How to Effectively Use db.collection.findOne() in MongoDB Queries
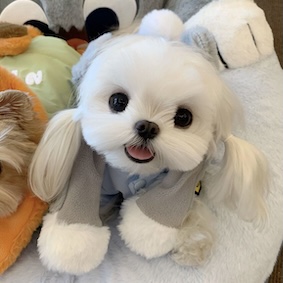
MongoDB is a powerful NoSQL database that provides developers with a flexible data model and an efficient querying system. One of the most essential methods for querying documents in MongoDB is db.collection.findOne()
. This method allows users to retrieve a single document from a collection that matches a specified query filter. In this article, we will explore how to effectively use db.collection.findOne()
in MongoDB queries, covering its syntax, optimization techniques, common use cases, error handling, and comparisons with other query methods. Additionally, we will highlight how integrating this method with tools like Chat2DB can enhance your database management experience.
Understanding db.collection.findOne() in MongoDB
The db.collection.findOne()
method is a cornerstone of MongoDB queries, designed to return a single document that matches a query filter. It is particularly useful when you expect to find one specific document or when you are only interested in retrieving a single document from a potentially larger set of matching documents. This method excels in operations requiring minimal data retrieval, making it an ideal choice for applications where performance and efficiency are key.
To illustrate, consider a MongoDB collection named "users" that contains documents representing user profiles. If you want to find a user with a specific email address, you can use db.collection.findOne()
as follows:
db.users.findOne({ email: "user@example.com" });
This query efficiently fetches the first document that matches the provided email, which is especially beneficial in applications requiring quick lookups.
Syntax and Parameters of db.collection.findOne()
To utilize db.collection.findOne()
effectively, it is crucial to understand its syntax and parameters. The basic syntax is:
db.collection.findOne(query, projection, options);
Query Parameter
The query
parameter is a filter that specifies the criteria to match documents. It uses field-value pairs to define which document to find. For example:
db.users.findOne({ username: "john_doe" });
Projection Parameter
The projection
parameter allows you to control which fields to include in the returned document. By default, all fields are returned. To return only specific fields, you can specify them as follows:
db.users.findOne({ email: "user@example.com" }, { username: 1, age: 1 });
In this example, only the username
and age
fields are returned.
Options Parameter
The options
parameter modifies the behavior of the operation. For instance, you can use options to sort the results or set a read concern. Here’s an example of using options:
db.users.findOne({ age: { $gte: 18 } }, null, { sort: { createdAt: -1 } });
This query finds the first user who is 18 years or older, sorted by their creation date in descending order.
Optimization Techniques for db.collection.findOne()
Optimizing db.collection.findOne()
queries is essential for enhancing performance, especially with large datasets. Here are some strategies:
Indexing
Creating indexes on fields used in queries can significantly improve performance. MongoDB utilizes indexes to limit the number of documents it needs to scan. For example, an index on the email
field in the "users" collection can be created as follows:
db.users.createIndex({ email: 1 });
Compound Indexes
For queries involving multiple fields, compound indexes can be beneficial. For instance, if you frequently search by both username
and email
, you can create a compound index:
db.users.createIndex({ username: 1, email: 1 });
Covering Indexes
Using covering indexes allows MongoDB to return results without accessing the actual documents, enhancing performance. Ensure that your projection includes only indexed fields to take advantage of this feature.
Limiting Fields with Projection
By limiting the fields returned using projection, you can reduce the size of the data transferred, improving query performance. For example:
db.users.findOne({ email: "user@example.com" }, { username: 1 });
Common Use Cases for db.collection.findOne()
db.collection.findOne()
is particularly useful in various scenarios:
Use Case | Example Code |
---|---|
User Authentication | db.users.findOne({ email: "user@example.com" }); |
Configuration Settings | db.configurations.findOne({ settingName: "theme" }); |
Retrieving the Latest Document | db.logs.findOne({}, { sort: { timestamp: -1 } }); |
First Match Retrieval | db.products.findOne({ sku: "12345" }); |
Handling Errors and Edge Cases with db.collection.findOne()
When using db.collection.findOne()
, it is essential to handle potential errors and edge cases effectively. Here are some considerations:
Null Results
If no matching document is found, the method returns null
. Ensure to handle this case programmatically:
const user = db.users.findOne({ email: "nonexistent@example.com" });
if (!user) {
console.log("User not found.");
}
Invalid Query Syntax
Errors may arise from invalid query syntax. Always validate your queries before execution to avoid exceptions. Use try-catch blocks to catch exceptions:
try {
const user = db.users.findOne({ email: "user@example.com" });
} catch (error) {
console.error("Query error:", error);
}
Data Consistency
For maintaining data consistency and reliability, especially in sharded clusters or replica sets, consider using transactions where necessary.
Logging and Debugging
Implement logging and debugging mechanisms to identify and resolve issues in your queries. This can help in optimizing query performance and understanding failure points.
Comparing db.collection.findOne() with Other Query Methods
Understanding the differences between db.collection.findOne()
and other MongoDB query methods can clarify when to use each effectively.
db.collection.find()
Unlike findOne()
, the find()
method returns multiple documents matching the query. It is suitable for scenarios where you expect multiple results:
db.users.find({ age: { $gte: 18 } });
db.collection.findOneAndUpdate()
The findOneAndUpdate()
method allows you to retrieve and update a document in a single operation. This method is useful when you need to modify a document while fetching its data:
db.users.findOneAndUpdate(
{ email: "user@example.com" },
{ $set: { lastLogin: new Date() } },
{ returnNewDocument: true }
);
Aggregation Framework
For complex data retrieval and transformations, the aggregation framework provides powerful capabilities. However, it is more resource-intensive compared to findOne()
, which is optimized for simple queries.
Integrating db.collection.findOne() with Chat2DB
Integrating db.collection.findOne()
with Chat2DB can significantly enhance your application's functionality. Chat2DB is an AI-powered database visualization management tool that simplifies database interactions.
Personalized User Experiences
Using findOne()
within Chat2DB can help retrieve user-specific data for personalized chat experiences. For instance, fetching user preferences quickly ensures a tailored user interaction.
Quick Lookups
You can utilize findOne()
for efficient lookups of chat history or user settings. This capability allows Chat2DB to provide swift responses, enhancing user satisfaction.
Performance Optimization
Chat2DB's features, such as connection pooling and caching mechanisms, can further optimize database interactions. Leveraging these tools can help you streamline your queries, ensuring they run efficiently.
Monitoring and Improvement
With Chat2DB, you can monitor database performance and identify areas for improvement in your queries. This monitoring ensures that your use of db.collection.findOne()
remains effective and efficient.
Frequently Asked Questions (FAQ)
-
What is the primary purpose of db.collection.findOne() in MongoDB?
- The primary purpose is to retrieve a single document that matches a specified query filter.
-
How can I optimize my queries using db.collection.findOne()?
- You can optimize queries by indexing fields, using projection to limit returned data, and employing covering indexes.
-
What happens if db.collection.findOne() does not find a matching document?
- It returns
null
if no matching document is found.
- It returns
-
Can I use db.collection.findOne() for updating documents?
- No, for updating documents while retrieving them, use
db.collection.findOneAndUpdate()
.
- No, for updating documents while retrieving them, use
-
How does Chat2DB enhance the use of db.collection.findOne()?
- Chat2DB leverages AI to optimize queries, provides quick lookups, and enhances user experiences through personalized data retrieval.
In conclusion, mastering the use of db.collection.findOne()
in MongoDB queries is essential for developers looking to optimize their database interactions. Integrating this method with tools like Chat2DB not only simplifies the querying process but also enhances overall database management efficiency. For a seamless experience and advanced AI functionalities, consider switching to Chat2DB for your database management needs.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!