How to Leverage MongoDB Realm for Efficient App Development
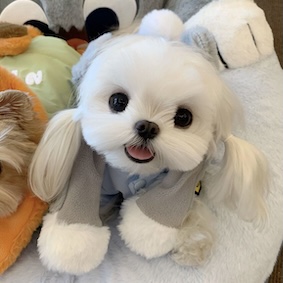
In today's fast-paced app development landscape, leveraging MongoDB Realm can significantly streamline the development process. This serverless application backend simplifies various aspects of app development, from database management through its integration with MongoDB Atlas (opens in a new tab) to ensuring real-time data synchronization and robust security features. By utilizing MongoDB Realm, developers can enjoy benefits such as rapid prototyping, offline-first capabilities, and reduced time-to-market. Furthermore, integrating tools like Chat2DB (opens in a new tab) enhances this experience by providing AI-driven database management solutions that further optimize the development workflow.
Understanding MongoDB Realm: The Serverless Solution
MongoDB Realm is designed as a serverless application backend that enables developers to focus on building applications without worrying about the complexities of traditional backend infrastructure. This powerful platform integrates seamlessly with MongoDB Atlas (opens in a new tab), which serves as the database management layer. The key benefits of using MongoDB Realm include:
Feature | Description |
---|---|
Scalability | Automatically scales with your application's needs. |
Flexibility | Allows developers to create applications with various architectures. |
Offline-first Development | Simplifies the creation of applications that can function without a constant internet connection. |
Core Components of MongoDB Realm
MongoDB Realm consists of several core components that enhance its functionality:
- Sync: Ensures real-time data synchronization across devices.
- Functions: Allows developers to run server-side logic effortlessly.
- Triggers: Automates responses to database changes.
- App Services: Provides a framework for building and deploying applications.
Robust Security Features
Security is paramount in application development. MongoDB Realm offers robust security features, including:
- Authentication: Supports multiple authentication methods such as email/password and OAuth.
- Access Control: Fine-grained permissions ensure that users only access data they should.
Ideal Applications for MongoDB Realm
MongoDB Realm is ideal for various applications, including mobile and web apps, especially those requiring real-time data access and offline capabilities.
Advantages of Using MongoDB Realm in App Development
One of the most significant advantages of using MongoDB Realm is its ability to reduce the complexity of backend development.
Serverless Architecture
The serverless architecture of MongoDB Realm eliminates the need for managing servers, allowing developers to focus more on coding and less on infrastructure.
Real-time Data Synchronization
With real-time data synchronization, applications can offer up-to-the-minute information to users, enhancing user experience significantly.
Rapid Prototyping
Developers can quickly prototype and iterate their applications, leading to faster development cycles and reduced time-to-market.
Cost-effectiveness
Using a cloud-based service like MongoDB Realm is often more cost-effective than maintaining physical servers, allowing teams to allocate resources to other critical areas.
Enhanced Productivity
The comprehensive ecosystem of MongoDB Realm improves team productivity by simplifying data access patterns and storage management.
Key Features of MongoDB Realm
Realm Sync
Realm Sync allows for real-time data synchronization across devices. This feature is crucial for applications that require consistent data availability.
Realm Functions
Realm Functions enable developers to execute backend logic directly in the cloud. Here's a simple example of a function that retrieves user data:
exports = async function(userId) {
const collection = context.services.get("mongodb-atlas").db("myDatabase").collection("users");
const user = await collection.findOne({ _id: BSON.ObjectId(userId) });
return user;
};
Triggers
Triggers in MongoDB Realm help automate responses to changes in the database. For instance, you can set up a trigger that sends an email notification every time a new user is added:
exports = async function(changeEvent) {
const userEmail = changeEvent.fullDocument.email;
const emailService = context.services.get("email-service");
await emailService.send({
to: userEmail,
subject: "Welcome!",
body: "Thank you for signing up!"
});
};
GraphQL API
The GraphQL API provides developers with flexible data querying capabilities. Here's an example of how to query users:
query {
users {
_id
name
email
}
}
Custom User Authentication
MongoDB Realm allows for custom user authentication and authorization, providing a secure way to manage user access.
Implementing MongoDB Realm in Your Projects
Initial Setup
To get started with MongoDB Realm, you need to create a Realm app and link it to a MongoDB Atlas cluster. The steps are as follows:
- Go to the MongoDB Realm (opens in a new tab) console and create a new application.
- Choose a linked MongoDB Atlas cluster.
- Set up authentication and permissions.
Configuring Realm Sync
To configure Realm Sync, follow these steps:
- In the Realm app, navigate to the Sync section.
- Enable Sync for your desired collections.
Setting Up Realm Functions
You can set up Realm Functions to handle server-side logic. Create a new function in the console and write your logic as shown above.
Implementing Triggers
Setting up triggers involves specifying the conditions under which the trigger should fire.
User Authentication
You can implement various user authentication methods, such as:
- Email/Password
- API Keys
- OAuth Providers
Using GraphQL API
To query data using the GraphQL API, you can use the provided query structure. This allows for efficient data retrieval.
Best Practices
When deploying and maintaining your MongoDB Realm applications, consider the following best practices:
- Regularly monitor performance metrics.
- Optimize data models for efficient querying.
- Implement robust security measures.
Optimizing App Performance with MongoDB Realm
Optimizing performance is crucial for enhancing user experience in applications powered by MongoDB Realm. Here are some strategies to consider:
Data Sync Performance
To optimize data synchronization, use batching techniques to reduce the number of sync operations.
Minimizing Latency
Minimize latency by leveraging caching strategies, which can significantly improve responsiveness.
Efficient Data Modeling
Efficiently modeling your data in MongoDB Realm can lead to better performance. For instance, use nested documents judiciously to keep data access fast.
Caching Strategies
Implement caching strategies to enhance offline performance. For example, store frequently accessed data in local storage.
Custom Indexes
Creating custom indexes can speed up query performance significantly. Here's an example of creating an index in MongoDB:
db.collection.createIndex({ "email": 1 });
Monitoring and Logging
Utilize monitoring and logging tools available in MongoDB Realm to track application performance and identify bottlenecks.
Scaling Applications
To handle increased user loads, scale your application using MongoDB Realm’s built-in capabilities.
Security Best Practices in MongoDB Realm
Security should be a top priority when developing applications. Here are some best practices for securing your MongoDB Realm applications:
Security Architecture
Understanding the security architecture of MongoDB Realm is crucial for maintaining a secure application.
Authentication Methods
Consider using multiple authentication methods, such as anonymous authentication for guest users, or OAuth for third-party integrations.
Access Control
Implement fine-grained access control using role-based permissions to restrict user access to sensitive data.
Data Encryption
Ensure data is encrypted both in transit and at rest to protect sensitive information.
Security Auditing
Utilize security auditing features in MongoDB Realm to monitor access and changes to your data.
Securing Backend Logic
Make sure to secure backend logic executed in Realm Functions to prevent unauthorized access.
Leveraging Chat2DB with MongoDB Realm for Enhanced Database Management
Introducing Chat2DB (opens in a new tab) as a powerful tool for enhancing database interaction and management. By integrating Chat2DB with MongoDB Realm, developers can streamline database queries and operations, leveraging features such as visual query builders and management dashboards.
AI-Driven Database Management
Chat2DB offers AI-driven functionalities that complement MongoDB Realm's capabilities. For instance, using natural language processing, developers can generate SQL queries effortlessly, making it easier to interact with data stored in MongoDB Realm.
Real-time Monitoring
With Chat2DB, users can monitor their MongoDB Realm databases in real-time, enhancing the ability to respond to issues as they arise.
Optimizing Query Performance
By utilizing Chat2DB, developers can optimize query performance and database indexing, ensuring applications run smoothly and efficiently.
Collaborative Development
Chat2DB facilitates collaborative database development and management, allowing teams to work together more effectively.
Advanced AI Features
One of the standout features of Chat2DB is its AI capabilities, which assist in generating data visualizations and performing data analysis. These features further enhance developer productivity when working with MongoDB Realm.
FAQ
-
What is MongoDB Realm?
- MongoDB Realm is a serverless application backend designed to simplify app development and integrate with MongoDB Atlas for database management.
-
How does MongoDB Realm improve app development?
- It reduces backend complexity, offers real-time data synchronization, and supports offline-first development.
-
What are the key features of MongoDB Realm?
- Key features include Realm Sync, Functions, Triggers, and a GraphQL API.
-
How can Chat2DB enhance my experience with MongoDB Realm?
- Chat2DB provides AI-driven database management, visual query builders, and real-time monitoring, enhancing productivity and efficiency.
-
Is MongoDB Realm secure for app development?
- Yes, MongoDB Realm has robust security features, including authentication, access control, and data encryption.
By leveraging the capabilities of MongoDB Realm and integrating with tools like Chat2DB (opens in a new tab), developers can significantly enhance their app development processes, ensuring they meet modern user demands while maintaining high standards of performance and security. For a more efficient and productive development experience, consider adopting Chat2DB as your go-to tool for database management.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!