How to Master MySQL Functions: A Practical Guide for Beginners
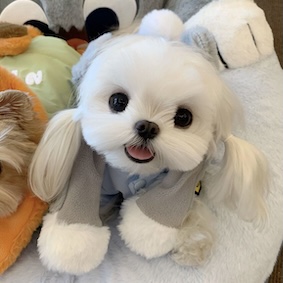
MySQL functions are essential tools in database management, enabling users to perform calculations, manipulate data, and format strings with remarkable efficiency. In this comprehensive guide, we will explore the fundamentals of MySQL functions, covering both aggregate and scalar functions. Practical examples and code snippets will illustrate each concept, enhancing your understanding of how to effectively utilize these functions. We will also delve into advanced string and date functions, custom functions, and strategies for optimizing performance. By the end of this article, you will have a solid grasp of MySQL functions and be well-prepared to integrate them into your database operations using tools like Chat2DB (opens in a new tab), which leverages AI-driven capabilities for enhanced database management.
Understanding MySQL Functions
MySQL functions are predefined routines that allow users to perform operations on data within a MySQL database. Unlike stored procedures, functions return a single value and can be directly used in SQL statements. MySQL functions can be categorized into two main types: aggregate functions and scalar functions.
Function Type | Description | Examples |
---|---|---|
Aggregate Functions | Operate on a set of values, returning a summary | COUNT() , SUM() , AVG() |
Scalar Functions | Operate on individual values, returning a single value | CONCAT() , NOW() |
Understanding the syntax and application of MySQL functions is crucial for effective database management. Functions simplify complex queries, enhance code readability, and promote efficient data manipulation.
Example of Common MySQL Functions
Here are a few common MySQL functions to illustrate their usage:
SELECT COUNT(*) AS total_rows FROM employees;
SELECT AVG(salary) AS average_salary FROM employees;
SELECT CONCAT(first_name, ' ', last_name) AS full_name FROM employees;
These examples demonstrate the efficiency with which MySQL functions can retrieve and manipulate data.
Essential Aggregate Functions
Aggregate functions are vital for summarizing and analyzing data, allowing for the collection of data points and the generation of meaningful insights. Let’s explore some key aggregate functions in detail.
COUNT()
The COUNT()
function counts the number of rows in a result set, allowing you to count all rows or specific rows based on conditions.
SELECT COUNT(*) AS total_employees FROM employees WHERE department = 'Sales';
SUM()
The SUM()
function adds values in a numeric column, providing insights into total figures.
SELECT SUM(salary) AS total_salary FROM employees WHERE department = 'Sales';
AVG()
The AVG()
function calculates the average of a numeric column, useful for understanding overall performance metrics.
SELECT AVG(salary) AS average_salary FROM employees;
MIN() and MAX()
The MIN()
and MAX()
functions are used to find the minimum and maximum values in a dataset, respectively.
SELECT MIN(salary) AS lowest_salary FROM employees;
SELECT MAX(salary) AS highest_salary FROM employees;
GROUP BY Clause
The GROUP BY
clause is often used with aggregate functions to group rows sharing a common attribute.
SELECT department, COUNT(*) AS total_employees
FROM employees
GROUP BY department;
This query will return the number of employees in each department, showcasing the power of aggregate functions in data analysis.
Scalar Functions for Data Manipulation
Scalar functions manipulate individual data elements, especially useful when dealing with string and date data types.
String Functions
- CONCAT(): Concatenates two or more strings.
SELECT CONCAT(first_name, ' ', last_name) AS full_name FROM employees;
- SUBSTRING(): Extracts a portion of a string.
SELECT SUBSTRING(first_name, 1, 3) AS short_name FROM employees;
Date Functions
- NOW(): Returns the current date and time.
SELECT NOW() AS current_time;
- DATE_FORMAT(): Formats date values according to a specified format.
SELECT DATE_FORMAT(hire_date, '%Y-%m-%d') AS formatted_hire_date FROM employees;
Mathematical Functions
- ROUND(): Rounds a number to a specified number of decimal places.
SELECT ROUND(AVG(salary), 2) AS average_salary FROM employees;
- CEIL(): Returns the smallest integer value greater than or equal to a number.
SELECT CEIL(3.14) AS rounded_value;
These scalar functions enhance the flexibility of queries, allowing for customized data manipulation and presentation.
Advanced String Functions
Advanced string functions provide greater control over text manipulation, enabling more complex data processing tasks.
REPLACE()
The REPLACE()
function replaces occurrences of a substring with another string.
SELECT REPLACE(email, '@example.com', '@newdomain.com') AS updated_email FROM employees;
INSTR()
The INSTR()
function returns the position of the first occurrence of a substring within a string.
SELECT INSTR(full_name, 'John') AS position FROM employees;
TRIM()
The TRIM()
function removes unwanted spaces from a string.
SELECT TRIM(first_name) AS trimmed_name FROM employees;
LCASE() and UCASE()
These functions convert text to lowercase and uppercase, respectively.
SELECT LCASE(first_name) AS lowercase_name FROM employees;
SELECT UCASE(last_name) AS uppercase_name FROM employees;
Combining these string functions can solve complex text processing challenges, making them invaluable for data cleansing and formatting tasks.
Utilizing Date and Time Functions
Date and time functions facilitate the management of temporal data, crucial for various business applications.
DATE_ADD() and DATE_SUB()
These functions allow users to add or subtract time intervals from a date.
SELECT DATE_ADD(hire_date, INTERVAL 1 YEAR) AS next_year_hire_date FROM employees;
SELECT DATE_SUB(hire_date, INTERVAL 1 MONTH) AS last_month_hire_date FROM employees;
DATEDIFF()
The DATEDIFF()
function calculates the difference between two dates.
SELECT DATEDIFF(NOW(), hire_date) AS days_since_hired FROM employees;
EXTRACT()
The EXTRACT()
function retrieves specific parts of a date, such as the year or month.
SELECT EXTRACT(YEAR FROM hire_date) AS hire_year FROM employees;
Using these date functions is essential for applications like scheduling and time-based analysis, especially in business scenarios.
Custom Functions with CREATE FUNCTION
Creating custom functions in MySQL allows you to encapsulate complex logic and enhance database functionality.
Syntax of CREATE FUNCTION
The syntax for defining a user-defined function (UDF) is as follows:
CREATE FUNCTION function_name(parameter1 datatype, parameter2 datatype)
RETURNS return_datatype
BEGIN
-- function logic
RETURN value;
END;
Example of a Custom Function
Here’s a simple example of a custom function that calculates the total compensation of an employee based on their salary and bonus.
CREATE FUNCTION calculate_compensation(salary DECIMAL(10,2), bonus DECIMAL(10,2))
RETURNS DECIMAL(10,2)
BEGIN
RETURN salary + bonus;
END;
Best Practices
When creating custom functions, adhere to best practices such as:
- Use descriptive names for functions.
- Document the function's purpose and parameters.
- Focus functions on a single task.
Custom functions can significantly enhance database operations, especially in cases where repetitive calculations or business-specific logic is required.
Optimizing Function Performance
To ensure that MySQL functions run efficiently, consider the following strategies for performance optimization.
Impact of Indexing
Indexing can greatly enhance the efficiency of function calls, especially for aggregate functions. By indexing the relevant columns, you can speed up data retrieval.
Query Optimization Techniques
Utilizing query optimization techniques can improve the execution of functions. Always analyze the execution plan of your queries using the EXPLAIN
statement to identify potential bottlenecks.
EXPLAIN SELECT COUNT(*) FROM employees WHERE department = 'Sales';
Efficient Function Writing
When writing functions, aim to minimize function calls within loops and avoid unnecessary complexity to reduce execution time.
Performance Monitoring Tools
Employ performance monitoring tools such as Chat2DB (opens in a new tab) to track function efficiency. Chat2DB offers AI-driven insights that can help you identify slow-performing functions and optimize them accordingly.
Integrating MySQL Functions with Chat2DB
Integrating MySQL functions with Chat2DB (opens in a new tab) enhances the management and utilization of functions within your database. Chat2DB is an AI database visualization tool designed to streamline database operations and improve productivity.
Key Features of Chat2DB
- Query Builder: Easily construct complex queries without needing extensive SQL knowledge.
- Data Visualization: Generate visual representations of data, making it easier to interpret results.
- Natural Language Processing: Utilize AI to convert natural language commands into SQL queries, simplifying database interactions.
By leveraging the capabilities of Chat2DB, you can effectively utilize MySQL functions, streamline function testing, and enhance collaboration within your development team.
Example Use Case
Imagine you want to analyze employee data using aggregate functions and visualize the results. With Chat2DB, you can easily create the necessary SQL queries, run them, and visualize the output in charts or graphs, making data analysis more intuitive.
FAQ
-
What are MySQL functions? MySQL functions are predefined routines that perform operations on data within a MySQL database, including aggregate and scalar functions.
-
What is the difference between aggregate and scalar functions? Aggregate functions perform calculations on multiple values and return a single value, while scalar functions operate on individual values.
-
How do I create a custom function in MySQL? You can create a custom function using the
CREATE FUNCTION
statement, defining parameters and return types. -
What is the role of indexing in optimizing function performance? Indexing enhances the efficiency of function calls, particularly for aggregate functions, by speeding up data retrieval.
-
How can Chat2DB help with MySQL functions? Chat2DB provides AI-driven features such as query builders and data visualization tools, making it easier to manage and utilize MySQL functions effectively.
By mastering MySQL functions and utilizing tools like Chat2DB (opens in a new tab), you can enhance your database management skills and improve your overall productivity. Switch to Chat2DB today to experience the advantages of its AI-driven features and streamline your database operations!
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!