calculated fields.
- Minimize Nesting: Limit the depth of nested 'CASE WHEN' statements to enhance performance.
Integrating 'CASE WHEN' with Other SQL Features
The integration of 'CASE WHEN' with other SQL features can significantly enhance query capabilities. By combining it with JOINs, GROUP BY, and ORDER BY clauses, users can create more sophisticated queries.
Combining with JOINs
Using 'CASE WHEN' in conjunction with JOINs allows for selective data retrieval and manipulation across related tables:
SELECT a.customer_name,
a.order_total,
CASE
WHEN b.loyalty_status = 'Gold' THEN 'Premium Customer'
ELSE 'Regular Customer'
END AS customer_type
FROM orders a
JOIN customers b ON a.customer_id = b.id;
Using with GROUP BY and ORDER BY
When combined with GROUP BY and ORDER BY clauses, 'CASE WHEN' can further refine data organization:
SELECT category,
COUNT(*) AS num_products,
CASE
WHEN COUNT(*) > 50 THEN 'Large Category'
ELSE 'Small Category'
END AS category_size
FROM products
GROUP BY category
ORDER BY num_products DESC;
Window Functions and Subqueries
The benefits of using 'CASE WHEN' extend to window functions, allowing for advanced analytical tasks:
SELECT employee_name,
sales_amount,
SUM(sales_amount) OVER (PARTITION BY department ORDER BY sales_amount DESC) AS department_sales_total,
CASE
WHEN sales_amount = department_sales_total THEN 'Top Seller'
ELSE 'Regular Seller'
END AS seller_status
FROM sales;
This query categorizes employees based on their sales performance relative to their department.
Practical Examples and Use Cases
The versatility of 'CASE WHEN' shines through in practical examples and real-world use cases. Below are several scenarios where 'CASE WHEN' adds significant value.
Use Case | SQL Example |
---|---|
Financial Data Analysis | sql SELECT transaction_id, amount, CASE WHEN amount > 1000 THEN 'High Value' WHEN amount BETWEEN 500 AND 1000 THEN 'Medium Value' ELSE 'Low Value' END AS transaction_category FROM transactions; |
Customer Segmentation in Marketing | sql SELECT customer_id, total_spent, CASE WHEN total_spent > 500 THEN 'VIP' WHEN total_spent BETWEEN 250 AND 500 THEN 'Regular' ELSE 'New' END AS customer_segment FROM customer_purchases; |
Health Data Reporting | sql SELECT patient_id, condition, CASE WHEN condition IN ('Diabetes', 'Hypertension') THEN 'Chronic' ELSE 'Non-Chronic' END AS condition_type FROM patient_records; |
Time Series Data Analysis | sql SELECT date, sales, CASE WHEN sales > LAG(sales) OVER (ORDER BY date) THEN 'Increase' WHEN sales < LAG(sales) OVER (ORDER BY date) THEN 'Decrease' ELSE 'Stable' END AS trend FROM daily_sales; |
Inventory Management | sql SELECT product_id, stock_quantity, CASE WHEN stock_quantity < 10 THEN 'Reorder' ELSE 'Sufficient Stock' END AS stock_status FROM inventory; |
Optimizing SQL Queries with 'CASE WHEN'
When utilizing 'CASE WHEN', it is crucial to optimize SQL queries to enhance performance and maintainability. Here are some strategies to consider:
Indexing Techniques
Implementing proper indexing can significantly improve query performance, especially for tables with large datasets. Consider adding indexes to frequently queried columns.
Structuring Queries
Structure queries to minimize computational overhead. Avoid unnecessary complexity by keeping conditions straightforward and concise.
Trade-offs Between Complexity and Performance
When using complex 'CASE WHEN' logic, be aware of the trade-offs between query complexity and performance. Strive for efficiency while delivering the required functionality.
Best Practices for Efficiency
To ensure efficient and maintainable 'CASE WHEN' statements, adhere to the following best practices:
- Use Descriptive Aliases: This enhances readability and understanding.
- Limit Nested Conditions: Simplifying your logic improves performance.
- Benchmark Queries: Regularly evaluate the performance of your queries to identify areas for optimization.
Case Study with Chat2DB
Chat2DB, an AI-driven database management tool, significantly enhances the use of 'CASE WHEN' for dynamic data manipulation. By leveraging natural language processing, developers and database administrators can generate SQL queries more intuitively.
Features of Chat2DB
In Chat2DB, 'CASE WHEN' plays a critical role in enabling users to execute complex queries effortlessly. The tool's intelligent SQL editor offers auto-completion and suggestions, making it easier to construct queries incorporating 'CASE WHEN' logic.
User Experience Improvements
Feedback from users indicates that the integration of 'CASE WHEN' within Chat2DB simplifies data interactions, allowing for more efficient data management and analysis. The AI capabilities of Chat2DB minimize the learning curve for new users, enabling them to perform complex SQL operations without extensive prior knowledge.
Future Plans for Expansion
As Chat2DB continues to evolve, there are plans to expand the functionality of 'CASE WHEN' within the platform, providing even more dynamic data manipulation options for users.
By utilizing Chat2DB, you can streamline your SQL operations, making dynamic data manipulation with 'CASE WHEN' more accessible and efficient compared to other tools like DBeaver, MySQL Workbench, and DataGrip.
FAQs
-
What is the purpose of 'CASE WHEN' in SQL? 'CASE WHEN' is used to implement conditional logic in SQL queries, allowing users to return different values based on specific conditions.
-
Can 'CASE WHEN' be nested in SQL? Yes, 'CASE WHEN' statements can be nested to handle multiple layers of conditions, enhancing the complexity and depth of queries.
-
How does 'CASE WHEN' improve data readability? By categorizing data into understandable groups based on conditions, 'CASE WHEN' makes datasets more interpretable and actionable.
-
What are some common use cases for 'CASE WHEN'? Common use cases include financial data analysis, customer segmentation, health data reporting, and inventory management.
-
How can I optimize SQL queries using 'CASE WHEN'? To optimize SQL queries, consider indexing, structuring queries efficiently, and following best practices to enhance performance and maintainability.
By mastering 'CASE WHEN' in SQL and utilizing tools like Chat2DB (opens in a new tab), you can greatly enhance your data manipulation capabilities and streamline your database management processes.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!