Use SQL Aliases to Simplify Your Queries
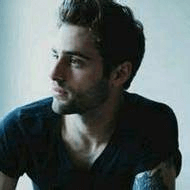
Unlocking the Power of SQL Aliases for Enhanced Query Optimization
When it comes to optimizing your SQL queries, SQL aliases are essential tools that can dramatically improve the readability and manageability of your code. By allowing developers to assign temporary names to tables and columns, SQL aliases simplify complex queries, enhance performance, and clarify the intent behind SQL statements. In this guide, we will delve into the significance of SQL aliases, provide detailed code examples, and demonstrate how tools like Chat2DB can leverage these concepts for superior query management.
What Are SQL Aliases?
SQL aliases serve as temporary names for tables or columns, making it easier to work with complex queries, especially when multiple tables are involved. Consider the following SQL query using no aliases:
SELECT Employees.EmployeeID, Employees.FirstName, Employees.LastName, SUM(Orders.TotalAmount) AS TotalSales
FROM Employees
JOIN Orders ON Employees.EmployeeID = Orders.EmployeeID
GROUP BY Employees.EmployeeID, Employees.FirstName, Employees.LastName;
This query can be simplified and made more readable with aliases:
SELECT e.EmployeeID, e.FirstName, e.LastName, SUM(o.TotalAmount) AS TotalSales
FROM Employees AS e
JOIN Orders AS o ON e.EmployeeID = o.EmployeeID
GROUP BY e.EmployeeID, e.FirstName, e.LastName;
By using e
for Employees
and o
for Orders
, the query becomes easier to read and manage, especially as the complexity of the SQL statement increases.
Enhancing Readability and Manageability
Utilizing SQL aliases goes beyond mere aesthetics; they significantly improve the maintainability of your SQL scripts. In collaborative projects, using consistent and meaningful aliases fosters better communication among developers. Clear aliases enhance productivity and reduce the risk of errors.
Aliases also play a crucial role in joining tables, especially when similar column names exist across multiple tables. By clarifying the source of each column, aliases reduce ambiguity, which is vital in queries involving multiple joins.
Simplifying Nested Queries and Subqueries
Nested queries and subqueries can quickly become intricate, hindering clarity. Here’s an example of a nested query without aliases:
SELECT EmployeeID, FirstName, LastName
FROM Employees
WHERE EmployeeID IN (SELECT EmployeeID FROM Orders WHERE TotalAmount > 1000);
Introducing aliases makes the structure clearer:
SELECT e.EmployeeID, e.FirstName, e.LastName
FROM Employees AS e
WHERE e.EmployeeID IN (SELECT o.EmployeeID FROM Orders AS o WHERE o.TotalAmount > 1000);
Using aliases not only simplifies the query but also clarifies the relationship between the tables involved.
Practical Use Cases for SQL Aliases
Streamlining Query Operations
When faced with lengthy table names, aliases provide clarity and conciseness. Consider this verbose query:
SELECT `CustomerOrders`.`OrderID`, `CustomerOrders`.`CustomerName`
FROM `CustomerOrders`
WHERE `CustomerOrders`.`OrderDate` > '2023-01-01';
With an alias, the query can be streamlined:
SELECT co.OrderID, co.CustomerName
FROM CustomerOrders AS co
WHERE co.OrderDate > '2023-01-01';
Renaming Output Columns for Clarity
Column aliases allow for renaming output columns, enhancing clarity in results. For example:
SELECT SUM(TotalAmount) AS TotalSales
FROM Orders;
Here, the alias TotalSales
clearly indicates what the output represents.
Using Aliases in Self-Joins
Self-joins can be confusing without proper aliasing. For comparing employees with their managers, consider this query:
SELECT e1.EmployeeID AS EmployeeID, e2.FirstName AS ManagerName
FROM Employees AS e1
JOIN Employees AS e2 ON e1.ManagerID = e2.EmployeeID;
The aliases e1
and e2
clarify the two instances of the Employees
table.
Improving Reports and Dashboards
Aliases assist in creating understandable reports and dashboards. By providing meaningful labels, you make it easier for end-users to interpret data. For instance:
SELECT SUM(TotalAmount) AS TotalSales, COUNT(OrderID) AS TotalOrders
FROM Orders;
Using aliases like TotalSales
and TotalOrders
conveys the necessary information directly.
Real-World Example: Aggregations with Aliases
In aggregate functions, aliases simplify the interpretation of results. For example:
SELECT ProductID, COUNT(OrderID) AS OrderCount
FROM Orders
GROUP BY ProductID;
The alias OrderCount
allows users to quickly understand the output, enhancing usability.
Query Type | Example Query | Benefits |
---|---|---|
Basic Select | SELECT e.EmployeeID, e.FirstName FROM Employees AS e; | Simplifies table reference |
Nested Query | SELECT e.EmployeeID FROM Employees AS e WHERE e.EmployeeID IN (SELECT o.EmployeeID FROM Orders AS o); | Clarifies relationships |
Self-Join | SELECT e1.FirstName AS Employee, e2.FirstName AS Manager FROM Employees AS e1 JOIN Employees AS e2; | Distinguishes roles |
Aggregation | SELECT ProductID, COUNT(OrderID) AS OrderCount FROM Orders GROUP BY ProductID; | Enhances output clarity |
Advanced Techniques with SQL Aliases
Using Aliases with Window Functions
Aliases truly shine when paired with window functions. For instance, calculating a moving average becomes clearer with aliases:
SELECT OrderID,
TotalAmount,
AVG(TotalAmount) OVER (ORDER BY OrderDate ROWS BETWEEN 4 PRECEDING AND CURRENT ROW) AS MovingAverage
FROM Orders;
Here, MovingAverage
is an alias that simplifies understanding the calculation.
Common Table Expressions (CTEs) and Aliases
CTEs help break down complex queries, and aliases are key for clarity. For example:
WITH SalesCTE AS (
SELECT ProductID, SUM(TotalAmount) AS TotalSales
FROM Orders
GROUP BY ProductID
)
SELECT * FROM SalesCTE;
The alias SalesCTE
provides a clear reference to the dataset being queried.
Conditional Expressions and Case Statements
Aliases can enhance conditional expressions and case statements. For example:
SELECT
ProductID,
CASE
WHEN TotalAmount > 1000 THEN 'High'
ELSE 'Low'
END AS SalesCategory
FROM Orders;
The alias SalesCategory
makes the output immediately understandable.
Optimizing UNION Operations with Aliases
In UNION operations, aliases help label the combined result set. Consider:
SELECT ProductID, 'Sales' AS Source FROM Sales
UNION ALL
SELECT ProductID, 'Returns' AS Source FROM Returns;
The Source
alias clarifies the origin of each record.
Simplifying Recursive Queries with Aliases
Recursive queries can be complex, but aliases simplify the process. For example:
WITH RECURSIVE EmployeeHierarchy AS (
SELECT EmployeeID, ManagerID, FirstName, LastName
FROM Employees
WHERE ManagerID IS NULL
UNION ALL
SELECT e.EmployeeID, e.ManagerID, e.FirstName, e.LastName
FROM Employees AS e
INNER JOIN EmployeeHierarchy AS eh ON e.ManagerID = eh.EmployeeID
)
SELECT * FROM EmployeeHierarchy;
The use of aliases in this recursive query helps clarify relationships between employees and their managers.
Common Pitfalls and Best Practices in Using SQL Aliases
Avoiding Reserved Keywords
A common mistake is using reserved keywords as aliases. For example, using Order
might lead to confusion. Always check SQL standards for reserved keywords.
Consistent Naming Conventions
Establish consistent naming conventions for aliases to maintain readability. For instance, using a prefix like t_
for table aliases can help distinguish them from column aliases.
Preventing Ambiguity
When using multiple aliases, it’s essential to avoid ambiguity. Clearly define each alias and its associated table or column to prevent confusion.
Documenting Aliases
In collaborative environments, documenting aliases in SQL scripts is vital. This aids other developers in understanding the purpose of each alias and facilitates easier maintenance.
Ensuring Backward Compatibility
As databases evolve, maintaining backward compatibility is essential. Meaningful aliases help ensure that legacy queries remain understandable and functional.
Harnessing the Power of SQL Aliases with Chat2DB for Enhanced Query Management
Chat2DB is an innovative AI-powered database visualization tool that seamlessly integrates SQL aliases to enhance query management. By simplifying the application of aliases, Chat2DB allows developers to construct complex queries without sacrificing clarity.
Key Features of Chat2DB
- Natural Language Processing: Chat2DB utilizes AI to convert natural language into SQL queries, enabling users to generate queries quickly and accurately.
- Smart SQL Editor: The smart SQL editor in Chat2DB supports aliasing, offering real-time suggestions and helping developers avoid common pitfalls.
- Visual Query Builder: The visual query builder facilitates the creation of complex queries, demonstrating how aliases simplify query construction.
- Automated Feedback: Chat2DB provides immediate feedback on alias usage, aiding developers in optimizing their queries and improving performance.
Case Studies Illustrating Chat2DB's Capabilities
Teams that have adopted Chat2DB's aliasing capabilities report improved database performance and increased developer efficiency. By streamlining the query-building process, Chat2DB allows developers to focus on logic rather than syntax.
Community Feedback
Users praise Chat2DB for its user-friendly interface, particularly how its AI features make managing SQL aliases seamless. Many have noted significant reductions in time spent on query optimization.
By choosing Chat2DB, developers can effectively leverage SQL aliases to enhance both query performance and collaboration across teams. For more information, explore Chat2DB (opens in a new tab).
Frequently Asked Questions (FAQ)
-
What are SQL aliases? SQL aliases are temporary names assigned to tables or columns in SQL queries, enhancing readability and manageability.
-
Why should I use SQL aliases? SQL aliases improve query clarity, reduce ambiguity, and foster collaboration among developers.
-
How do SQL aliases improve performance? While aliases do not directly enhance performance, they simplify complex queries, leading to more efficient execution plans.
-
Can I use SQL aliases in nested queries? Yes, using aliases in nested queries simplifies the structure and enhances readability.
-
What tools can help with SQL alias management? Consider using Chat2DB, an AI-powered tool that streamlines database management and enhances the use of SQL aliases.
By mastering SQL aliases, you can significantly enhance your query optimization, making your SQL development more efficient and collaborative.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!