How to Effectively Use SQL Update with Aliases and Subqueries
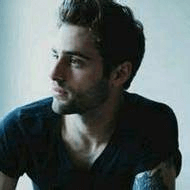
Understanding the Essentials of SQL Update Statements
SQL Update statements are fundamental for modifying existing records within a database. They empower users to change data in one or more columns of a table based on specific criteria. The core structure of an SQL Update statement consists of the SET clause, which specifies the columns to be updated, and the WHERE clause, which identifies the records that should be modified.
Syntax Breakdown
Here’s a basic syntax example of an SQL Update statement:
UPDATE table_name
SET column1 = value1, column2 = value2
WHERE condition;
In this example, table_name
represents the table you want to update, while column1
and column2
are the columns where you intend to set new values. The WHERE
clause is crucial; without it, all records in the table would be updated, potentially leading to unintended consequences.
Common Pitfalls
One frequent mistake is omitting the WHERE clause, which can result in all rows being updated. For instance:
UPDATE employees
SET salary = salary * 1.10; -- This updates every row in the employees table
Instead, a proper approach would be:
UPDATE employees
SET salary = salary * 1.10
WHERE department = 'Sales'; -- This only updates the sales department
Before executing updates on a production database, it’s prudent to test your SQL statements in a safe environment to ensure they behave as expected.
The Role and Benefits of Using Aliases in SQL
Aliases in SQL provide temporary names for tables or columns, enhancing readability and simplifying complex queries. By leveraging aliases, you can create more manageable SQL statements, especially when dealing with multiple tables.
Benefits of Using Aliases
Benefit | Description |
---|---|
Increased Readability | Aliases make queries easier to read and understand. |
Reduced Complexity | Simplifies complex joins and subqueries. |
Enhanced Maintainability | Easier to modify queries as table structures change. |
For example:
SELECT e.first_name AS 'First Name', e.last_name AS 'Last Name'
FROM employees AS e
JOIN departments AS d ON e.department_id = d.id;
In this query, e
and d
serve as aliases for the employees
and departments
tables, respectively.
Common Syntax Errors
When using aliases, it’s essential to avoid common syntax errors. For instance, forgetting to use the AS
keyword can lead to confusion. The correct approach is:
SELECT e.first_name AS 'First Name' FROM employees AS e;
This ensures clarity in your queries and prevents syntax-related issues.
Harnessing the Power of Subqueries in SQL Update Statements
Subqueries are powerful tools that allow you to nest queries within other queries. They can provide dynamic data for updates, enabling complex data transformations that would be challenging to achieve with straightforward SQL statements.
Types of Subqueries
- Scalar Subqueries: Return a single value.
- Column Subqueries: Return a single column of values.
- Row Subqueries: Return a single row of values.
- Table Subqueries: Return multiple rows and columns.
Using Subqueries in Update Statements
Incorporating subqueries into SQL Update statements can enhance their functionality. For example, consider the following scenario where you want to update salaries based on the average salary of a department:
UPDATE employees
SET salary = salary * 1.10
WHERE department_id IN (
SELECT id
FROM departments
WHERE name = 'Sales'
);
In this example, the subquery retrieves the department ID for 'Sales', and only employees in this department receive a salary increase.
Performance Considerations
While subqueries are powerful, they can affect performance if not used judiciously. Ensure that subquery results are compatible with the main query's update logic. Testing subqueries in a development environment can help identify performance bottlenecks before deployment.
Step-by-Step Guide to Combining SQL Update with Aliases and Subqueries
Combining SQL Update with aliases and subqueries can streamline your database operations. Here’s a step-by-step guide to effectively implementing these techniques.
Step 1: Identify Update Requirements
Begin by determining what data needs to be updated and the source from which this data will come. For instance, you may need to update customer records based on changes in their order status.
Step 2: Define Aliases for Tables and Columns
Using aliases can simplify your SQL statements:
UPDATE c
SET c.status = 'Shipped'
FROM customers AS c
WHERE c.id IN (
SELECT o.customer_id
FROM orders AS o
WHERE o.status = 'Processed'
);
In this example, c
and o
serve as aliases for the customers
and orders
tables, respectively.
Step 3: Construct Subqueries
Subqueries can dynamically fetch data required for the update. Ensure you use the correct syntax and that the subquery returns the expected results.
Step 4: Nest Subqueries Properly
Nesting subqueries within the Update statement must be done carefully to maintain clarity:
UPDATE products AS p
SET p.price = p.price * 1.05
WHERE p.id IN (
SELECT o.product_id
FROM orders AS o
WHERE o.order_date > '2023-01-01'
);
Step 5: Testing Updates
Before applying changes to the production database, use tools like Chat2DB to simulate your updates. Chat2DB provides an intuitive interface for testing SQL queries, allowing you to validate your updates in a safe environment.
Step 6: Backup Your Database
Always maintain a backup of your database before executing significant updates. This precaution ensures that you can restore the original data if something goes wrong.
Best Practices and Common Pitfalls
When using SQL Update with aliases and subqueries, adhering to best practices is crucial for maintaining data integrity and performance.
Best Practices
- Write Clear Queries: Use meaningful aliases that describe the data being referenced.
- Understand Your Data Model: Familiarize yourself with the relationships between tables to construct effective subqueries.
- Optimize Performance: Avoid overly complex subqueries that could degrade performance. Instead, consider using joins where appropriate.
Common Pitfalls to Avoid
- Incorrect Join Conditions: Ensure that your join conditions are accurate to prevent unintended updates.
- Overlooked Null Values: Be mindful of null values in your data, as they can lead to unexpected results in updates.
Using tools like Chat2DB can help you identify and rectify these issues before executing your queries.
Exploring Real-World Scenarios and Use Cases
SQL Update with aliases and subqueries finds application across diverse industries. Here are some real-world scenarios where these techniques can be beneficial.
Mass Data Updates in E-commerce
In e-commerce databases, you may need to update product prices based on seasonal promotions. A combined SQL Update with subqueries can facilitate this process efficiently.
UPDATE products AS p
SET p.price = p.price * 0.90
WHERE p.id IN (
SELECT o.product_id
FROM orders AS o
WHERE o.order_date BETWEEN '2023-11-01' AND '2023-11-30'
);
Data Migration Projects
During data migration, complex transformations are often required. Using SQL Update with subqueries and aliases can streamline this process, ensuring data consistency across systems.
Data Cleaning Processes
SQL Update plays a crucial role in data cleaning, such as standardizing inconsistent entries in databases. For instance:
UPDATE customers AS c
SET c.email = LOWER(c.email)
WHERE c.email IS NOT NULL;
Business Intelligence Updates
In business intelligence applications, maintaining analytical datasets is vital. Updating these datasets accurately is essential for reliable reporting.
FAQs
Q1: What is the purpose of SQL Update statements?
A1: SQL Update statements are used to modify existing records in a database, allowing you to change data in specific columns based on defined conditions.
Q2: What are aliases in SQL?
A2: Aliases are temporary names assigned to tables or columns in SQL, enhancing query readability and simplifying complex operations.
Q3: How do subqueries work in SQL Update statements?
A3: Subqueries allow you to nest queries within other queries, providing dynamic data for updates and enabling complex data transformations.
Q4: What are some best practices when using SQL Update?
A4: Best practices include writing clear queries, understanding your data model, and using tools like Chat2DB for testing and validation.
Q5: How can Chat2DB assist with SQL updates?
A5: Chat2DB is an AI-driven database management tool that simplifies SQL query testing and validation. With its advanced natural language processing features, it allows users to generate SQL queries effortlessly, enhancing productivity compared to traditional tools like DBeaver, MySQL Workbench, and DataGrip.
In conclusion, for a more streamlined and efficient database management experience, consider transitioning to Chat2DB, which not only simplifies SQL updates but also integrates various intelligent features that significantly enhance productivity and ease of use.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!