Working with JSON in SQL Server: Unlocking Data Management Potential
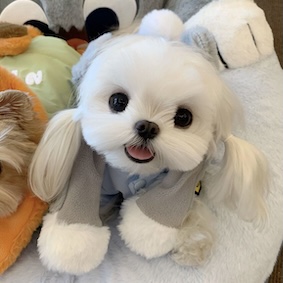
Introduction
SQL Server has integrated JSON (JavaScript Object Notation) capabilities, allowing developers to handle data effectively. JSON is favored for its lightweight and easy-to-use format, making it an ideal choice for data interchange in modern applications. SQL Server's support for JSON simplifies data manipulation, and tools like Chat2DB enhance these workflows, making data management more efficient. Understanding how to leverage SQL Server's JSON functions is crucial for developers aiming to create robust applications.
Understanding JSON in SQL Server
JSON is a text-based format that represents structured data. It is lightweight and easy to read, which is why it is commonly used for data interchange between a server and a web application. SQL Server introduced native JSON support starting with SQL Server 2016, allowing developers to work with JSON data directly within the database.
Advantages of JSON Over XML
While both JSON and XML can be used for data interchange, JSON has several advantages:
- Simplicity: JSON uses a straightforward syntax, making it easier to read and write.
- Less Verbose: JSON typically requires fewer characters than XML, reducing data size and improving performance.
- Native Support: SQL Server provides built-in functions for JSON handling, making operations more efficient.
JSON Data Types in SQL Server
In SQL Server, JSON data is treated as an nvarchar
type. This allows developers to store JSON strings in tables, enabling them to manage structured data without needing a separate schema. The following JSON functions are essential for working with JSON data:
- JSON_VALUE: Extracts a scalar value from a JSON string.
- JSON_QUERY: Retrieves an object or array from a JSON string.
- JSON_MODIFY: Updates a JSON string without rewriting the entire structure.
Parsing and Storing JSON Data
Efficiently parsing and storing JSON data is key to leveraging SQL Server's capabilities.
Using OPENJSON
The OPENJSON
function parses JSON data and returns a relational format. This is particularly useful for converting JSON arrays into tabular formats. Here’s an example of how to use OPENJSON
:
DECLARE @json NVARCHAR(MAX) = '[
{"id": 1, "name": "John"},
{"id": 2, "name": "Jane"}
]';
SELECT *
FROM OPENJSON(@json)
WITH (
id INT '$.id',
name NVARCHAR(200) '$.name'
);
Storing JSON Data
Storing JSON data in SQL Server tables allows for flexible data handling. Developers should follow best practices to ensure optimal performance:
- Use appropriate data types: Store JSON in
nvarchar(max)
columns. - Index JSON data: Create computed columns for frequently accessed JSON properties and index them to improve query performance.
Validating JSON Data
To ensure data integrity, the ISJSON
function is essential. It checks if a string contains valid JSON format. Here’s how to use it:
IF ISJSON(@json) = 1
BEGIN
PRINT 'Valid JSON';
END
ELSE
BEGIN
PRINT 'Invalid JSON';
END
Manipulating JSON Data
Manipulating JSON data is straightforward with SQL Server's built-in functions.
Extracting Values
To extract specific values from JSON, use the JSON_VALUE
function. For instance, if you want to get the name of the first object in the previous example:
SELECT JSON_VALUE(@json, '$[0].name') AS FirstName;
Retrieving Sub-Objects
If you need to retrieve more complex structures, JSON_QUERY
is your go-to function. This can be used to extract entire objects or arrays:
SELECT JSON_QUERY(@json, '$') AS AllData;
Modifying JSON Data
Updating JSON data can be efficiently managed using JSON_MODIFY
. This function allows you to change values without replacing the entire JSON string. For example, if you want to change Jane's name:
SET @json = JSON_MODIFY(@json, '$[1].name', 'Janet');
Concatenating JSON Data
You can concatenate JSON data by combining multiple JSON strings. This can be useful when merging datasets:
DECLARE @json2 NVARCHAR(MAX) = '[
{"id": 3, "name": "Sam"}
]';
SET @json = JSON_MODIFY(@json, 'append $', @json2);
Integrating JSON with SQL Queries
Integrating JSON with SQL queries enhances dynamic data manipulation capabilities.
Using JSON in SQL Statements
You can include JSON data in SELECT
, UPDATE
, and DELETE
statements. For instance, to update a record based on a JSON value:
UPDATE YourTable
SET YourColumn = JSON_VALUE(@json, '$[0].name')
WHERE YourCondition = 'SomeCondition';
Creating Dynamic SQL Queries
For complex data operations, combining JSON functions with traditional SQL queries can be powerful. Here’s an example of creating dynamic SQL:
DECLARE @sql NVARCHAR(MAX);
SET @sql = 'SELECT * FROM YourTable WHERE YourColumn = ' + JSON_VALUE(@json, '$[0].name');
EXEC sp_executesql @sql;
Performance Considerations
When working with JSON in SQL Server, be mindful of performance. Complex JSON queries can slow down operations. It is crucial to monitor and optimize queries involving JSON data to ensure efficiency.
Best Practices and Performance Optimization
Optimizing JSON handling in SQL Server is essential for maintaining performance.
Indexing Strategies
Implement indexing strategies by creating computed columns for frequently queried JSON properties. This can significantly enhance query performance.
Managing JSON Data Size
The size of JSON data can affect performance. It is advisable to keep JSON data compact and avoid unnecessary nesting.
Trade-offs Between JSON and Relational Data
Consider the trade-offs between using JSON and traditional relational data models. JSON offers flexibility, but in certain cases, a relational approach can provide better performance and integrity.
Monitoring and Troubleshooting
Regularly monitor JSON performance issues and troubleshoot queries. Use SQL Server’s built-in tools to analyze query performance and optimize where necessary.
Use Cases and Real-world Applications
SQL Server's JSON features have real-world applications across various domains.
Web Applications
JSON is widely used in web applications for data interchange between clients and servers. It simplifies the process of sending and receiving structured data.
Mobile Applications
In mobile applications, JSON facilitates data synchronization by providing a lightweight format that reduces bandwidth usage.
IoT Data Management
JSON plays a vital role in IoT systems, where devices generate large amounts of data. SQL Server's JSON support enables efficient storage and retrieval of this data.
Cloud-based Applications
JSON is integral to cloud services and applications, allowing for seamless integration and data sharing across platforms.
Business Intelligence and Analytics
JSON enhances business intelligence by enabling data integration from diverse sources. SQL Server’s JSON capabilities allow organizations to analyze data more effectively.
Further Learning with Chat2DB
To enhance your data handling and manipulation tasks, consider using Chat2DB. This tool provides a user-friendly interface for managing SQL Server databases and working with JSON data efficiently. By utilizing Chat2DB, developers can unlock new possibilities in their data workflows and improve application development processes.
For more insights and practical applications of SQL Server’s JSON capabilities, continue exploring resources and tutorials available online. Embrace the power of JSON in SQL Server to elevate your development skills and create innovative solutions.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!