What Are Database Triggers: A Beginner's Guide
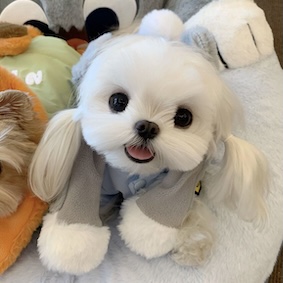
Learn about database triggers, their types, and how they automate tasks and maintain data integrity. Learn real-world applications and best practices.
Database triggers are automated mechanisms designed to run automatically when specific activities occur on a database table or view. The key role of triggers is their ability to respond instantly to changes in the database, ensuring data consistency and integrity. They can automatically perform a series of predefined actions such as data validation, recording change history, or implementing business logic rules when data is inserted, updated, or deleted. This feature of triggers is essential to maintaining database accuracy and reliability because they can take immediate action during data modification, thereby preventing non-compliant data from entering the database system.
Understanding Database Triggers
Definition and Basic Concepts
What are database triggers?
Database triggers are program segments stored in the database system that are automatically called and executed by the system when certain database events occur, such as INSERT, UPDATE, or DELETE operations on tables or views. Triggers are designed to perform certain actions when data is modified, thereby ensuring data integrity and consistency. By implementing predetermined logic immediately when data changes, triggers help maintain the overall reliability and structural integrity of the database.
Key Components of Triggers
Database triggers are composed of several key components:
- Trigger name: a unique identifier for the trigger.
- Trigger event: an event that activates the trigger, such as INSERT, UPDATE, or DELETE.
- Trigger condition: a condition that must be met for the trigger to execute.
- Trigger action: the program code that is executed when the trigger fires.
For example: We will create a simple trigger that automatically updates records in other table logs when a new record is inserted into the employees table.
Types of Database Triggers
Before Triggers:
Before triggers are executed before data is inserted, updated, or deleted. This type of trigger is mainly used to perform data validation or execute business logic before data changes to ensure that the upcoming operation meets pre-set conditions. For example, a before trigger can check whether the records meet specific standards or rules before inserting new records.
After Triggers:
After triggers are executed after data is successfully inserted, updated, or deleted. This type of trigger is usually used to record the changes made or update related tables. For example, after triggers can be used to create an audit trail that records the details of each modification to a table, which is very useful for future auditing or analysis.
Instead Of Triggers:
Instead Of Triggers are executed instead of the default behavior when performing INSERT, UPDATE, or DELETE operations on a view. They are mainly used to handle complex operations that cannot be directly completed through standard SQL statements. For example, when a user attempts to modify data through a view, an instead of trigger can ensure that multiple underlying tables are properly updated instead of simply applying the operation to the view itself.
How Database Triggers Work
Triggering Events
Triggering events are specific actions that activate a trigger. Common triggering events include INSERT, UPDATE, and DELETE. Each event type can have multiple triggers associated with it, allowing for complex data interactions.
Triggering Conditions
Triggering conditions specify when a trigger executes. These conditions can be simple or complex, depending on the requirements. For example, a triggering condition might check if a specific column value exceeds a threshold before executing the triggering action.
Triggering Actions
Trigger actions are program code that is executed when a trigger fires. These actions can range from simple data validation to complex business logic. For example, a trigger action might update related records in other tables or send a notification to a user.
Practical Applications of Database Triggers
Automated Data Validation
Database triggers can automatically perform data validation to ensure that only compliant data is entered into the system. For example, a trigger can check whether an email address meets the expected format specifications before allowing an insert operation. This not only reduces the amount of data that needs to be manually checked, but also enhances the consistency and reliability of the data. By automating these validation steps, triggers help improve data quality and simplify the data management process.
Example Use Case
Consider an e-commerce platform where users register using their email addresses. A BEFORE INSERT trigger can validate the email format. The trigger ensures that each new entry meets the required conditions, thereby preventing invalid data from entering the database. This validation process helps maintain the integrity of user information.
CREATE TRIGGER validate_email_before_insert
BEFORE INSERT ON users
FOR EACH ROW
BEGIN
-- Verify email address format
IF NOT (NEW.email REGEXP '^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,4}$') THEN
SIGNAL SQLSTATE '45000' SET MESSAGE_TEXT = 'Invalid email format';
END IF;
END;
Enforcing Business Rules
Database triggers ensure that business rules are enforced by automatically executing preset actions when certain events occur. These triggers help to align database operations with corporate policies and procedures. For example, a trigger can enforce certain rules to ensure that all data processing follows established standards and requirements.
Example Use Case
In a payroll system, a BEFORE UPDATE trigger can check whether a proposed salary increase exceeds 10%. If the increase exceeds this limit, the trigger can block the update. This enforcement mechanism ensures compliance with company policies and maintains fairness and consistency in salary adjustments.
CREATE TRIGGER check_salary_increase
BEFORE UPDATE ON employees
FOR EACH ROW
BEGIN
DECLARE salary_increase_percentage DECIMAL(5, 2);
-- Calculate salary increase percentage
SET salary_increase_percentage = ((NEW.salary - OLD.salary) / OLD.salary) * 100;
-- Check if salary increase is more than 10%
IF salary_increase_percentage > 10 THEN
SIGNAL SQLSTATE '45000'
SET MESSAGE_TEXT = 'Salary increase exceeds the allowed limit of 10%.';
END IF;
END;
Auditing and Logging
Database triggers support auditing and logging by automatically recording changes to data. The audit trail generated by these triggers contains important information such as who made the change, what was changed, and when the change occurred. This detailed record is important for tracking data changes and ensuring accountability for actions.
Example Use Case
Financial institutions can use AFTER UPDATE triggers to record changes to customer account balances. Each time the account balance is updated, the trigger records the old and new values, along with a timestamp and user ID. This audit trail provides a comprehensive record of all transactions, which helps with compliance and fraud detection.
CREATE TRIGGER log_account_balance_changes
AFTER UPDATE ON accounts
FOR EACH ROW
BEGIN
-- Only record when the balance changes
IF NEW.balance != OLD.balance THEN
INSERT INTO account_audit (account_id, old_balance, new_balance, updated_by)
VALUES (OLD.id, OLD.balance, NEW.balance, NEW.last_updated_by);
END IF;
END
Advantages and Disadvantages of Using Database Triggers
Benefits
1. Automation
Database triggers can automate repetitive tasks, reducing the need for manual intervention. For example, when data changes, triggers can automatically synchronize these changes to related tables. This process not only improves work efficiency, but also ensures the consistency of the entire database environment.
2. Data Integrity
Triggers play a key role in ensuring data integrity. They can automatically implement various rules and constraints. For example, triggers can ensure the integrity of foreign key relationships and prevent inconsistent data from entering the database. This automated execution helps avoid data anomalies and maintain the stability and reliability of the database.
Disadvantages
1. Complexity
Database triggers may introduce additional complexity. The logic inside the trigger may become difficult to maintain and manage. Developers may find it quite tricky to find and fix problems in triggers. This complexity increases the difficulty of maintenance.
2. Performance Impact
Triggers may have an impact on database performance. Every time a trigger is activated and executed, additional overhead is added to database operations. Especially for large-scale databases, this overhead may accumulate into a significant performance bottleneck. Under high load conditions, especially when processing a large number of transactions, performance degradation may be observed. Therefore, when implementing triggers, you need to weigh their functional requirements against their potential performance impact.
Best Practices for Implementing Database Triggers
Writing Efficient Triggers
Avoid Recursive Triggers
A recursive trigger is a situation where the action of a trigger indirectly or directly causes the same trigger to be fired again. This can lead to an infinite loop and negatively impact database performance. To avoid this, developers should carefully design the behavior of triggers to ensure that the actions of the trigger do not modify the table or view that originally activated the trigger. It is also a good idea to use conditional logic to prevent unnecessary trigger execution.
Minimize Performance Overhead
The use of triggers imposes additional processing overhead that can affect database performance. To minimize this overhead, developers should write efficient and concise trigger code. Optimize the SQL statements within the trigger to shorten execution time, limit the amount of data processed by the trigger, use indexes to speed up data retrieval, and avoid performing complex calculations or operations inside the trigger. Following these best practices can help keep database operations efficient.
Testing and Debugging Triggers
Common Testing Strategies
The key to ensuring that database triggers work correctly is to test them thoroughly. A common testing approach is to create a series of test cases that simulate the triggering events. For example, you can test insert, update, and delete operations to verify that the trigger behaves as expected. Use a diverse set of test data, including various scenarios including edge cases. Monitor database activity to confirm that the triggers are working as intended. Log test results to track trigger performance and identify problems.
Tools and Techniques
There are many tools and techniques for testing and debugging database triggers. Take advantage of database management systems (DBMS) with built-in debugging capabilities. These tools allow developers to execute trigger code line by line and examine the state of intermediate variables. Log the execution of triggers to collect detailed information about trigger activity. Use this log data to diagnose and resolve problems. Use a version control system to track changes to trigger code, which helps record modification history and roll back to a previous version when necessary.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!