PL/SQL vs Oracle SQL: Understanding Their Distinct Roles and Functionalities
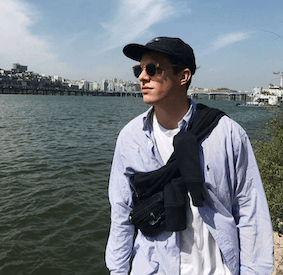
Understanding PL/SQL and Oracle SQL
PL/SQL and Oracle SQL are two essential components of the Oracle Database ecosystem, each serving distinct purposes. Oracle SQL is a standard language used for querying and manipulating data, while PL/SQL extends SQL by adding procedural capabilities. This combination allows developers to perform complex logical operations and control structures that are not possible with SQL alone.
PL/SQL (Procedural Language/SQL) integrates seamlessly with SQL, enabling developers to execute SQL statements within PL/SQL blocks. This integration is crucial for scenarios requiring both data manipulation and business logic execution. Unlike SQL, which has a declarative nature focused solely on data retrieval and management, PL/SQL follows a block structure that includes declarations, executable statements, and exception handling.
Here’s a brief comparison of their syntax:
-- Oracle SQL Example
SELECT employee_id, first_name
FROM employees
WHERE department_id = 10;
-- PL/SQL Example
DECLARE
v_employee_id employees.employee_id%TYPE;
BEGIN
SELECT employee_id INTO v_employee_id
FROM employees
WHERE department_id = 10;
DBMS_OUTPUT.PUT_LINE('Employee ID: ' || v_employee_id);
END;
In the SQL example, the focus is on querying data, while the PL/SQL example demonstrates how to handle data within a procedural block. Understanding these differences is critical for selecting the right tool for specific tasks in database management.
Core Features and Capabilities
PL/SQL offers several core features that enhance its utility for developers:
-
Exception Handling: PL/SQL allows developers to manage errors gracefully using exception handling mechanisms. This capability ensures that applications can continue functioning even when unexpected issues arise.
-
Modular Programming: Developers can create procedures, functions, and packages in PL/SQL, promoting code reusability and organization. This modular approach helps in maintaining large codebases.
-
Data Encapsulation: PL/SQL supports encapsulation, enabling developers to hide data and methods within packages. This feature enhances security and reduces the risk of unauthorized access.
On the other hand, Oracle SQL provides advanced querying capabilities:
-
Joins and Subqueries: Oracle SQL efficiently handles complex queries involving multiple tables through joins and subqueries, which are fundamental for data retrieval in relational databases.
-
Analytical Functions: SQL includes analytical functions that allow for sophisticated data analysis, such as running totals and moving averages, critical for reporting and business intelligence.
-
Data Manipulation: SQL facilitates insert, update, delete, and merge operations, which are essential for maintaining data integrity and performing batch operations.
Here’s a simple example of an update operation in SQL:
UPDATE employees
SET salary = salary * 1.1
WHERE department_id = 10;
In contrast, PL/SQL can execute complex business logic using loops and conditional statements. For instance:
DECLARE
CURSOR emp_cursor IS SELECT employee_id FROM employees WHERE department_id = 10;
v_employee_id employees.employee_id%TYPE;
BEGIN
FOR emp_record IN emp_cursor LOOP
v_employee_id := emp_record.employee_id;
-- Perform additional logic here
END LOOP;
END;
This structure demonstrates how PL/SQL can iterate through records and apply business rules, showcasing its procedural strengths.
Performance Considerations
When choosing between PL/SQL and Oracle SQL, performance is a crucial factor. PL/SQL’s procedural nature allows developers to optimize code to reduce network traffic by bundling multiple SQL statements into a single PL/SQL block. This optimization is essential in scenarios where round-trip delays between the application and the database can hinder performance.
However, developers must also be aware of the impact of context switching between PL/SQL and SQL. Each switch can introduce execution delays; therefore, minimizing these transitions is vital for performance. In scenarios that require large data processing, PL/SQL’s capabilities for batch processing can significantly enhance efficiency.
Moreover, SQL's set-based operations often outperform PL/SQL’s procedural approach in certain scenarios. For instance, executing a single SQL statement to update multiple records is generally faster than processing each record individually in PL/SQL.
Here’s an example of performance optimization in PL/SQL using bulk operations:
DECLARE
TYPE emp_record_type IS TABLE OF employees%ROWTYPE;
v_emp_records emp_record_type;
BEGIN
SELECT * BULK COLLECT INTO v_emp_records FROM employees WHERE department_id = 10;
-- Process records in v_emp_records
END;
This bulk collect statement retrieves multiple rows in a single operation, minimizing context switches and improving performance.
Use Cases and Applications
PL/SQL is often preferred in scenarios requiring complex algorithms and data processing workflows. For example, financial institutions may use PL/SQL to implement intricate business logic for transaction processing, ensuring accuracy and compliance with regulations.
In contrast, Oracle SQL excels in reporting and analytics applications where quick data retrieval is crucial. For instance, a business intelligence dashboard may rely on SQL queries to fetch data in real-time for analysis and reporting.
PL/SQL enhances data validation and cleanup processes by leveraging its procedural capabilities. For instance, during ETL (Extract, Transform, Load) processes, PL/SQL can validate data formats and perform necessary transformations before loading the data into target tables.
Additionally, PL/SQL is widely used for automating database tasks and scheduling jobs. For instance, organizations can schedule PL/SQL procedures to run at specific intervals, performing routine maintenance and reporting tasks without manual intervention.
Oracle SQL, on the other hand, is prevalent in data warehousing environments, where it manages large datasets efficiently. It handles complex queries that analyze historical data, providing insights into business performance.
Integrating Chat2DB with PL/SQL and Oracle SQL enhances database management by providing a user-friendly interface for executing queries and managing database objects. Chat2DB streamlines the development process, enabling faster and more efficient database interactions.
Development and Debugging
Developers can utilize various tools and environments for PL/SQL and Oracle SQL development, with Oracle SQL Developer and Chat2DB being among the most popular. These tools provide features such as code completion, syntax highlighting, and debugging capabilities, making the development process more efficient.
In PL/SQL, debugging features include the use of DBMS_OUTPUT
for tracing errors and monitoring program execution. Developers can insert output statements to track variable values and flow control, helping identify issues during execution.
Best practices for writing maintainable and efficient PL/SQL code include:
- Modularizing code into procedures and functions.
- Documenting code for better understanding.
- Using meaningful variable names to enhance readability.
- Implementing exception handling to manage errors effectively.
Testing and validating SQL queries are equally important to ensure accuracy and performance. Developers should regularly review and optimize their SQL queries, considering factors such as execution plans and indexing strategies.
Version control systems play a critical role in managing changes to PL/SQL and SQL code. Utilizing tools like Git enables teams to collaborate effectively, track changes, and maintain code integrity. Additionally, integrating CI/CD (Continuous Integration/Continuous Deployment) pipelines into database development workflows can automate testing and deployment processes, ensuring code reliability.
Automated testing frameworks for PL/SQL and SQL further enhance code reliability by allowing developers to create test cases and validate functionality before deployment.
Advanced Topics and Future Trends
Delving into advanced PL/SQL features reveals capabilities such as dynamic SQL, which allows developers to construct and execute SQL statements at runtime. This flexibility is particularly useful in scenarios where SQL statements need to be generated based on user input or other dynamic conditions.
Autonomous transactions in PL/SQL enable developers to execute independent transactions within a larger transaction, providing greater control over error handling and rollback mechanisms.
Pipelined table functions are another advanced feature that allows for processing large data sets in a more efficient manner. By returning rows one at a time, these functions can optimize resource utilization.
On the other hand, Oracle SQL is evolving with emerging features such as JSON data handling and machine learning capabilities. These advancements open up new possibilities for data analysis and application development.
In cloud environments, the role of PL/SQL is adapting to Oracle Cloud Infrastructure, providing developers with the tools needed to build scalable and efficient applications. The integration of PL/SQL with modern application development frameworks, such as microservices and serverless computing, is becoming increasingly relevant.
Artificial intelligence and machine learning are also influencing PL/SQL and SQL development, offering new ways to analyze data and automate decision-making processes. Developers must stay updated with the latest trends and best practices to leverage these technologies effectively.
Conclusion
In summary, understanding the distinct roles and functionalities of PL/SQL and Oracle SQL is essential for effective database management. Both languages offer unique capabilities that cater to different use cases and requirements. Whether you're looking to perform complex business logic with PL/SQL or execute efficient data retrieval with Oracle SQL, having a solid grasp of these tools will enhance your effectiveness as a database developer.
For further learning and exploration of database management, consider using Chat2DB, which provides a user-friendly interface and powerful features to streamline your development process and enhance your productivity.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!