Mastering Supabase Drizzle: A Comprehensive Guide to Building Modern Applications
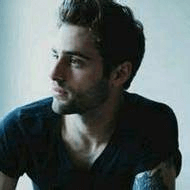
Understanding Supabase Drizzle: The Future of Database Management
Supabase Drizzle is an innovative ORM (Object-Relational Mapping) framework designed to simplify database interactions within modern applications. By streamlining the development process, it enables developers to manage data flow and interactions more effectively. In this section, we will delve into the purpose of Supabase Drizzle, its core components, and the vast array of benefits it offers for application development.
What is Supabase Drizzle?
Supabase Drizzle is a modern ORM tool that allows developers to interact with their databases efficiently. By abstracting complex SQL queries into straightforward JavaScript functions, Drizzle enables developers to concentrate on building applications rather than getting ensnared in database management tasks. For a deeper understanding of ORM, you can check this Wikipedia entry (opens in a new tab).
Core Components of Supabase Drizzle
Supabase Drizzle consists of several core components that work harmoniously to facilitate the development process:
Component | Description |
---|---|
Connection Management | Drizzle seamlessly handles connections to the Supabase database, allowing developers to focus on application logic. |
Query Building | Developers can construct complex queries using JavaScript syntax, making interactions with the database more intuitive. |
Real-time Capabilities | Drizzle's ability to manage real-time data updates allows applications to reflect changes immediately. |
These components collectively create a smooth and efficient development experience, empowering developers to build applications that are both powerful and responsive.
Benefits of Using Supabase Drizzle
Integrating Supabase Drizzle into application development enhances performance and efficiency. Key benefits include:
- Improved Development Speed: By simplifying database interactions, Drizzle allows developers to build applications faster and with fewer errors.
- Real-time Synchronization: Applications powered by Drizzle can handle live data updates, which is crucial for modern applications requiring immediate feedback.
- Seamless Integration: Supabase Drizzle integrates effortlessly with existing Supabase services, making it easy to incorporate additional functionality as required.
Potential Challenges with Supabase Drizzle
Despite its numerous advantages, developers may encounter some limitations or challenges when using Supabase Drizzle. Understanding these potential issues is essential for maximizing its effectiveness:
- Learning Curve: Developers new to the framework may require time to acclimatize to its syntax and functionality.
- Performance Bottlenecks: In certain cases, complex queries may lead to performance issues, necessitating optimization techniques.
Setting Up Your Environment for Supabase Drizzle
Before diving into application development with Supabase Drizzle, it's vital to set up your development environment correctly. This section will guide you through the prerequisites and installation steps necessary for a successful setup.
Prerequisites
To work with Supabase Drizzle, ensure that you have the following software installed:
- Node.js: The JavaScript runtime environment that allows you to run JavaScript on the server-side.
- NPM (Node Package Manager): A package manager for JavaScript that simplifies the installation of libraries and dependencies.
Installation Steps
-
Install Node.js: Download and install the latest version of Node.js from the official website (opens in a new tab).
-
Initialize a New Project:
mkdir my-supabase-app cd my-supabase-app npm init -y
-
Install Supabase Drizzle:
npm install @supabase/drizzle
-
Set Up Environment Variables: Create a
.env
file in your project root and add the necessary Supabase credentials:SUPABASE_URL=your_supabase_url SUPABASE_KEY=your_supabase_key
Best Practices for Project Structure
Organizing your project structure is crucial for maintaining code readability and manageability. A recommended structure is as follows:
my-supabase-app/
├── src/
│ ├── index.js
│ ├── models/
│ └── controllers/
├── .env
└── package.json
Troubleshooting Tips
Common setup issues may include problems with package installations or environment variable configurations. Here are some troubleshooting tips:
- Ensure that Node.js and NPM are properly installed by running
node -v
andnpm -v
. - Double-check your environment variables for any typos.
Recommended Tools
While setting up your environment, consider utilizing tools like Chat2DB (opens in a new tab). This AI-powered database management tool enhances your workflow by providing features such as natural language SQL generation and data visualization, making it easier to manage your database interactions effectively.
Building Your First Application with Supabase Drizzle
With your environment set up, you can now start building your first application using Supabase Drizzle. This section provides a step-by-step guide to creating a basic application, including code examples for key functionalities.
Setting Up Database Schema
Begin by defining your database schema. For this example, let's create a simple "users" table:
CREATE TABLE users (
id SERIAL PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(100) UNIQUE
);
Establishing a Connection
To connect your application to the Supabase database, use the following code in src/index.js
:
import { createClient } from '@supabase/supabase-js';
import { Drizzle } from '@supabase/drizzle';
const supabaseUrl = process.env.SUPABASE_URL;
const supabaseKey = process.env.SUPABASE_KEY;
const supabase = createClient(supabaseUrl, supabaseKey);
const drizzle = new Drizzle(supabase);
Implementing CRUD Operations
Now that you've set up the connection, let's implement basic CRUD operations.
Create a User
async function createUser(name, email) {
const { data, error } = await drizzle.insert('users', { name, email });
if (error) console.error('Error creating user:', error);
return data;
}
Read Users
async function readUsers() {
const { data, error } = await drizzle.select('users');
if (error) console.error('Error reading users:', error);
return data;
}
Update a User
async function updateUser(id, newName) {
const { data, error } = await drizzle.update('users', { name: newName }, { id });
if (error) console.error('Error updating user:', error);
return data;
}
Delete a User
async function deleteUser(id) {
const { data, error } = await drizzle.delete('users', { id });
if (error) console.error('Error deleting user:', error);
return data;
}
Handling Real-time Data Updates
One of the key features of Supabase Drizzle is its ability to manage real-time data updates. You can set up a listener as follows:
drizzle.on('users', (data) => {
console.log('Updated users:', data);
});
User Authentication and Authorization
To implement user authentication, you can utilize Supabase's built-in authentication features:
async function signUp(email, password) {
const { user, error } = await supabase.auth.signUp({ email, password });
if (error) console.error('Error signing up:', error);
return user;
}
async function signIn(email, password) {
const { user, error } = await supabase.auth.signIn({ email, password });
if (error) console.error('Error signing in:', error);
return user;
}
Testing and Debugging
As you build your application, it's crucial to test and debug your code. Consider using tools like Chat2DB (opens in a new tab) for enhanced database management capabilities. Its AI features can help you generate queries quickly and visualize your database structure, making testing much easier.
Advanced Features and Optimization Techniques
Once you've built a basic application with Supabase Drizzle, you may want to explore more advanced features and optimization techniques to enhance performance.
Optimizing Database Queries
To improve efficiency, consider optimizing your database queries. Use techniques such as indexing and caching to reduce latency:
CREATE INDEX idx_users_email ON users(email);
Implementing Complex Data Relationships
Drizzle allows you to manage complex data relationships. For instance, if you have a "posts" table related to the "users" table, you can set up foreign keys to maintain referential integrity.
Real-time Data Subscriptions
Utilize Drizzle's real-time capabilities to create subscriptions for data changes:
const subscription = drizzle.subscribe('users', (data) => {
console.log('Real-time update:', data);
});
Caching and Data Indexing
Implementing caching mechanisms can significantly enhance application speed. Consider using in-memory caches or Redis for rapid data access.
Analytics and Reporting
Drizzle's capabilities can be leveraged for analytics and reporting. By tracking user interactions and database changes, you can generate insightful reports to guide your development efforts.
Monitoring Application Performance
Regularly monitor your application's performance to identify bottlenecks and areas for improvement. Use profiling tools and logging to gain insights into query execution times and resource usage.
Scaling Your Application
As your application grows, you may need to scale it to handle increased data loads and user traffic. Supabase Drizzle offers features that facilitate scaling, such as load balancing and horizontal scaling strategies.
Integrating Supabase Drizzle with Existing Technologies
Integrating Supabase Drizzle with other technologies can enhance your application's capabilities. This section explores how to achieve seamless integration with popular frameworks and services.
Compatibility with Front-end Libraries
Supabase Drizzle works well with popular front-end libraries like React, Vue.js, and Angular. Integrate Drizzle into your chosen framework to manage data more effectively.
Using Supabase Drizzle with Server-side Frameworks
You can also combine Drizzle with server-side frameworks like Express or Next.js to create full-stack applications. For example, setting up an Express server with Drizzle could look like this:
const express = require('express');
const app = express();
const port = 3000;
app.get('/users', async (req, res) => {
const users = await readUsers();
res.json(users);
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
Enhancing Scalability with Cloud Services
Consider integrating Drizzle with cloud services to improve scalability and reliability. By leveraging cloud infrastructure, you can ensure that your applications remain responsive even under heavy load.
Third-party APIs and Services
Integrate Supabase Drizzle with third-party APIs to extend functionality. For example, you could connect to payment processing services or external data sources to enrich your application.
Security Considerations
When integrating with other technologies, prioritize security. Implement best practices such as data validation, encryption, and secure authentication methods to protect your application from vulnerabilities.
Data Migrations and Versioning
Managing data migrations and versioning can be challenging in a multi-service architecture. Use Drizzle's built-in capabilities to handle these tasks efficiently, ensuring that your application remains up-to-date with the latest schema changes.
Recommended Resources
For developers looking to enhance their integration capabilities, tools like Chat2DB (opens in a new tab) can be invaluable. Chat2DB's AI features allow for intuitive database management, making it easier to visualize and interact with your data.
FAQs
-
What is Supabase Drizzle?
- Supabase Drizzle is an ORM tool designed to simplify database interactions in modern applications, providing real-time data capabilities and seamless integration with Supabase services.
-
How do I set up my environment for Supabase Drizzle?
- Install Node.js and NPM, create a new project, and install the Supabase Drizzle library. Configure your environment variables for database connectivity.
-
Can I use Supabase Drizzle with front-end frameworks?
- Yes, Supabase Drizzle is compatible with popular front-end libraries such as React, Vue.js, and Angular, enabling efficient data management in your applications.
-
What are the benefits of using Supabase Drizzle?
- Key benefits include improved development speed, real-time data synchronization, and seamless integration with existing Supabase services.
-
How does Chat2DB enhance my experience with Supabase Drizzle?
- Chat2DB offers AI-powered features for natural language SQL generation and data visualization, making database management more efficient and user-friendly compared to traditional tools like DBeaver, MySQL Workbench, and DataGrip.
By leveraging the power of Supabase Drizzle and tools like Chat2DB (opens in a new tab), developers can create modern applications that are efficient, robust, and scalable.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!