SQL Native Client: A Comprehensive Guide for Developers
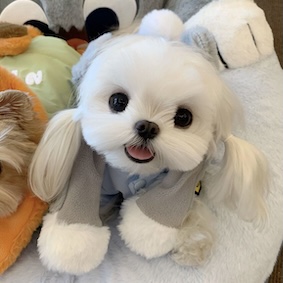
Introduction
SQL Native Client is an essential data access technology for developers working with SQL Server. It plays a critical role in facilitating communication between applications and SQL Server databases. Understanding SQL Native Client helps developers optimize their applications and improve data access performance. This article outlines the key features, installation guidelines, and best practices for using SQL Native Client effectively.
Before diving into the specifics, it is crucial to define some essential terms. OLE DB (Object Linking and Embedding Database), ODBC (Open Database Connectivity), and ADO.NET (ActiveX Data Objects for .NET) are integral to understanding how SQL Native Client operates. SQL Native Client combines the features of both OLE DB and ODBC, offering a robust solution for accessing SQL Server data.
The evolution of data access technologies for SQL Server has seen significant advancements, which have streamlined the development process. Chat2DB is a valuable tool that integrates with SQL Native Client, providing an enhanced user experience for database management and development.
Understanding SQL Native Client
SQL Native Client is a data access technology specifically designed for SQL Server. It offers a unique combination of OLE DB and ODBC features, making it a versatile choice for developers. The primary role of SQL Native Client is to enable applications to access SQL Server data with enhanced performance and security.
One of the significant advantages of using SQL Native Client is its ability to integrate seamlessly with existing data access architectures. This integration allows developers to leverage the advanced capabilities of SQL Server while maintaining compatibility with traditional data access methods.
SQL Native Client is particularly beneficial in scenarios where performance and security are paramount. For example, applications that require high data throughput or handle sensitive information can greatly benefit from using SQL Native Client over traditional OLE DB or ODBC providers. It is compatible with various versions of SQL Server, making it a reliable choice for diverse development environments.
Key Features of SQL Native Client
Support for Native SQL Server Data Types
SQL Native Client provides support for native SQL Server data types. This support allows developers to work with complex data structures more efficiently, improving the overall application performance. By using native data types, applications can minimize data conversion overhead and ensure optimal data handling.
Advanced Connection Pooling
Connection pooling is a crucial feature for improving application performance. SQL Native Client supports advanced connection pooling capabilities, allowing multiple connections to be reused, reducing the overhead of establishing new connections. This enhancement can significantly speed up data access in high-traffic applications.
Multiple Active Result Sets (MARS)
MARS is a feature that enables multiple active result sets on a single connection. This capability allows developers to execute multiple queries simultaneously without the need for additional connections. It enhances data retrieval efficiency, making it easier to handle complex data access scenarios.
Integration of SQL Server Security Features
Security is a top priority for any application accessing sensitive data. SQL Native Client integrates SQL Server's security features, such as encryption and authentication, ensuring that data remains secure during transmission. This integration is vital for applications that handle confidential information or operate in regulated environments.
Support for XML Data Types
The support for XML data types in SQL Native Client is beneficial for modern applications that rely on XML for data interchange. This feature allows developers to work with XML data seamlessly, enabling efficient data manipulation and storage.
Bulk Copy Program (BCP) Enhancements
SQL Native Client enhances the Bulk Copy Program (BCP), which is a utility for importing and exporting large volumes of data. These enhancements improve the performance and usability of BCP, making it easier for developers to manage large data sets efficiently.
Asynchronous Data Access
Asynchronous data access is crucial for building responsive applications. SQL Native Client supports asynchronous operations, allowing applications to continue processing while waiting for data retrieval. This feature is essential for improving user experience in data-intensive applications.
Installation and Configuration Guide
Step-by-Step Installation Guide
Installing SQL Native Client is a straightforward process. Follow these steps to install it on different operating systems:
- Download SQL Native Client: Visit the Microsoft website to download the latest version of SQL Native Client.
- Run the Installer: Double-click the downloaded file and follow the installation prompts.
- Accept the License Agreement: Read and accept the license terms to proceed.
- Select Installation Type: Choose the appropriate installation type (e.g., typical or custom).
- Complete Installation: Follow the remaining prompts to complete the installation.
Prerequisites and Software Requirements
Before installing SQL Native Client, ensure that your system meets the following prerequisites:
- A compatible version of Windows or SQL Server.
- Sufficient disk space for installation.
- Administrator privileges to install software.
Configuration Process
After installation, configure ODBC and OLE DB connections using SQL Native Client:
-
ODBC Configuration:
- Open the ODBC Data Source Administrator.
- Select the 'System DSN' tab and click 'Add'.
- Choose 'SQL Server Native Client' from the list and click 'Finish'.
- Enter the necessary connection details (e.g., server name, database name).
- Test the connection and save the configuration.
-
OLE DB Configuration:
- Open the relevant application (e.g., Visual Studio).
- Navigate to the connection properties and select 'SQL Native Client' as the data source.
- Enter the required credentials and connection details.
Troubleshooting Tips
If you encounter issues during installation, consider the following troubleshooting tips:
- Ensure that your system meets the software requirements.
- Check for any conflicting software that may interfere with the installation.
- Review the installation logs for detailed error information.
Verifying Installation
To verify a successful installation, perform the following steps:
- Open the ODBC Data Source Administrator and check for the SQL Native Client listed under the 'Drivers' tab.
- Test the configured connections to ensure they are working correctly.
Upgrading SQL Native Client
When upgrading from previous versions of SQL Native Client, follow these steps:
- Uninstall the older version from your system.
- Download the latest version from the Microsoft website.
- Follow the installation steps outlined earlier.
Keeping SQL Native Client updated is crucial for maintaining security and performance.
SQL Native Client in Application Development
SQL Native Client can be seamlessly integrated into various programming languages for application development. Let's explore how it works with different technologies.
ADO.NET Integration
In .NET applications, SQL Native Client can be easily integrated using ADO.NET. Here is a sample code snippet demonstrating how to connect to a SQL Server database using ADO.NET with SQL Native Client:
using System;
using System.Data.SqlClient;
class Program
{
static void Main()
{
string connectionString = "Server=myServerAddress;Database=myDataBase;User Id=myUsername;Password=myPassword;";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
Console.WriteLine("Connection successful.");
// Execute queries here
}
}
}
Java Application Using JDBC
For Java applications, SQL Native Client can be used with JDBC. Below is a code example for connecting to SQL Server using SQL Native Client:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class Main {
public static void main(String[] args) {
String url = "jdbc:sqlserver://myServerAddress;databaseName=myDataBase;user=myUsername;password=myPassword;";
try (Connection connection = DriverManager.getConnection(url)) {
System.out.println("Connection successful.");
// Execute queries here
} catch (SQLException e) {
e.printStackTrace();
}
}
}
C/C++ Development with ODBC
For C/C++ applications, SQL Native Client can be accessed using ODBC. Below is a simple example demonstrating the connection:
#include <stdio.h>
#include <sql.h>
#include <sqlext.h>
int main() {
SQLHENV hEnv;
SQLHDBC hDbc;
SQLRETURN ret;
SQLAllocHandle(SQL_HANDLE_ENV, SQL_NULL_HANDLE, &hEnv);
SQLSetEnvAttr(hEnv, SQL_ATTR_ODBC_VERSION, (SQLPOINTER)SQL_OV_ODBC3, 0);
SQLAllocHandle(SQL_HANDLE_DBC, hEnv, &hDbc);
ret = SQLDriverConnect(hDbc, NULL,
"DRIVER={SQL Server Native Client};SERVER=myServerAddress;DATABASE=myDataBase;UID=myUsername;PWD=myPassword;",
SQL_NTS, NULL, 0, NULL, SQL_DRIVER_COMPLETE);
if (ret == SQL_SUCCESS || ret == SQL_SUCCESS_WITH_INFO) {
printf("Connection successful.\n");
// Execute queries here
}
SQLDisconnect(hDbc);
SQLFreeHandle(SQL_HANDLE_DBC, hDbc);
SQLFreeHandle(SQL_HANDLE_ENV, hEnv);
return 0;
}
PHP Applications
In PHP, SQL Native Client can be accessed through extensions. A simple connection example is shown below:
$serverName = "myServerAddress";
$connectionOptions = array(
"Database" => "myDataBase",
"Uid" => "myUsername",
"PWD" => "myPassword"
);
// Establishes the connection
$conn = sqlsrv_connect($serverName, $connectionOptions);
if ($conn) {
echo "Connection successful.";
// Execute queries here
} else {
echo "Connection failed.";
}
Integration with Scripting Languages
SQL Native Client can also be integrated with scripting languages like Python and Perl. For Python, you can use the pyodbc
library:
import pyodbc
connection_string = 'DRIVER={SQL Server Native Client};SERVER=myServerAddress;DATABASE=myDataBase;UID=myUsername;PWD=myPassword;'
conn = pyodbc.connect(connection_string)
print("Connection successful.")
# Execute queries here
Best Practices and Performance Tuning
To optimize SQL Native Client performance, consider the following best practices:
Efficient Connection Management
Implement efficient connection management strategies, such as reusing connections through pooling. This practice reduces the overhead of establishing new connections and enhances application responsiveness.
Query Optimization
Optimize your SQL queries to ensure they run efficiently. Use indexing, avoid unnecessary joins, and limit the number of rows returned by queries. This optimization can significantly improve data access times.
Mitigating Network Latency
Network latency can impact SQL Native Client performance. To mitigate this, consider using local databases for testing and optimize network configurations. Implementing caching strategies can also help reduce the frequency of database calls.
Monitoring and Profiling
Regularly monitor and profile SQL Native Client applications to identify performance bottlenecks. Use tools like SQL Server Profiler to analyze query performance and make necessary adjustments.
Security Best Practices
Implement security best practices by using strong passwords, encrypting sensitive data, and keeping your SQL Native Client updated. Regularly review security configurations to prevent unauthorized access.
Scaling Applications
As applications grow, scalability becomes essential. SQL Native Client supports scaling by efficiently managing connections and handling large data volumes. Consider using partitioning and sharding techniques to manage extensive datasets effectively.
Further Learning with Chat2DB
To enhance your experience with SQL Native Client, consider using Chat2DB. Chat2DB is a database management tool that simplifies database interactions and provides a user-friendly interface for managing SQL Server databases. It supports various features, including query building, data visualization, and performance monitoring.
By incorporating Chat2DB into your workflow, you can streamline your development process and leverage the full capabilities of SQL Native Client. Explore further resources and documentation to deepen your understanding of SQL Native Client and improve your database management skills.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!