How to Optimize Your Project with Spring Data MongoDB: Best Practices and Tools
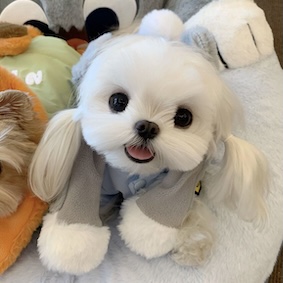
Optimizing your project with Spring Data MongoDB is essential for enhancing productivity and simplifying database interactions. This article covers foundational concepts of Spring Data MongoDB, setting up your development environment, implementing repositories, optimizing query performance, leveraging the aggregation framework, handling transactions, and integrating Chat2DB for efficient database management. By the end of this guide, you will have a comprehensive understanding of how to effectively utilize these technologies for superior application development.
Understanding Spring Data MongoDB
Spring Data MongoDB is a powerful framework that simplifies the integration of MongoDB within the Spring ecosystem. By providing a data access layer, it abstracts the complexities associated with MongoDB queries, enabling developers to focus on their application's core functionalities. One of the significant benefits of using Spring Data MongoDB is the reduction of boilerplate code, which significantly enhances developer productivity.
Spring Data MongoDB seamlessly integrates with Spring Boot, making it easier for developers to create standalone, production-grade applications. Key concepts within this framework include repositories, which provide an interface for CRUD operations, and MongoTemplate, which allows for more complex query executions and manipulations. You can learn more about these concepts in the Spring Data MongoDB documentation (opens in a new tab).
Setting Up Your Project Environment
To begin using Spring Data MongoDB, you need to set up your development environment properly. Here are the steps you should follow:
-
Install Prerequisites:
- Ensure that you have the Java Development Kit (JDK) (opens in a new tab) installed.
- Choose a build tool, either Maven (opens in a new tab) or Gradle (opens in a new tab), according to your preference.
- Set up a running instance of MongoDB (opens in a new tab).
-
Create a New Spring Boot Project:
- Use the Spring Initializr (opens in a new tab) to bootstrap a new Spring Boot project.
- Add the dependency for Spring Data MongoDB in the project configuration file (pom.xml for Maven or build.gradle for Gradle).
Here is an example for Maven:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-mongodb</artifactId> </dependency>
-
Configure Application Properties: Open your application.properties file and configure the MongoDB connection:
spring.data.mongodb.uri=mongodb://localhost:27017/yourdbname
-
Troubleshooting Tips: If you encounter issues connecting to the database, ensure that your MongoDB service is running and that the URI is correctly formatted.
Implementing Spring Data Repositories
Creating repositories in Spring Data MongoDB allows you to abstract and simplify CRUD operations. Here’s how to implement repositories:
-
Create a Repository Interface: Define an interface for your repository by extending
MongoRepository
orCrudRepository
. For example:import org.springframework.data.mongodb.repository.MongoRepository; public interface UserRepository extends MongoRepository<User, String> { User findByUsername(String username); }
-
Using @Query Annotations: You can define custom queries using the
@Query
annotation. For example:@Query("{ 'age': ?0 }") List<User> findByAge(int age);
-
Performing Common Operations: Below is a sample code snippet demonstrating common repository operations:
@Autowired private UserRepository userRepository; public void saveUser(User user) { userRepository.save(user); } public User findUserById(String id) { return userRepository.findById(id).orElse(null); } public void deleteUser(String id) { userRepository.deleteById(id); }
Optimizing Query Performance
To ensure your application remains performant, it’s crucial to optimize your queries when using Spring Data MongoDB. Here are some strategies:
-
Indexing: Indexes improve query execution speed. You can create indexes on fields frequently used in queries:
@Document(collection = "users") public class User { @Id private String id; @Indexed private String username; // other fields... }
-
Using Projections: Projections allow you to limit the data returned in queries:
public interface UserProjection { String getUsername(); }
-
Pagination and Sorting: Implement pagination and sorting to manage large datasets effectively:
Page<User> users = userRepository.findAll(PageRequest.of(0, 10));
-
Profiling Queries: Utilize MongoDB’s built-in profiling tools to identify performance bottlenecks.
Strategy | Description |
---|---|
Indexing | Improve query execution speed |
Projections | Limit the amount of data returned |
Pagination and Sorting | Manage large datasets efficiently |
Profiling Queries | Identify performance issues in your queries |
Leveraging Aggregation Framework
The aggregation framework in MongoDB is a powerful feature for processing data and generating computed results. Here’s how to use it with Spring Data MongoDB:
-
Understanding Aggregation: The aggregation framework allows you to perform operations like grouping, filtering, and sorting.
-
Using Aggregation Class: You can construct complex queries using the
Aggregation
class:Aggregation aggregation = Aggregation.newAggregation( Aggregation.match(Criteria.where("age").gt(18)), Aggregation.group("gender").count().as("total") );
-
Common Aggregation Operations: Examples of common aggregation tasks include calculating averages or summing fields:
AggregationResults<GenderCount> results = mongoTemplate.aggregate(aggregation, User.class, GenderCount.class);
-
Performance Considerations: Be mindful of the implications of using aggregations on performance. Always test and optimize your aggregation queries.
Handling Transactions in MongoDB
Transactions are crucial for maintaining data integrity. Here’s how to manage transactions in MongoDB with Spring Data:
-
Understanding ACID Transactions: ACID (Atomicity, Consistency, Isolation, Durability) transactions ensure that your operations are processed reliably.
-
Enabling Transactions: Use the
@Transactional
annotation to manage transactions effectively:@Transactional public void performTransactionalOperation(User user) { userRepository.save(user); // Other database operations... }
-
Limitations of Transactions: Be aware of the limitations of transactions in MongoDB, such as scope and performance implications.
-
Best Practices: Design your applications to minimize the need for heavy transactions, and always test transaction behaviors thoroughly.
Integrating Chat2DB for Enhanced Database Management
Integrating Chat2DB into your workflow can significantly enhance your database management experience alongside Spring Data MongoDB. Chat2DB is an AI-powered database visualization tool that supports multiple databases and offers a user-friendly interface for managing your MongoDB instances effectively.
Key Features of Chat2DB:
- Real-Time Data Monitoring: Monitor your database's performance in real-time.
- Query Execution: Execute complex queries effortlessly with an intelligent SQL editor.
- Performance Analytics: Gain insights into your database performance metrics.
- Natural Language Processing: Generate SQL queries using natural language, making it easier for non-technical users to interact with databases.
With Chat2DB, developers can streamline their development processes, making it easier to manage their MongoDB databases. The AI capabilities of Chat2DB, such as natural language query generation and intelligent SQL editing, set it apart from traditional management tools like DBeaver, MySQL Workbench, and DataGrip.
FAQ
-
What is Spring Data MongoDB? Spring Data MongoDB is a framework that simplifies the integration of MongoDB with Spring applications by providing a data access layer.
-
How do I set up a Spring Data MongoDB project? You can set up a project using Spring Initializr, adding the necessary dependencies for Spring Data MongoDB and configuring your MongoDB connection.
-
What are repositories in Spring Data MongoDB? Repositories are interfaces that provide methods for CRUD operations and custom queries, simplifying data access.
-
How can I optimize query performance in MongoDB? You can optimize performance by creating indexes, using projections, implementing pagination, and profiling your queries.
-
What advantages does Chat2DB offer for MongoDB management? Chat2DB offers AI-powered features such as natural language processing for SQL generation, real-time monitoring, and intuitive performance analytics, making it a powerful tool for database management.
By understanding and applying the concepts discussed in this article, you can optimize your projects using Spring Data MongoDB effectively and leverage tools like Chat2DB for enhanced database management.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!