How to Effectively Use MySQL COALESCE for Data Management
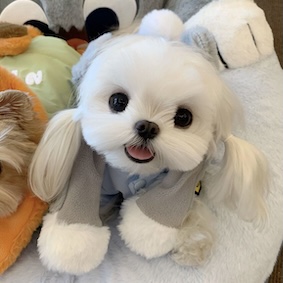
The MySQL COALESCE function is a vital tool for effective data management, particularly when dealing with NULL values in databases. This article delves into the essence of the COALESCE function, illustrating its primary role in returning the first non-NULL value from a list of expressions. We will explore its syntax, practical applications, and the benefits of using COALESCE over similar functions like IFNULL. Additionally, we will provide detailed code examples, discuss performance optimization techniques, and highlight advanced usage scenarios. Throughout the article, we will also introduce Chat2DB, an innovative AI database management tool that enhances the utilization of COALESCE in MySQL.
Understanding MySQL COALESCE
The MySQL COALESCE function serves an essential purpose in SQL queries by allowing developers to handle NULL values efficiently. It returns the first non-NULL value from a list of expressions, which is particularly useful when working with data that may not be complete. The syntax of COALESCE is straightforward:
COALESCE(expr1, expr2, ..., exprN)
This function can accept two or more arguments, making it flexible in handling multiple data sources. For example:
SELECT COALESCE(column1, column2, 'Default Value') AS result FROM my_table;
Here, if column1
is NULL, the function will return the value of column2
. If both are NULL, it will return 'Default Value'. This is particularly vital in ensuring data integrity and avoiding errors during data processing.
Handling NULL values is crucial in databases to prevent unexpected results or errors during data retrieval and manipulation. For instance, when joining tables, if one table has NULL values in a key column, it could lead to incomplete results. COALESCE simplifies SQL queries by reducing the necessity for complex conditional statements, thus enhancing readability and maintainability.
Moreover, COALESCE is compatible with various data types, making it a versatile option for developers. As an ANSI SQL standard function, it is available across different SQL databases beyond MySQL, which enhances its portability.
COALESCE vs. IFNULL: Key Differences
When it comes to handling NULL values in MySQL, developers often encounter the IFNULL function alongside COALESCE. Both functions serve similar purposes, but there are key differences that may influence a developer's choice.
Feature | COALESCE | IFNULL |
---|---|---|
Argument Count | Two or more | Exactly two |
Portability | ANSI SQL standard | MySQL-specific |
Use Cases | Complex queries | Simple NULL checks |
Performance | Slight overhead with multiple args | Efficient for two args |
- COALESCE can handle multiple arguments, while IFNULL only evaluates two expressions.
- The syntax for IFNULL is as follows:
IFNULL(expr1, expr2)
This means that if expr1
is NULL, it will return expr2
; otherwise, it returns expr1
.
In terms of performance, COALESCE may introduce slight overhead due to its ability to evaluate multiple expressions. However, this overhead is often negligible compared to the flexibility it offers. In scenarios requiring checks on more than two values, COALESCE is the preferable choice. For example:
SELECT COALESCE(column1, column2, column3, 'No Value') AS result FROM my_table;
This approach showcases COALESCE's multi-argument feature, making it beneficial for complex data handling scenarios.
Practical Applications of MySQL COALESCE
COALESCE enhances database management significantly through various real-world applications. Let’s explore some of these scenarios in detail:
1. Ensuring Data Integrity in SELECT Statements
When joining tables that may contain NULL values, COALESCE helps maintain data integrity. For instance, consider two tables: orders
and customers
. If an order does not have a corresponding customer, a NULL value will be returned. Using COALESCE, you can ensure that a default value is displayed instead:
SELECT o.order_id, COALESCE(c.customer_name, 'Unknown Customer') AS customer_name
FROM orders o
LEFT JOIN customers c ON o.customer_id = c.customer_id;
2. Providing Default Values in User Interfaces
In user interface applications, it is often necessary to display default values when data is missing. Here’s how COALESCE can facilitate this:
SELECT COALESCE(user_input, 'Default Text') AS display_text FROM user_data;
This ensures that users always see meaningful content, enhancing their experience.
3. Data Aggregation
COALESCE plays a critical role in data aggregation by substituting NULLs with meaningful values. For example, in an e-commerce platform, you may want to calculate total sales, where some sales records might be NULL:
SELECT SUM(COALESCE(sale_amount, 0)) AS total_sales FROM sales;
4. Dynamic SQL Generation
In cases where SQL queries must adapt to different conditions, COALESCE can help formulate flexible queries. Consider a scenario where a query is built dynamically based on user input:
SET @user_input = NULL;
SET @default_value = 'Fallback Value';
PREPARE stmt FROM
'SELECT COALESCE(?, ?) AS result';
EXECUTE stmt USING @user_input, @default_value;
5. Financial Databases
In financial databases, ensuring reliable reporting is crucial, especially when handling incomplete data. COALESCE can be invaluable here:
SELECT account_id, COALESCE(balance, 0) AS account_balance FROM accounts;
This guarantees that reports do not reflect NULL balances, thus providing accurate financial insights.
Chat2DB, an AI database visualization management tool, can significantly enhance the visualization and management of such queries. By integrating with MySQL, it simplifies the creation of complex queries involving COALESCE, allowing users to visualize data relationships more intuitively.
Optimizing Performance with COALESCE
While COALESCE is a powerful function, it is essential to use it wisely to maintain query performance. Here are some tips for optimizing its use:
1. Order Expressions by Likelihood
When using COALESCE with multiple arguments, order them from most likely to least likely to be non-NULL. This can minimize evaluation time:
SELECT COALESCE(column1, column2, column3) AS result FROM my_table;
2. Reduce Query Complexity
COALESCE can often simplify queries that would otherwise require nested conditional statements. This can lead to enhanced execution speed:
SELECT COALESCE(column1, 'Default Value') AS result FROM my_table WHERE column2 = 'Some Condition';
3. Indexing Considerations
Understanding how COALESCE interacts with indexing is crucial for performance. If possible, avoid using COALESCE on indexed columns, as it can prevent the optimizer from using indexes effectively.
4. Profiling SQL Queries
Regularly profile SQL queries that involve COALESCE to ensure they contribute positively to performance. Tools like Chat2DB can assist in monitoring query performance and identifying bottlenecks.
Advanced COALESCE Techniques
For seasoned developers, exploring advanced techniques with COALESCE can unlock new possibilities. Here are some sophisticated applications:
1. Nested COALESCE Functions
Nest COALESCE functions for intricate data handling strategies:
SELECT COALESCE(COALESCE(column1, column2), 'Fallback Value') AS result FROM my_table;
2. Integration with Other Functions
Combine COALESCE with other MySQL functions such as CONCAT:
SELECT CONCAT('User: ', COALESCE(username, 'Guest')) AS display_name FROM users;
3. Conditional Logic Constructs
Utilize COALESCE within conditional logic for more expressive SQL scripts:
SELECT CASE
WHEN COALESCE(column1, 0) > 0 THEN 'Positive'
ELSE 'Non-positive'
END AS status FROM my_table;
4. Stored Procedures and Triggers
Incorporate COALESCE into stored procedures and triggers for robust error handling:
CREATE TRIGGER before_insert_my_table
BEFORE INSERT ON my_table
FOR EACH ROW
SET NEW.column1 = COALESCE(NEW.column1, 'Default Value');
5. Recursive Queries
COALESCE can be beneficial in recursive queries, especially in hierarchical data structures:
WITH RECURSIVE cte AS (
SELECT id, parent_id, name
FROM my_table
WHERE parent_id IS NULL
UNION ALL
SELECT t.id, t.parent_id, COALESCE(t.name, 'Unnamed')
FROM my_table t
INNER JOIN cte ON t.parent_id = cte.id
)
SELECT * FROM cte;
Encouraging developers to experiment with COALESCE in innovative ways can enhance their MySQL expertise significantly.
Common Pitfalls and Troubleshooting
Despite its advantages, developers may encounter challenges when implementing COALESCE. Here are common pitfalls and troubleshooting tips:
1. Misunderstanding Return Types
One common mistake is misunderstanding COALESCE's return type when dealing with mixed data types. Always ensure that expressions are of compatible types.
2. Unexpected Results
If results are unexpected, check the order of expressions within COALESCE. The first non-NULL value will be returned, so the order matters.
3. Performance Issues
Improper COALESCE usage in extensive queries can lead to performance bottlenecks. Utilize tools like Chat2DB for debugging SQL scripts to gain insights into execution plans and performance metrics.
4. Testing COALESCE Implementations
Comprehensive testing is vital in complex databases. Test the implementation of COALESCE thoroughly to ensure it behaves as expected under various conditions.
5. Engaging with the Community
Engaging with the developer community can provide valuable insights into common issues and solutions related to COALESCE. Sharing experiences often leads to better practices.
Expanding Your MySQL Toolkit with Chat2DB
Chat2DB stands out as an effective tool for enhancing MySQL database management, particularly regarding COALESCE functionality. Its intuitive interface allows for easy visualization of complex SQL queries, including those utilizing COALESCE.
Key Features of Chat2DB
- AI-Powered Query Suggestions: Chat2DB leverages AI to suggest optimized queries, including those that effectively utilize COALESCE, streamlining the development process.
- Performance Monitoring: Chat2DB aids in monitoring SQL queries in real-time, enabling developers to optimize COALESCE usage effectively.
- Dynamic Query Builder: The tool simplifies the creation of complex SQL queries involving COALESCE, allowing for seamless integration into broader data management workflows.
- Collaborative Management: Chat2DB supports collaborative database management, facilitating team-based projects with shared access to COALESCE-enhanced queries.
- Community Resources: The platform offers community resources that empower developers to leverage shared knowledge and best practices in utilizing COALESCE.
For those looking to enhance their MySQL database management and leverage the power of COALESCE, adopting Chat2DB can significantly improve efficiency and productivity in data operations. Unlike traditional tools such as DBeaver, MySQL Workbench, or DataGrip, Chat2DB provides a more intuitive, AI-driven experience, making complex database management simpler and more effective.
FAQ
1. What is the primary function of MySQL COALESCE?
COALESCE returns the first non-NULL value from a list of expressions, helping manage NULL values effectively in SQL queries.
2. How does COALESCE differ from IFNULL?
COALESCE can handle multiple arguments, while IFNULL only evaluates two expressions, making COALESCE more versatile for complex queries.
3. What are some practical applications of COALESCE?
COALESCE is used in ensuring data integrity, providing default values in user interfaces, data aggregation, and dynamic SQL generation.
4. How can I optimize my queries using COALESCE?
To optimize queries, order COALESCE expressions by likelihood of being non-NULL, and consider indexing strategies to maintain performance.
5. How does Chat2DB enhance the use of COALESCE?
Chat2DB provides an intuitive interface for visualizing queries, real-time performance monitoring, and collaborative features that streamline the use of COALESCE in data management.
For a deeper understanding and practical experience with COALESCE and MySQL, consider exploring Chat2DB, which can elevate your database management capabilities significantly.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!