Integrating Prisma with Supabase: A Comprehensive Guide for Developers
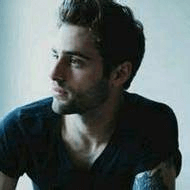
Leveraging the Power of Prisma and Supabase for Modern Applications
Prisma is an open-source next-generation Object-Relational Mapping (ORM) tool that empowers developers to build scalable and efficient databases. It provides various benefits such as type safety, auto-completion, and comprehensive migration capabilities. With Prisma, developers can focus on writing code rather than worrying about database queries, making it a preferred choice for many.
On the other hand, Supabase (opens in a new tab) is an open-source alternative to Firebase, offering a suite of backend services including authentication, database, and storage solutions. Supabase leverages PostgreSQL (opens in a new tab) as its database engine, emphasizing simplicity and scalability. The combination of Prisma's ORM capabilities and Supabase's backend services provides a robust framework for building modern applications.
The synergy between Prisma and Supabase allows developers to manage their database schema efficiently while utilizing powerful backend services. This integration ensures that applications are not only fast but also maintainable over time.
Setting Up the Environment
To integrate Prisma with Supabase, developers need to set up their environment correctly. Here are the prerequisites for a successful integration:
Requirement | Description |
---|---|
Node.js | Ensure that Node.js is installed on your machine. You can download it from the official website (opens in a new tab). |
Package Manager | Use npm or yarn for package management. You can install yarn via npm with the following command: npm install --global yarn |
Basic Understanding of JavaScript or TypeScript | Familiarity with either language is essential for effective development. |
Initial Project Setup
Create a new project directory and initialize it:
mkdir prisma-supabase-integration
cd prisma-supabase-integration
npm init -y
Next, install the necessary CLI tools for Prisma and Supabase:
npm install prisma @prisma/client
npm install supabase
Initialize Prisma in your project:
npx prisma init
This command creates a prisma
directory containing a schema.prisma
file where you define your database schema and connection settings.
Configuring Environment Variables
To connect to Supabase, you need to set up environment variables. In your .env
file, add the following line:
DATABASE_URL="your_supabase_database_url"
Replace your_supabase_database_url
with your actual Supabase database URL, which you can find in your Supabase project settings.
Connecting Prisma to Supabase
Connecting Prisma to Supabase's PostgreSQL database involves several steps. First, you need to generate your Supabase database URL from the Supabase dashboard.
Once you have the URL, open the schema.prisma
file and configure the datasource as follows:
datasource db {
provider = "postgresql"
url = env("DATABASE_URL")
}
Introspecting the Supabase Database Schema
To introspect your database schema, run the following command:
npx prisma db pull
This command fetches the schema from your Supabase database and updates your schema.prisma
file accordingly.
Generating Prisma Client
Once your schema is set up, generate the Prisma Client, which allows you to interact with your database:
npx prisma generate
You can now use Prisma Client to perform operations on your Supabase database.
Database Schema Management
Managing your database schema using Prisma with Supabase is straightforward. The Prisma schema file allows you to define your data models, directly impacting your database structure.
Defining Models
Here's an example of defining a simple User
model in your schema.prisma
file:
model User {
id Int @id @default(autoincrement())
name String
email String @unique
createdAt DateTime @default(now())
}
Performing Migrations
To perform database migrations, first, create a new migration with the following command:
npx prisma migrate dev --name init
This command creates a new migration file with the changes you made to your schema. You can then apply the migration to your database:
npx prisma migrate deploy
Tracking Schema Changes
It's essential to keep track of schema changes over time. Prisma's migration tools make it easy to manage and apply changes, ensuring synchronization between your development and production environments.
Implementing CRUD Operations
Implementing CRUD (Create, Read, Update, Delete) operations using Prisma with Supabase is quite efficient. Below are examples of how to perform these operations.
Create Operation
To create a new user, use the following code snippet:
const { PrismaClient } = require('@prisma/client');
const prisma = new PrismaClient();
async function createUser(name, email) {
const newUser = await prisma.user.create({
data: {
name: name,
email: email,
},
});
console.log('User created:', newUser);
}
// Usage
createUser('John Doe', 'john@example.com');
Read Operation
To read users from the database, implement the following function:
async function getUsers() {
const users = await prisma.user.findMany();
console.log('Users:', users);
}
// Usage
getUsers();
Update Operation
To update an existing user, use this code snippet:
async function updateUser(userId, newName) {
const updatedUser = await prisma.user.update({
where: { id: userId },
data: { name: newName },
});
console.log('User updated:', updatedUser);
}
// Usage
updateUser(1, 'Jane Doe');
Delete Operation
Finally, to delete a user, you can use the following function:
async function deleteUser(userId) {
const deletedUser = await prisma.user.delete({
where: { id: userId },
});
console.log('User deleted:', deletedUser);
}
// Usage
deleteUser(1);
Advanced Features and Optimization
Integrating Prisma with Supabase opens up numerous advanced features and optimization techniques. One notable feature is the use of Prisma middleware, which can be utilized for logging and authentication purposes.
Leveraging Real-time Capabilities
Supabase offers real-time capabilities, enabling developers to respond to changes in the database instantaneously. This feature can be leveraged alongside Prisma for building dynamic applications.
Query Optimization Techniques
To optimize database queries, consider the following techniques:
- Batching: Group multiple database operations into a single request to reduce overhead.
- Pagination: Implement pagination to manage large datasets efficiently.
Visualizing Database Operations with Chat2DB
During development, visualizing and interacting with your database can enhance productivity. Chat2DB (opens in a new tab) is an AI-driven database visualization management tool that simplifies database management. It supports over 24 databases and combines natural language processing with database management functions.
With Chat2DB, developers can generate SQL queries using natural language, providing a user-friendly interface for database interactions. Its intelligent SQL editor and capabilities for data analysis and visualization make it an invaluable tool for developers working with Prisma and Supabase.
Deployment and Production Considerations
When deploying an application integrated with Prisma and Supabase, several factors need to be considered:
Environment Configuration
Ensure that your environment variables are correctly configured for production. This includes setting up the production database URL and any necessary secrets.
Monitoring and Logging
Implement monitoring and logging to keep track of application performance and database interactions. Tools like Chat2DB can assist in monitoring database operations in a live environment, providing insights into performance bottlenecks.
Automated Deployment Pipelines
Consider setting up automated deployment pipelines to streamline the deployment process. This can significantly reduce the time required for updates and ensure that your application is always up to date.
Scaling Strategies
As your application grows, it's essential to have scaling strategies in place. Monitor your application's performance and adjust your infrastructure as necessary to handle increased load.
FAQs
-
What is Prisma?
- Prisma is an open-source ORM that helps developers build scalable and efficient databases with features like type safety and auto-completion.
-
What is Supabase?
- Supabase is an open-source backend-as-a-service platform that provides database, authentication, and storage services, built on PostgreSQL.
-
How do I connect Prisma to Supabase?
- You need to configure the database URL in your
schema.prisma
file and use the Prisma CLI commands to introspect and generate the client.
- You need to configure the database URL in your
-
What are the advantages of using Chat2DB?
- Chat2DB offers AI-driven database management, allowing users to generate SQL queries using natural language and providing tools for data visualization and analysis. This sets it apart from traditional tools like DBeaver or MySQL Workbench, which may not offer the same level of intelligent assistance.
-
Can I deploy a Prisma and Supabase application to production?
- Yes, you can deploy a Prisma and Supabase integrated application. Ensure proper environment configuration, monitoring, and scaling strategies are in place.
By integrating Prisma with Supabase, developers can leverage the strengths of both platforms to build robust applications efficiently. For an even more streamlined development process, consider using Chat2DB (opens in a new tab) to enhance your database management experience. Embrace the future of database interactions with AI capabilities that significantly improve productivity and efficiency.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!